ASP.NET Web Forms Interview Questions and Answers
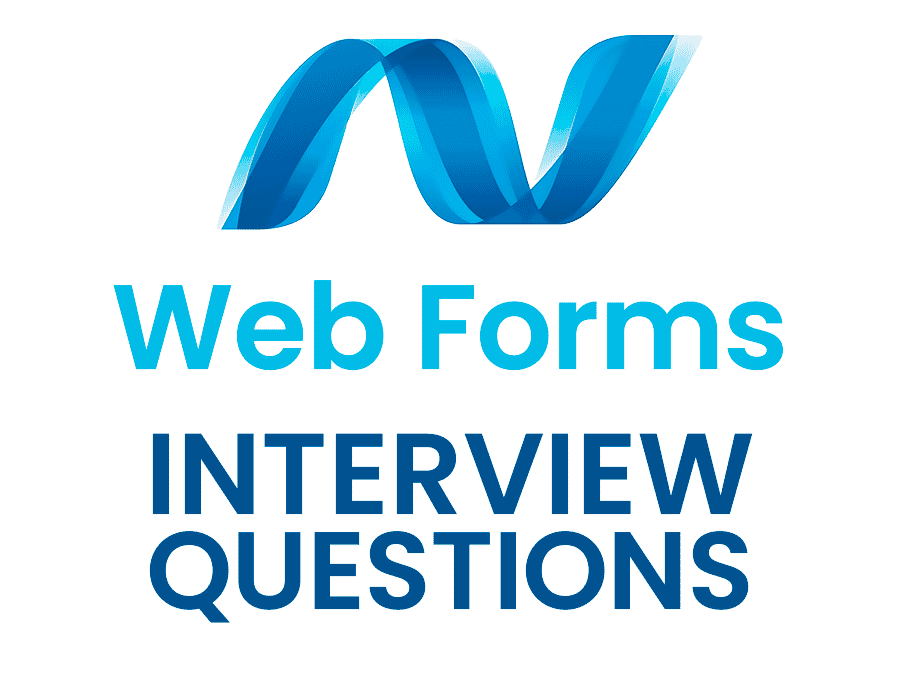
If you’re preparing for an ASP.NET Web Forms interview, you’ve come to the right place. In this comprehensive guide, we’ve compiled the most frequently asked ASP.NET Web Forms interview questions to help you ace your upcoming interview.
Providing detailed answers for each question, this article serves as an invaluable resource to both beginners and experienced developers looking to brush up on their knowledge.
Whether you’re aiming to step up your career or simply expand your understanding of ASP.NET Web Forms, these web forms interview questions and answers will help you navigate even the toughest asp net web forms interview.
How does the ViewState mechanism work in ASP.NET Web Forms, and what is its significance in maintaining the state of controls across postbacks?
Answer
- ViewState is a client-side state management mechanism used in ASP.NET Web Forms to preserve the state of controls across postbacks.
- When a user interacts with a web page (e.g., submits a form), the page is sent to the server for processing, and then the server sends the updated page back to the user. This is called a postback.
- By default, HTML controls do not maintain their states across postbacks, which means their values are lost when the page is sent to the server. In ASP.NET Web Forms, ViewState is used to solve this issue.
- On a postback, ViewState stores the state of the controls in a hidden field and sends it to the server alongside the page’s other data. The server then uses this ViewState data to recreate the state of the controls.
How ViewState works:
- During the initial page load, the ViewState is empty.
- When a control’s property value changes, ASP.NET updates the ViewState to store the new value.
- Before rendering the page, the ViewState data is serialized, encrypted (if configured), and stored in a hidden field on the page.
- When the user submits the page (postback), the ViewState data is sent to the server, along with the other page data.
- On the server, the ViewState is deserialized, decrypted (if encrypted), and used to set the control’s properties.
- The page life cycle continues, and the control maintains its state across postbacks.
Explain the difference between server-side and client-side state management techniques in ASP.NET Web Forms. Provide examples of specific techniques for both server-side and client-side state management.
Answer
In ASP.NET Web Forms, state management techniques can be divided into two categories: server-side and client-side.
