Conversion of a C# Enum to Int: Guide for Developers
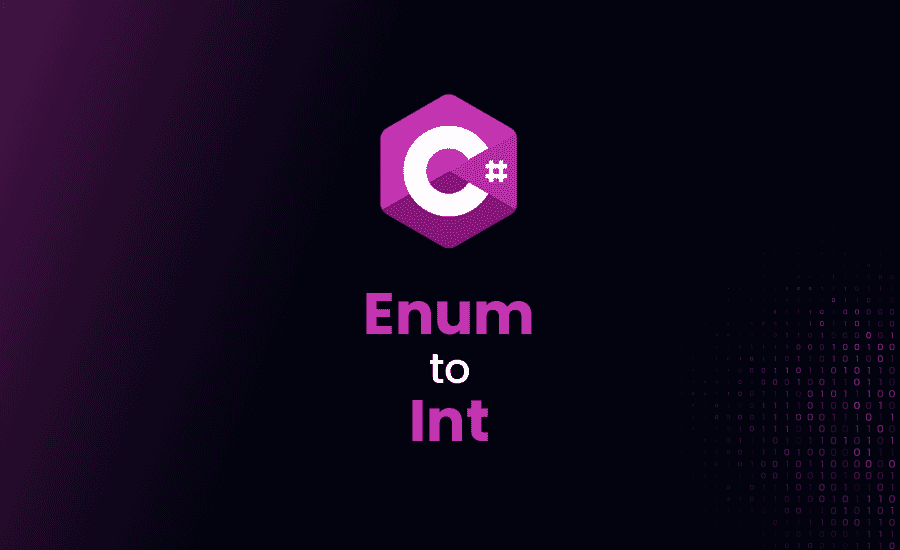
Hey fellow developers, have you been scratching your head trying to figure out how to convert a C# enum to int? Ready to dive into the world of enums and unearth the magic beneath straight conversions? Then buckle up and let me guide you through this adventure.
Convert C# Enums to Integers
But before we jump into the thick of things, let’s dust off some basics. Alright? Conversion processes in programming can sometimes leave us at our wits’ end. However, fear not as we’re going to gracefully tackle everything today, from nifty tricks to dodging common potholes.
Basics of C# Conversions
Before bringing out the big guns, let’s take a short detour to refresh on our C# basics. Remember, a little recap never hurt anyone.
Converting C# Enum Values to Integers
So, are we ready now? Hold tight to your hats as we plunge straight into the magic of converting enums to ints. And of course, not just any regular way, but using some neat tricks and shortcuts I have up my sleeve.
Here is a quick example of how we can convert an enum to an integer using a cast:
Loading code snippet...
Common Mistakes to Avoid While Converting Enums to Ints in C#
Done already? Not so fast! Even the best of us stumble, and here I’ve compiled a handy list of common mistakes we all love to make. So let’s learn how to steer clear and master this conversion once and for all.
The Role of
Alrighty then! Time to dig deep into the machinations of the Enum.GetValues
method: a veritable Swiss Army knife in managing and converting enums to ints in C#. This method is like an ace up our sleeve, taking the ‘complexity’ out of conversions and making it smooth as a run down a ski slope on a sunny day. So, grab a cup of coffee (or two) because we’re about to dissect the vital bits and pieces of how Enum.GetValues
operates.
Using
Lost already? No stress, let’s break it down.
Let’s assume you’re creating a calendar app. You’ve got an enum
type to represent the days of the week.
Loading code snippet...
But how can we easily number these days? After all, the app needs to know that Sunday is the first day(0) and Saturday is the last day(6). This is where Enum.GetValues
shows its magic.
Loading code snippet...
Enum.GetValues
method creates an array, making it super easy for us to assign or print the numerical equivalents of each day. See like magic, right?
If you’ve a curious mind like mine, you’d be wondering, what if the enum’s actual values were different? Well, in that case:
Loading code snippet...
allScores
will now hold {1, 5, 10}
. Neat, huh?
