How to Convert C# Enums to Lists
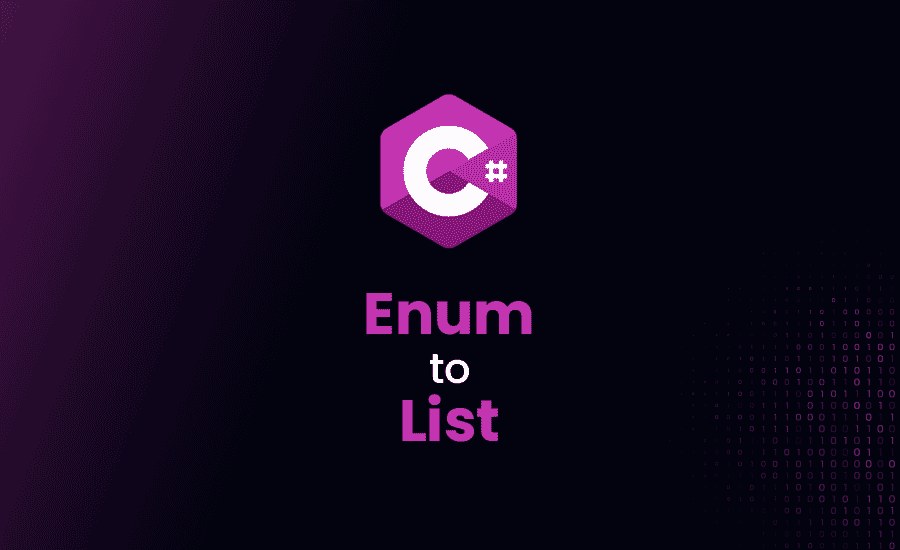
Writing code in a clean and efficient manner is every developer’s ultimate aim. Converting enums to a list can save you valuable time and make your code more elegant. In this guide, we are going to uncover in detail, the procedures and practices of converting C# enums to lists.
Why Convert C# Enum to List
Enum – it’s one little component in the vast and complex world of C#, but it’s got massive potential. Converting enums to lists might seem arbitrary to some, but there are real-world benefits and scenarios where it comes in handy. Buckle up as we dive into this fascinating exploration!
Benefits of Converting Enums to Lists
Converting enums to lists in C# opens up a wealth of possibilities:
- Efficiently iterates through all enum values.
- Enhances readability by giving a clearer picture of what’s happening in your code.
- Simplifies data binding in UI frameworks.
Who knew four lines of code could simplify your programming life so much?
Real-world Scenarios for Converting Enum to List
Real-world instances where converting enum to list would be beneficial are numerous:
- Filling combo boxes or drop-down lists with enum values in a user interface.
- Performing operations on all enum values in a maintainable way.
- Filtering lists based on enum values.
It’s like using a Swiss Army knife instead of a simple pocket knife.
Basic Techniques of Converting Enum to List in C#
When it comes to converting enums to lists, there are a few basic techniques that you can use. It’s kind of like choosing between a screwdriver, a hammer, and a wrench. Each tool has its purpose and utility.
Using
In C#, the built-in Enum.GetValues()
method returns an array of the values of the constants in a specified enum. It’s simple and straightforward.
Loading code snippet...
The code above is all it takes to convert an enum to a list. Easy, right? Proceeding to the next level.
Converting Enum into List with LINQ
LINQ (Language Integrated Query) is an efficient querying tool in C#. To convert an enum into a list, you can use a simple LINQ query.
Loading code snippet...
In the above code snippet, we are using the Range
function from the Enumerable
class to generate a list. All from one succinct line of code!
Performing Cast and Conversion
This method involves first casting the enum to an IEnumerable
then converting to a list.
Loading code snippet...
With the invention of casting and conversion, your coding life just got easier. It’s like switching from manual washing to a washing machine.
Common Pitfalls and Solutions While Converting Enum to List
Every development journey comes with a set of challenges, and converting enums to lists in C# is no exception. Some problems are akin to pesky mosquitoes that buzz around, ready to sting! However, like most things in programming and life, these obstacles create the lessons necessary for us to become better developers.
Dealing with Conversion Exceptions
The process of converting enums to lists can sometimes result in conversion exceptions. A typical example is the InvalidCastException
. This treacherous fellow raises its head when we try to convert an enum to a list of an incompatible type. It’s like trying to fit a square peg in a round hole.
Loading code snippet...