Server-side state management:
- State data is stored on the server and can be kept in memory or persisted to external storage like a database.
- Examples of server-side techniques:
- Session State: Stores data for the duration of a user’s session on the website, and is managed by the server. Session state uses a session ID to uniquely identify each user.
- Application State: Stores data that is shared across all user sessions for an application. It can be used to store site-wide settings, global variables, and counters.
Client-side state management:
- State data is stored in the client’s browser and sent back to the server when needed.
- Examples of client-side techniques:
- ViewState: As explained earlier, it is a hidden field on the page that maintains the state of controls across postbacks.
- Cookies: Small text files stored in the user’s browser containing key-value pairs. Cookies can persist across multiple visits and are sent to the server with each request.
- Query Strings: Data appended to the URL as key-value pairs. Query strings can be used to pass information between pages, but are limited in size and should not be used to store sensitive information.
What are the performance implications of using large ViewStates in an ASP.NET Web Forms application? How can you mitigate the negative effects of large ViewStates?
Answer
Using large ViewStates can have several performance implications in an ASP.NET Web Forms application:
- Increased page size: ViewState data is stored in a hidden field on the web page, and large ViewState data can significantly increase the page size, leading to slower page load times for users.
- Increased bandwidth usage: Large ViewState data must be transferred from the server to the client browser and back during each postback, consuming more bandwidth and potentially increasing the response time.
- Higher server-side processing time: Serialization, deserialization, encryption, and decryption of large ViewState data may require more server-side processing time, affecting server performance.
To mitigate these negative effects:
- Disable ViewState for unnecessary controls: Disable ViewState for controls that do not need to maintain their state across postbacks, such as static labels or placeholders.
- Store ViewState information in a compressed format: Compress the ViewState data to reduce its size and minimize the bandwidth consumed during transmission.
- Store ViewState on the server: In cases where the ViewState is still large, even after optimization, consider storing the ViewState data on the server. This can be achieved using third-party libraries or by creating custom ViewState storage providers.
- Enable ViewState encryption only when necessary: Encrypting the ViewState increases its size and adds processing overhead. Use encryption only when it is necessary to protect sensitive information.
- Opt for alternative state management techniques: For large amounts of data, consider using other state management techniques like Session State or Application State, which can store data on the server more efficiently.
Describe the Page Life Cycle in ASP.NET Web Forms. Mention the order and significance of the various events that occur during the entire lifecycle.
Answer
The Page Life Cycle in ASP.NET Web Forms comprises multiple stages and events that occur in a particular order. Understanding the life cycle allows developers to write code at appropriate events and effectively manage control states, user interactions, and error handling.
The stages and events of the Page Life Cycle are:
- Page Request: The user requests a page. If it’s the first request, a new instance of the page class is created.
- Start: Page properties like Request, Response, and IsPostBack are set in this stage.
- Page Initialization: Controls on the page are initialized, their unique IDs are set, and themes and master pages are applied. The
Init
event is raised for each control, including the Page. - Load ViewState: The ViewState data from the previous postback is loaded into the controls.
- Load Postback Data: Data submitted by the user during the postback is loaded into the controls. The
LoadPostData
event is raised for controls that implementIPostBackDataHandler
. - Page Load: The
Page_Load
event is raised in this stage. Developers can write code to set the initial state of controls, perform calculations, and load data based on user actions or query string parameters. - Control Event Handling: Events triggered by user interaction, like button clicks or list selection changes, are raised and handled in this stage.
- Page Validation: Input validation is performed using validation controls, and the
IsValid
property is set. Developers can further validate user input in this stage. - Postback Event Handling: If the validation is successful and any relevant events are raised, the corresponding event handlers are executed (e.g., button click or dropdown selection change).
- Page Rendering: The controls are prepared for rendering into HTML. The
PreRender
andPreRenderComplete
events are raised for the controls and the page. This is the last chance to change the control properties before they are converted into HTML. - Save ViewState: The ViewState data for the controls is serialized, encrypted (if configured), and saved in a hidden field on the page.