A Quick Walkthrough on How
But, how does it do that? We’re all like curious cats here, so let’s peak under the hood. Enum.GetValues
method uses some .NET Framework sorcery to fetch the underlying integer values of the enum. It sees the enum as a special kind of integer, retrieves those values, and kindly hands them over to you in an array.
Let’s add a twist for our 8-year old friends:
Imagine, your enums are cans of differently flavored sodas, and Enum.GetValues
is the soda dispensing machine. When you tell Enum.GetValues(the name of your desired soda)
, it checks your choice, picks out the correct can (integer value), and plops it right into your hands (returns it as an array).
Role of
Wondering how to retrieve values based on enum names? Well strap on, buddy, we’re going on another ride.
The permutations and combinations do not stop as Enum.GetValues
allows you to convert an enum name to its respective integer value. Here’s how you do it:
Loading code snippet...
So, in this example, Enum.GetValues
retrieves all enum names, and the infamous casting method shows its charm to convert these enum names to respective integer values. Voila, we now know the integer equivalent of each vehicle in our enum.
Alternate Approaches to Convert C# Enum to Int
Programming is like having a shiny Swiss army knife. We have a plethora of tools and methods that accomplish the same job, yet each with its distinct charm. That way, there’s generally more than one nifty trick to solve a problem. So now, we’ll put enums under our magnifying glasses and examine the multiple approaches to convert C# enum to int.
How to Convert Enum to Int Using
You know how sometimes you have a number written in words and you want to, well, convert it into a number? Coding works pretty much the same way. Convert.ToInt32
is akin to a translation dictionary. It helps translate enums to ints flawlessly. Not sure how it works? Let’s break it down.
Loading code snippet...
Think of enums as a list of distinct elements. They possess an underlying integral numeric representation. In our case, ‘Sun’ is 0, ‘Mon’ is 1, and so forth. Convert.ToInt32
takes an enum as input and outputs its numeric equivalent.
Converting C# Enum to Int Using The Casting Approach
Didn’t like the translation idea? How about if I told you that we could, um, ‘persuade’ the enum to impersonate an int? That sounds fun, right? In the programming world, it’s known as casting.
This is how you do it:
Loading code snippet...
In plain ol’ English, we’re telling our C# program, “Hey, see this Days.Sun enum? Treat it as an int just this one time, alright?” The program complies, and the result is the same as before.
Converting Enum to Int: The Explicit and Implicit Conversion Way
Fancy more drama? Alright, buckle up for an adventure of playing both sides with explicit and implicit conversions. When you explicitly convert an enum to an int, you’re outright instructing the program to change forms. However, the implicit conversion is more of an undercover agent, seamlessly transitioning from enum to int in variable assignments.
Have a peek at these agents in action:
Loading code snippet...
Imagine a kid in a toy store. Explicit conversion is when you straight up tell your parents that you want that cool spaceship. Implicit conversion, on the other hand, is like subtly showing interest in that spaceship, and your parents, understanding your hint, get it for you.
Insights and Expert Tips on Performance Considerations for Int-Enum Conversion
Strapping on our developer goggles, reeling from the thrill of conversions, we suddenly recall an important player in this game – performance.
Oh yes, the ever imposing and persistently lurking question of performance is something we can’t ignore. So, let’s dive into the deep end and shed some light on how these enum conversions sway the performance of our beloved applications.
How Int to Enum Conversion Affects Your Application Performance?
Been eyeballing the stop-motion of your application lately and wondering, could it be the Int-Enum conversions? Let’s roll up our sleeves and scatter some breadcrumbs to navigate this performance maze.
To demonstrate, let’s consider an application where we have an enum for Status
with variables like Active
, Inactive
, Pending
, and so forth. When this application scales, and we’re dealing with hundreds of thousands of status conversions, you might notice a lag in the application performance. Here is an example:
Loading code snippet...
Keep in mind, every time the conversion happens, it requires processing, and this constant conversion could take a toll on your CPU usage with high volume applications.
Performance Optimization Techniques for Converting Enums to Integers
Is there a light at the end of this tunnel? Of course, where there’s a problem, there’s a solution. Let me whisper some developer’s secrets in your ears to keep the blues at bay.