In the code above, we are trying to convert the enum to a list of integers instead of its actual type, MyEnum
. This will result in an InvalidCastException
, caught by our trusty try-catch
block.
The mere mention of exceptions might send shivers down your spine. But like superheroes ready to combat trouble, have no fear; try-catch
blocks are here! Deflecting exceptions is their specialty.
Experiencing NullReference Exceptions
NullReferenceException is another common exception that strikes when you least expect it. It occurs when you’re trying to access members (properties, methods, or indexes) on a type that’s null
.
Loading code snippet...
We’re attempting to access the Count
property of a list that’s null
. This would result in a NullReferenceException
. It’s like expecting water from a tap when the water supply is disconnected. Just another case for our try-catch
block to solve!
Addressing Performance Issues
While the process of converting an enum to list is nifty, it might cause performance issues when done repetitively. Converting the same enum to a list, again and again, can consume more CPU and memory, slowing down the application performance. It’s like lighting a fire with flintstones every time you need it instead of using a match or lighter.
Loading code snippet...
In the snippet above, we cleverly sidestep the performance problem by creating a static and read-only list from the enum. This operation takes place only once and the result is reused in subsequent operations. It’s storing up potential energy that can be deployed as kinetic energy when needed!
Casting Enum to Wrong Numeric Type
Enums in C# are essentially a type of integer. However, they cannot implicitly convert to other numeric types, and will throw an InvalidCastException
.
Loading code snippet...
In this example, casting to a decimal
instead of an int
will result in an InvalidCastException
. It’s like trying to pour a gallon of water into a quart pot. Remember, having an ironclad try-catch
block in place is like kitting out with protective gear – you’ll brave the exceptions with confidence!
Key Takeaways from Converting C# Enum to List
Closing in on our conversation on enum to list conversion, let’s take a moment to reflect upon the key takeaways. Backed by some extensive examples and real-life instances, we aim to solidify your understanding. Just as a space capsule re-enters the Earth’s atmosphere, we’re going in for the final descent – let’s dive!
C# Provides Different Mechanisms to Convert Enums into Lists
C# is a language that gives you multiple ways to achieve the same result. When it comes to converting enums into lists, we have several practical options at our disposal.
Enum.GetValues()
The static method Enum.GetValues()
gets an array of the values of the constants in a specified enumeration. We can cast this array to our enum type and then convert it into a list.
Loading code snippet...
LINQ
Language Integrated Query (LINQ) adds querying capabilities to .NET languages. It offers a more elegant and readable syntax for filtering, projecting, and sorting data in C#.
Loading code snippet...
Understanding the Principles of These Conversions Is Crucial
Just like understanding the rules of the road is essential for navigating traffic, understanding the principles behind these conversions is crucial for mastering C# enum list conversions. Code without understanding is like a ship without a rudder!
Casting
The Cast<>
function in C# is a powerful feature that allows us to perform conversions between incompatible types. It’s like a magic wand that transforms pumpkins into carriages!
Loading code snippet...
Utilizing LINQ efficiently
A LINQ query is fully capable to be as powerful as a SQL query if used correctly. With this ability, you don’t just get the power to convert enums to lists, but also to manipulate and query those lists.
Loading code snippet...
Handle Exceptions, and Always Be Conscious of Performance Issues
When converting enums to lists, always anticipate potential conversion exceptions. Being a step ahead of potential hiccups is like knowing the weather forecast – you’ll always be prepared!
Handling Exceptions
Using try-catch blocks can handle exceptions that may occur from invalid casting during conversion.
Loading code snippet...
Avoiding Performance Issues
When it comes to performance, simplifying repetitive tasks is key. It’s like taking the highway instead of local roads for a cross-country trip. You save time by avoiding unnecessary traffic!
If the enum values are static and don’t change, you can convert the enum to list once and cache it.
Loading code snippet...
With these key takeaways by your side, it’s now your turn to take the wheel and navigate the road of converting enums to lists! Remember, a coder is the artist, and lines of code are the paint that creates an artwork – make every line count!
Final Thoughts
The journey from no knowledge of enum-to-list conversion to a good understanding of it is like the journey from caterpillar to butterfly – completely transforming. As we wave goodbye, always remember – when you get your basics right, you can build a castle. Even if it’s one line of code at a time!
The act of converting enums to a list in C# doesn’t have to be daunting. As with everything else, practice makes perfect. The sooner you adapt these strategies to your own projects, the sooner you’ll find your workload lightening and your efficiency increasing. So why not dive in now? Happy programming!