- Control Rendering: The controls are rendered into HTML and combined with the ViewState data. The
Render
method is called for each control. - Unload: The
Unload
event is raised for all controls and the page. Cleanup code and resource disposal can be performed in this stage.
How can you implement Master Pages and Content Pages in ASP.NET Web Forms? Explain their advantages and best practices for organizing complex applications.
Answer
In ASP.NET Web Forms, Master Pages and Content Pages are used to create a consistent layout and structure for an application by sharing common UI elements, styles, and scripts.
Master Pages:
- Contain the shared layout, navigation, header, and footer elements for the application.
- Include one or more ContentPlaceHolder controls, which define the areas where the content pages can inject their content.
Content Pages:
- Contain the content-specific UI elements and code-behind logic.
- Include a Content control for every ContentPlaceHolder defined in the Master Page.
Advantages of using Master Pages and Content Pages:
- Maintain a consistent look and feel across the application.
- Reduce code duplication, making maintenance and updates easier.
- Increase the scalability and organization of complex applications.
Best practices for organizing complex applications with Master Pages and Content Pages:
- Separate layout and functionality: Use Master Pages to define the layout and common UI elements, and Content Pages to manage content-specific functionality.
- Reuse Master Pages: Create multiple Master Pages for different application sections or user roles, and reuse the Master Pages across related Content Pages.
- Use nested Master Pages: In highly complex applications, use nested Master Pages to define hierarchical relationships and further modularize the page layouts.
- Define descriptive ContentPlaceHolder IDs: Use meaningful and descriptive IDs for the ContentPlaceHolder controls so that developers can easily understand the purpose of each content area.
- Organize resources: Keep styles, scripts, and other resources in separate folders and use a consistent naming convention to facilitate maintainability and organization.
As we transition from discussing Master and Content Pages to other essential aspects of ASP.NET Web Forms, it’s important to remember that understanding various techniques and features can optimize your development process.
The next set of questions focuses on postbacks, update panels, and cross-page postbacks, providing a well-rounded perspective for your upcoming asp net web forms interview.
In Web Forms, what are the implications of using synchronous vs. asynchronous postbacks? How does this compare to the update panel control used in AJAX?
Answer
In ASP.NET Web Forms, postbacks can be performed either synchronously or asynchronously.
Synchronous Postbacks:
- Default behavior in Web Forms.
- The entire page is sent to the server, processed, and returned to the client.
- The browser’s UI becomes temporarily unresponsive during the roundtrip, which can lead to a poor user experience, especially in slow networks.
Asynchronous Postbacks:
- Partial postback, where only a portion of the page is updated without refreshing the whole page.
- Improves user experience by allowing users to continue interacting with the page while the server processes the postback.
- Requires additional client-side code to manage the asynchronous request and update the relevant portions of the page.
UpdatePanel Control and AJAX:
- The UpdatePanel control is part of the ASP.NET AJAX framework, which provides an easy way to implement asynchronous postbacks in Web Forms.
- By placing content inside the UpdatePanel, the content is automatically refreshed asynchronously during a postback without updating the whole page.
- The UpdatePanel can improve user experience by reducing the time it takes to update parts of the page; however, it comes with some limitations:
- The ViewState is still sent with each asynchronous postback, increasing the request size and bandwidth usage.
- Overusing UpdatePanels can lead to complex, harder-to-maintain application code.
In summary, choosing between synchronous, asynchronous postbacks, and UpdatePanel control depends on the specific needs of the application and the desired user experience.
Explain the concept of “cross-page postbacks” in ASP.NET Web Forms and how they can be utilized in complex applications.
Answer
In ASP.NET Web Forms, cross-page postbacks refer to a postback operation where data is submitted from one page (source) to another page (target) instead of posting the data back to the same page.
Cross-page postbacks can be useful in scenarios such as multi-step forms, where each step is a separate Web Form, and the data from all steps needs to be submitted to the final target page for processing.
To implement cross-page postbacks:
- Set the
PostBackUrl
property of the control that triggers the postback (e.g., a button) to the target page’s URL. - In the target page, retrieve the values of the source page’s controls using the
Page.PreviousPage
property, which returns an instance of the source page.
