Finalize Objects C# – Full Guide
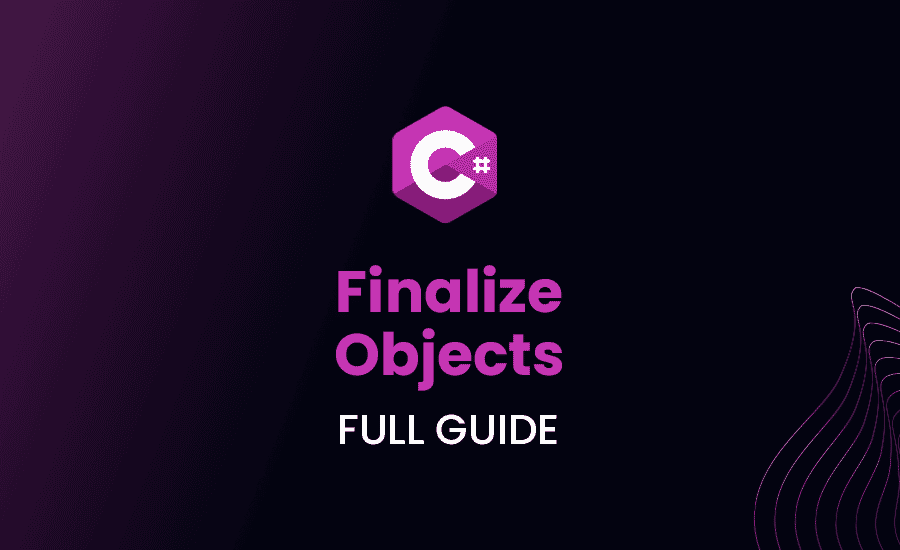
Fellow developers, let’s take a voyage through this exciting world of finalizing objects in C#! You know how sometimes you forget to clean up after a party, and the room remains messy? Just think of objects in the same way. That’s where finalize c# comes in – it’s kind of like your friendly cleaning crew, making sure your memory space is tidy! Without further ado, let’s dive into it!
Understanding Finalize C#
Settle in with your (possibly fourth) cup of coffee as we dig deeper into finalization in C#. We’re about to immerse ourselves in the ocean of finalize in C#. Stay with me on this, it will be an interesting ride!
Finalization is the process where objects that are no longer needed are reclaimed. In C#, finalization is automagically performed before the Garbage Collector (GC) reclaims the object. Yes, you heard right, it’s our lovely Garbage Collector who sets the finalization wheels in motion.
Loading code snippet...
In this code snippet, the BaseClass
has a finalizer, denoted by the ~
symbol. When the GC decides to clean up and reclaim the BaseClass
, it will first call the finalizer, allowing the BaseClass
to do its goodbyes and clean up after itself.
However, the real fun of C# lays in its “managed” environment. This means it handles most of the memory management for your applications. So, where do finalizers come in, you ask? You know, I see finalizing as your trusty Swiss army knife. It’s the tool C# gives us to clean up unmanaged resources. The databases, streams, or any other stuff, you know, those ancients of the code world that C# just can’t trash.
The Role of Finalizers in C#
Finalizers are the neat-freaks of your code, always ensuring a clean exit. They’re the orderly clerks at the supermarket, checking off every item before it goes out. But don’t get carried away; not everything needs checking off.
Now the real question, is why should we bother with finalizing when the GC is already doing all the heavy lifting? Let’s imagine you’re organizing the biggest music festival of the year. Sure, there is a clean-up crew (the GC), but there are also those unmanaged resources – like rented sound equipment and canopies. You can’t just leave everything for the clean-up crew. You have to return some things in order, right? That’s exactly what the finalizer does.
Let’s break this down:
Loading code snippet...
The UnManagedWrapper
class in this example uses unmanaged memory. In the constructor, we allocate some unmanaged memory. In the Dispose
method and the finalizer, we free that unmanaged memory. The GC.SuppressFinalize(this)
in the Dispose
method tells the GC that it doesn’t need to bother – we’ve already cleaned up after ourselves.
The Finalize Method C#
Pat yourself on the back because you’ve braved the churning seas of finalization in C#. Having anchored ourselves here, we’re going directly to the heart of the matter – the Finalize
method. This is, in essence, C#’s way of ensuring garbage collection operates seamlessly.
