Priority Queue in C#: Tips + Tricks
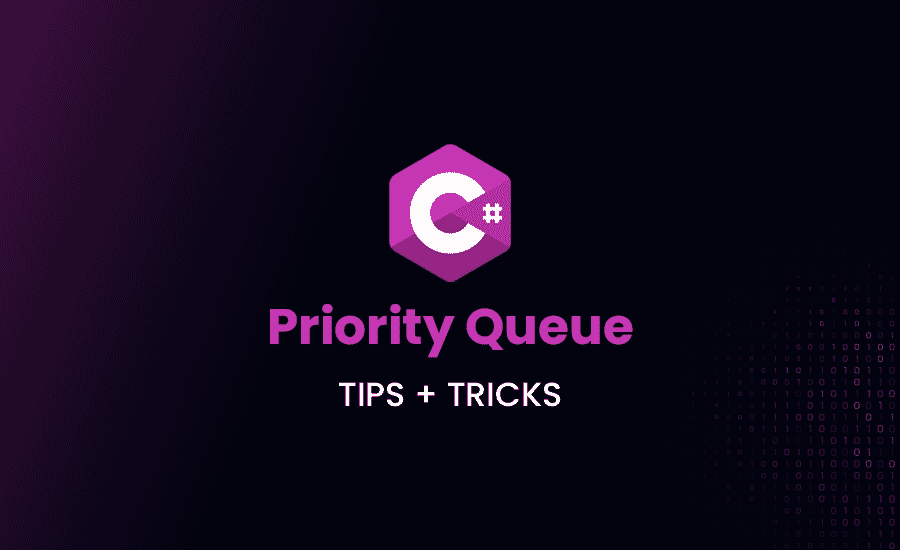
Imagine the world of programming without efficient data structures. Frightening, right? Thank heavens we have them! Today, we’ll dive into one of the most valuable data structures around – the Priority Queue. And the programming language in focus? C#! Get ready for a thrilling ride!
Introduction to Priority Queue C#
Before we dive into the core of our C# journey, let’s understand what a Priority Queue is.
What is a Priority Queue?
A Priority Queue is a sophisticated abstract data type resembling a queue or stack, with each element assigned a ‘priority.’ And just like in a real-life queue, higher priority elements show up front. Quite straightforward, right?
How Does a Priority Queue Work?
Now that we’re in the queue, how does it work? In simple terms, elements with high priority are dequeued before those with low priority. If elements have the same priority, they get served based on their order in the queue. This behaviour distinguishes Priority Queue from regular queues. Let’s explore more!
Creating a Priority Queue in C#
We’ve got the basics down, so let’s move on to the practical part. Brace yourself!
How To Declare Priority Queue C#
Here’s the burning question: how do we declare a priority queue in C#? Well, it’s a bit tricky because C# doesn’t have a built-in Priority Queue class. But fret not. We’ve got a strategy – we use the SortedDictionary class instead. Let’s see how!
Loading code snippet...
In this piece of code, we’ve effectively declared a Priority Queue using SortedDictionary. The int
keyword represents the keys (our priorities), and string
stands for the values (or data elements).
How To Create a Priority Queue in C#
Having declared our queue, how do we now concoct our Priority Queue?
Initial Setup for Priority Queue in C#
Here’s a simple class to get things off the ground:
Loading code snippet...
In this snippet, we’ve initialized a dynamic data structure, a list, that will behave as our Priority Queue.
Implementing the Queue
But our queue isn’t complete without some essential functions, right? Let’s add those!
Loading code snippet...
In this bit, we’ve built methods for inserting elements (Push
), removing elements (Pop
), and checking if the queue is empty (IsEmpty
). We’re one step closer to our Priority Queue!
- Wait, won’t these operations be costly?
- Sure, they would be in an extensive dataset. That’s where custom binary heap implementations come into play. But hey, isn’t this a good starting point?
C# Priority Queue in Applications
Priority Queues are like the secret sauce that adds flavor to an assortment of real-world applications. From scheduling tasks to facilitating efficient resource allocation, these data structures work behind the scenes to enhance our computing experiences every day.
Standard Applications of Priority Queue in C#
Priority Queues are used extensively across a wide range of domains and functionalities.
- Algorithmic Scheduling: To manage multiple tasks with varying priorities, a priority queue is used to sequence them. This scheduling is seen in CPU Scheduling, Disk Scheduling, and more.
- Graph Algorithms: Algorithms like Dijkstra’s Algorithm, Prim’s Algorithm, etc., make use of priority queues to extract the minimum in the shortest possible time.
- Traffic System Simulation: In real-life traffic junction simulations, the vehicle with the highest priority (for instance, ambulances or fire engines) is cleared first.
To give a better feel, let’s delve into a C# Priority Queue Example.
C# Priority Queue Example
In order to understand priority queues more intimately, let’s take a look at an example. We’ll create a Priority Queue and add a few elements to it. Then we’ll examine how it behaves when we try to retrieve those elements.
Loading code snippet...
In the above block of code, we’re adding elements (namely 3, 2 and 4) to our Priority Queue named priorityQueue
. Post this, when we invoke the Pop()
method, we retrieve ‘2’. This is because ‘2’ has the highest priority according to our Priority Queue implementation (the element with the smallest value takes precedence).
Concurrent Priority Queue C#
Distributed computing and multi-threading environments pose new challenges to existing data structures. If multiple threads try working with our Priority Queue simultaneously, the outcomes may get unpredictable. So, to ensure consistency and performance, Concurrent Priority Queue comes into the picture.
For instance, consider a multi-threaded server application scheduling tasks based on their priority. A multi-threaded operating system schedules tasks and maintains them in a job queue. A concurrent priority queue is perfect for exactly this kind of scenario.
C# Priority Queue: Under The Hood
A Priority Queue’s efficiency and efficacy primarily depend on its implementation details. To understand this, we need to delve into the mechanics of a Priority Queue.
Examining the Internal Mechanism
To handle priorities, Priority Queues implement the IComparable<T>
interface and leverage the CompareTo
method. This allows each element to be compared against others, enabling internal sorting.
Loading code snippet...
In the above code, when the Push
method is called, the element is added to the list. The list elements are then sorted by the Sort
method using lambda expressions. Here (a,b) => a.CompareTo(b)
serves as our comparison logic. This expression will compare a
and b
and sort them accordingly.
How Priority Queue Sorts its Elements
The Priority Queue sorts its elements based on the CompareTo
function of the IComparable<T>
interface. The CompareTo
function establishes a sort ordering. When comparing ‘a’ and ‘b’, if ‘a’ is less than ‘b’, it returns a negative number. A zero indicates equality, and a positive number implies ‘a’ is greater than ‘b’.
So, whether you are sorting integers, floating-point numbers, or custom objects like the Employee
class in your application, the Priority Queue can handle them all. All you need to do is provide a class or structure that implements the IComparable<T>
interface and specifies how to compare the object with others. This way, the Priority Queue will know how to sort them based on priority.
Conclusion
Before we part ways, here’s a quick recap of what we’ve learnt today:
- Priority Queues and how they work
- Declaring and implementing a Priority Queue in C#
- Practical applications of a Priority Queue, including concurrency
- The internal workings of a Priority Queue in C#
So, done waiting? Hop into your favourite text editor, open a new file (or that project you’ve been delaying), and play around with Priority Queues. Remember, practice is key! What will happen if you dispel Priority Queues? Well, in a world that equally loves efficiency and C#, I’m sure you can guess.
Now, go explore, create, innovate. The world of Priority Queues in C# is waiting for you!