Advantages of using cross-page postbacks:
- Separation of concerns and better organization of complex applications.
- Allows for independent validation and processing of each step in multi-step forms.
What is the role of the Global.asax file in an ASP.NET Web Forms application? Explain its significance and the various events that can be handled within it.
Answer
The Global.asax
file, also known as the “global application class,” is an optional file in an ASP.NET Web Forms application that can be used to define and handle application-wide and session-related events.
The main responsibilities of the Global.asax
file include:
- Application-level event handling.
- Session-level event handling.
- Management of global objects and variables.
Some of the common events that can be handled within Global.asax
are:
- Application-level events:
Application_Start
: Fires when the application starts; used for initialization tasks like populating cache, loading settings, or registering routes.Application_End
: Fires when the application shuts down; used for cleanup tasks like closing database connections and releasing resources.Application_Error
: Fires when an unhandled exception occurs within the application; used for global error handling and logging.Application_BeginRequest
: Fires with each HTTP request just before it is processed; used for tasks like URL rewriting or acquiring resources needed for request processing.Application_EndRequest
: Fires with each HTTP request after processing is completed; used for tasks like releasing resources or modifying the response.
- Session-level events:
Session_Start
: Fires when a new user session starts; used for initializing session-level data or settings.Session_End
: Fires when a user session ends, either by timeout or explicitly; used for cleanup tasks like releasing session resources or updating user activity logs.
The Global.asax
file serves a significant role in maintaining centralized application configuration, error handling, and resource management for ASP.NET Web Forms applications.
Describe the various ways to handle exceptions in ASP.NET Web Forms applications. Explain the differences between global exception handling, page-level exception handling, and control-level exception handling.
Answer
In ASP.NET Web Forms applications, exceptions can be handled at various levels to ensure proper error handling, logging, and user experience.
- Global Exception Handling:
- Provides a centralized way to handle exceptions throughout the application.
- The
Application_Error
event in theGlobal.asax
file can be used to catch unhandled exceptions and perform error logging, notifying administrators, or displaying a custom error page to the user. - Global exception handling should be used as a catch-all mechanism for unhandled exceptions that are not caught at the page or control levels.
- Page-Level Exception Handling:
- Suitable for handling exceptions specific to a single page.
- The
Page_Error
event is used to handle exceptions that occur within a specific page’s execution. - Page-level exception handling allows for more detailed error messages or recovery actions for particular scenarios tied to a specific page.
- Control-Level Exception Handling:
- Appropriate for handling exceptions that occur within a specific control, like a user control, custom control, or grid view.
- Exceptions can be captured and handled within the control’s event handlers, such as click events or data binding events.
- Control-level exception handling can provide more granular error messages or actions relevant to the control’s operation.
In general, a mix of global, page-level, and control-level exception handling should be used to provide appropriate error handling, logging, and user feedback based on the specific requirements of the application.
What is the role of the HttpHandlers and HttpModules in an ASP.NET Web Forms application, and how can they be utilized for various functionalities such as URL rewriting, caching, and compression?
Answer
HttpHandlers
and HttpModules
are two components in the ASP.NET Web Forms pipeline that allow developers to intercept and process HTTP requests and responses.
HttpHandlers:
- Act as the endpoint that processes an HTTP request and generates the final response.
- Each handler is associated with specific file types or URL patterns.
- Custom HttpHandlers can be created to process specific types of requests, such as generating dynamic images or processing custom file formats.
HttpModules:
- Intercept and modify HTTP requests and responses at various stages of the request pipeline.
- Allow developers to implement custom logic, such as authentication, logging, error handling, or modifying the request/response headers.
- Multiple HttpModules can be chained together to perform different tasks in the request pipeline.
HttpHandlers and HttpModules can be utilized for various functionalities:
- URL Rewriting: HttpModules can be used to implement URL rewriting by intercepting the request and changing the requested URL to a different one, based on predefined rules or patterns.
- Caching: HttpModules can be used to manage caching of static and dynamic content, such as setting cache duration, cache location, or managing cache dependencies.