Let’s consider an example. We create a class YourResourceClass
, which will simulate the use of some resources we should take care of, like file handlers, sockets or database connections. When this class is no longer necessary, we need to finalize it, that is to say, free up the resources it was using.
Loading code snippet...
In the example above, our finalizer – the ~YourResourceClass()
method, checks if the class is using a resource and if so, it frees it (isResourceInUse = false
) then prints a confirmation message “Finalized!” to the console.
Finalize Method Syntax
Understanding how the Finalize
method works is important, but how about writing it? Luckily, the syntax for the Finalize
method is standard in C# and not hard to grasp:
Loading code snippet...
In the above example, the Finalize
method is defined by the ~YourResourceClass()
. Notice it’s same as the class name but with a tilde ~
in front. This is the syntax C# uses to denote a finalizer! Have at it and code away.
Best Practices For Finalize Method C#
Finalize
methods can often lead to complications if not used correctly, which is where best practices come into play. Not only are these crucial to ensuring your code runs smoothly, but they’re also good habits to develop as a C# developer.
Here are a few important ones to remember:
- Only use when necessary. The Garbage Collector in .NET is very efficient and does a great job clearing up managed resources. So, if you don’t have any unmanaged resources to clean up, don’t use finalizers. It should act as a safety net for unmanaged resources and not for freeing managed ones.
Loading code snippet...
- Supress finalization if you’ve already done the cleanup. If you’ve cleaned up the resources (maybe because you used IDisposable and Dispose pattern), inform the GC so it doesn’t have to bother calling the
Finalize
method. Here’s how you can do it:
Loading code snippet...
- Use to free unmanaged resources. Unmanaged resources are the ones that aren’t automatically cleaned by the garbage collector, things like file handles, sockets, or database connections:
Loading code snippet...
In the example above, I demonstrate handling an unmanaged resource using interop with the CloseHandle
method coming from the Windows Kernel32 library. We also suppress the execution of the finalizer if Dispose()
is called, a key optimization that can boost performance.
Finalizers Vs. Dispose in C#
Finalizers and Dispose method, facing off in the C# stadium! Ever found yourself puzzled, scratching your head and wondering while coding – should I be using Finalize or Dispose? Well, worry not! Understanding their difference and choosing the right method for your application is like choosing the right tool for the job. It could make a massive difference in how your app performs, making it the smoothest, most efficient code deploying machine!
Finalizers and Dispose might seem like two peas in a pod, but they’re more like chalk and cheese. Finalizers are like your silent, behind-the-scenes ninjas. They operate by implicitly cleaning up unmanaged resources. You don’t see them in action, but they’re there, doing their job!
Loading code snippet...
See the ‘~’ sign before the class name MyClass
? That’s where the Finalizer does its magic.
Now, let’s talk about the Dispose method, the other knight in our C# coding kingdom. Unlike the Finalizer, Dispose doesn’t keep its work undercover. It’s explicit and handles both managed and unmanaged resources in a timely fashion.
Loading code snippet...
Here, MyClass is implementing IDisposable and explicitly calling the Dispose method, saying ‘Here I am, doing my resource cleaning magic!’
Performance Implications of Finalize C#
Let’s get back to our magic show for the final act! Let’s shine the spotlight on performance. If you’re a speed enthusiast, constantly pushing your code’s boundaries to run faster, you must be wondering – could the Finalize
method be slowing things down a tad bit? Instead of a rapid-firing code machine, could it be something more like the tortoise from our good old tortoise and the hare story?
Well, the answer is… it could be! But here’s the twist – you’ve got the Garbage Collector (GC) playing the Phoenix from Dumbledore’s office, rising from the ashes, reducing the overhead of finalization.
Loading code snippet...
By using GC.SuppressFinalize(this);
, you tell the Garbage Collector, ‘Hey GC, I got this! Skip the finalization for this object, will ya?’
Whoa! You did it, coding wizard! You’ve just witnessed the epic match of Finalizers vs Dispose, discovered their unique quirks and traits, and mastered their impact on performance. What’s left now? Unleashing this newfound knowledge onto your code, of course!