Caching
In several scenarios, the values of enums aren’t going to change frequently, if at all. In such cases, instead of converting enums every single time, you could use caching which will store the converted integers, and use them as needed.
Loading code snippet...
Caching can significantly improve the application’s performance, especially when the conversions are repeated in high frequency.
Understanding the Impact of Conversion Tactics on Memory Utilization
We’ve beaten the CPU blues, but what about memory? Yes, conversion strategies also have a say in memory utilization and it’s our turn to give a listen.
Memory Footprint
Consider this, your application has 10 different Enums, and you’re converting these repeatedly to integers. Every time, it’s eating up a bit of memory. And when your application grows into a mammoth, it all starts to add up.
If you’re caching conversions, keep in mind that while you’re saving CPU resources, you’re occupying memory. So strike up a balance between conversion and caching based on your application needs.
Lazy Loading
You can also consider implementing lazy loading, which means that the conversions are only done when they’re required and not at application start-up.
Loading code snippet...
In this way, you are optimizing both CPU usage and memory utilization.
Testing the Conversion: Simple and Advanced Cases of Debugging Enum-Int Conversion
Well then, made it this far, fellow code aficionado? Way to go! Our journey, though, doesn’t end here. Once we have donned our capes and plunged headfirst into the world of conversions, it wouldn’t do to sit smugly without testing the fruits of our hard work.
So hang tight and prepare to dive deeper. You might be eager to flex your newfound knowledge and put it to work. But before we release it into the unforgiving wilderness of code, let’s play detective and put our Enum-Int conversions to the test.
Basic Debugging Scenarios for Validating Enum to Int Conversion
Starting off our debugging journey, we’ll be keeping it simple. The basics are, after all, our best friends. To set the stage, here’s an enum called Days
:
Loading code snippet...
Suppose you want to convert Wed
into its corresponding integer value. A straightforward cast would suffice:
Loading code snippet...
Bingo! Wed
is neatly converted to its integer equivalent 3
. But how do we ensure that our conversion went off without a hitch? Well, a simple line of code producing console output would reassure us:
Loading code snippet...
Remember fellow coders, simplicity is the ultimate sophistication, even in the world of debugging.
Complex Debugging Scenarios: Handling Conversion Exceptions and Errors
Got the hang of the basics? Great! But, what’s an adventure without a few surprises around the corner? Onto the murky waters of complex scenarios we go, tackling exceptions and errors and emerging victorious.
What if your enum values aren’t sequential starting with 0? Take a look at this, I bet you weren’t expecting it!
Loading code snippet...
To convert Medium
to an int, a simple cast would work, right?
Loading code snippet...
However, in large-scale applications, you don’t control all enums, and things might get messy. What if you tried converting a string to an enum? The following code will throw an exception:
Loading code snippet...
In these scenarios, it’s safer to use Enum.TryParse
, which won’t throw an exception for invalid input:
Loading code snippet...
Tips For Maintaining Stable Enum-Int Conversion in Large Scale Applications
Aha, you made it to the end! But hold on, we’ve got some loose ends to tie. Ensuring that your enum-int conversion remains stable in large-scale applications can be quite a feat, but I’ve got you covered. Here are the ever-faithful tips that never disappoint:
- Always validate your conversions: Use
Enum.TryParse
to avoid nasty surprises in the form of exceptions. - Know your enum: Avoid assumptions. Enum members can hold any value their underlying type can hold, not just sequential ints. Always be aware of what you’re dealing with.
- Watch for duplicates: Enums can have members with the same value. So, keep an eye out to dodge potential confusion.
- Leverage the power of the IDE: Modern IDEs provide tools that can help detect possible conversion errors. Use them.
- Test, Test, and Test again: Write extensive unit tests to test the enum conversion from all angles – valid values, invalid values, boundaries, and so on.
With these trusty tips and tricks up your sleeve, take heart in knowing that you’re well equipped to maintain stable enum-int conversion.
Alright, hats off to you! You’ve braved the storm, lived to tell the tale, and learned (hopefully!) a few new tricks. Always remember that practice makes perfect. So, what are you waiting for? Start coding! But hey, don’t forget to have some fun along the way.