JsonSerialization in C#: Step-by-Step Guide
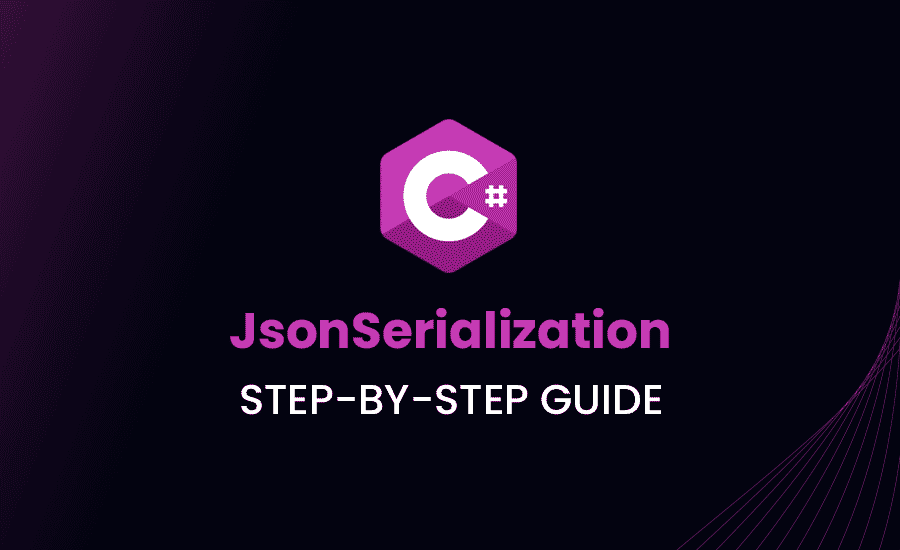
Hello there, dear reader. Are you interested in mastering JsonSerialization in C#? Well, you’ve come to precisely the right place. Let’s embark on an exciting journey together, exploring everything you need to know about JsonSerialization in C#.
Understanding JsonSerialization in C#
Ever wandered what is behind the data transformation process? How does a bunch of class files talk to other services possibly written in a different language? The answer lies in JsonSerialization.
What is JsonSerialization in C#
JsonSerialization, basically, is the process of converting an object into a JSON format string. Now, you might wonder why exactly we would need this. Let’s find out.
Why JsonSerialization is important in C#
In simple terms, JSON is a format that’s used to store information in an organized, and easy-to-access manner. It provides us a human-readable collection of data that we can access in a really logical manner, and it is predominantly used to transfer data across the web.
Dive into Json serialize with C#
Before we become JSON Serialization superstars, we’ll first cover some basics. Are you ready? Hang tight!
Basic usage of Jsonserializer in C#
How do you cook this JSON soup? Fear not, for C# provides an easy way to do that through the JsonSerializer.
Loading code snippet...
In the above snippet, we’re creating an object of Product and setting some properties. The line JsonSerializer.Serialize(product)
converts this product object into a JSON string. Easy right?
Exploring C# jsonserializer.deserialize
Now, imagine you received some JSON data and you need to convert it back into a C# object. That’s where deserialization comes into action.
Dive into Json serialize with C#
Let’s buckle up and dive into the bedrock of Json Serialization – embracing the journey of transforming C# objects into JSON and vice versa. Along the way, we’ll parse through examples and real-world scenarios, equipping you with the tools to confidently wield C# JsonSerializer.
Basic usage of Jsonserializer in C#
Ever wondered how to transmit your awesome C# objects to other systems, perhaps to a web service or a database? Say hello to JsonSerializer – an impressively handcrafted tool for this task. It helps you change or “serialize” your C# objects into JSON strings. Let’s look at an example:
Loading code snippet...
In this code, we create a Student
object and then serialize it using JsonSerializer.Serialize(student)
. This creates a string representation of our student object in JSON format. Now, you can conveniently send this JSON string to a web service, save it in a database or do whatever you please!
What if you have a list of student objects? No worries, JsonSerializer got you covered:
Loading code snippet...
Here we have a list of Student
objects and JsonSerializer conveniently serializes the whole list into a JSON array. Neat, isn’t it?
Exploring C# JsonSerializer.Deserialize
After unraveling the mystery of serialization, let’s flip the table and move to its alter ego – Deserialization. It’s the process of converting JSON data back to its original form, our beloved C# objects.
Imagine you’ve received a JSON string from a web service and you need to use this data. JsonSerializer.Deserialize helps you transform this JSON string back to a C# object. Let’s see how it works:
Loading code snippet...
In this snippet, we’re telling JsonSerializer to convert jsonStudent
to a Student
object using JsonSerializer.Deserialize<Student>(jsonStudent)
. Voila, our JSON string is back to being a Student
object.
What about a JSON array? Yup, JsonSerializer got that covered too:
Loading code snippet...
This code transforms the JSON array jsonStudents
back to a list of Student
objects. That’s how versatile JsonSerializer is, it never misses a beat!
Advanced JsonSerialization Options in C#
JSON Serialization in C# is not merely a tool, it’s a powerhouse. Its versatility helps developers cater to a wide range of requirements. Let’s delve deeper and explore some of the advanced JsonSerialization options available in C#.
How to Ignore Class Property from JsonSerialization in C#
Often while serializing an object to a JSON string, there are certain properties that you might not want included in your resultant JSON string. Well, JsonSerializer provides an easy way to achieve this.
Consider an example. Let’s say you are working on a user management system. Here is a simplified User class in C#:
Loading code snippet...
Now, you need to send the user’s information to the client but you don’t want to include the password. Here, JsonIgnore
attribute comes to the rescue:
Loading code snippet...
With the JsonIgnore
attribute added to the Password property, JsonSerializer will exclude it from the output JSON string, thus maintaining the security of user data.
Now, let’s look at other advanced JsonSerialization options in C#. In order to do so, we will need to utilize JsonSerializerOptions
. These options give us a greater control over the serialization process. We can alter things such as naming policy, date format and how null values are handled.
Loading code snippet...
In the JSON string that results from this code, property names will be in camelCase, it will be indented for easier reading, and any null values will be ignored.
But that’s just the tip of the iceberg. JsonSerializer provides several more options. For example, you can control how to handle properties with default values:
Loading code snippet...
With these options, JsonSerializer will include readonly properties in the output, but ignore properties that have default values.
Now, there’s a whole wealth of advanced serialization topics in C# we haven’t covered yet. But don’t sweat it, we’ll tackle them in our upcoming posts. Remember, it’s not the destination, but the journey that counts. Get your hands dirty with what you’ve learned so far, play around and experiment, the pieces will start to connect. Get all inquisitive about it.