- Compression: HttpModules can be utilized to compress the response content, such as using gzip or deflate, to reduce the response size and improve website performance.
To use HttpHandlers or HttpModules in an ASP.NET Web Forms application, you need to:
- Create a custom class that inherits from
IHttpHandler
(for HttpHandlers) orIHttpModule
(for HttpModules). - Implement the required methods and properties to carry out the desired functionality.
- Register the custom handler or module in the application’s Web.config file, associating it with the required file types, URL patterns, or request pipeline stages.
By using HttpHandlers and HttpModules, developers can gain fine-grained control over the request and response processing in an ASP.NET Web Forms application, allowing them to implement custom functionality and optimizations efficiently.
Now that we’ve covered HttpHandlers and HttpModules in-depth, let’s explore other vital functionalities of ASP.NET Web Forms that are essential for a successful interview.
In the next segment, we dive into custom server controls, dynamic data binding, and templated controls, equipping you with the knowledge to answer any net web forms interview questions on these topics.
In ASP.NET Web Forms, how can you build a custom server control and integrate it into your application? Explain the steps and benefits of using custom server controls.
Answer
Custom server controls in ASP.NET Web Forms are reusable components that encapsulate UI and logic for specific functionalities, making it easier to maintain and reuse code across different pages and applications.
To build a custom server control, follow these steps:
- Create a new class: Create a new class that inherits from
System.Web.UI.WebControls.WebControl
,System.Web.UI.WebControls.CompositeControl
, or another appropriate base class, depending on the desired functionality. - Define properties: Define properties to manage the state and behavior of the control. Use attributes like
Browsable
,Category
, andDefaultValue
to control how the properties appear in design-time tools and the default values. - Override methods: Override methods such as
Render
,OnInit
,OnPreRender
, orOnLoad
to implement the control’s logic, user interface, and any required event handling. - Add child controls (if necessary): For composite controls, create and add child controls to the control’s
Controls
collection, and manage their state and events. - Compile: Compile the control into a library that can be distributed as a separate DLL or included in the application’s bin folder.
To integrate the custom server control into an application:
- Register the control: Register the control in the application’s Web.config file or on an individual page using the
<%@ Register %>
directive. - Add the control to a page: Use the control’s tag on a Web Forms page, set its properties, and handle any required events.
Benefits of using custom server controls:
- Encapsulate functionality and markup in a reusable component, reducing code duplication and improving maintainability.
- Simplify complex UI logic by organizing it into a single, cohesive control.
- Provide a consistent look and behavior across the application or multiple applications.
- Enable better design-time support and easier integration with the Visual Studio toolbox.
Describe dynamic data binding and templated controls in ASP.NET Web Forms. Explain how they can improve the data manipulation experience for users.
Answer
Dynamic data binding and templated controls in ASP.NET Web Forms allow developers to create more advanced user interfaces for displaying and manipulating data, providing greater control over the presentation and behavior of data-bound content.
Dynamic Data Binding:
- Refers to the process of binding data from a data source, such as a database, XML file, or object model, to UI elements on a page at runtime.
- Data-binding expressions can be used to bind properties of controls (such as
Text
,ImageUrl
, orVisible
) to fields from the data source. - Supports two-way data binding, allowing user changes to be automatically synchronized with the data source.
Templated Controls:
- Controls that allow developers to define customizable templates for different parts of the control, which can be modified to match the desired appearance and behavior of the data-bound content.
- Examples of templated controls include
Repeater
,DataList
,GridView
,FormView
, andDetailsView
. - Templates can include static HTML, server controls, and data-binding expressions to create a flexible and customizable UI.
Dynamic data binding and templated controls can improve the data manipulation experience for users by:
- Customizing the UI: Allow developers to create custom layouts, formats, and styles for data presentation, making it easier for users to understand and interact with the data.
- Reducing code duplication: Encapsulate common data display and manipulation behaviors in reusable controls, simplifying the development and maintenance process.
- Increasing productivity: Leverage built-in features such as sorting, paging, editing, and deleting, reducing the amount of custom code needed to implement these functionalities.
- Enhancing interactivity: Enable rich client-side interactions and updates using AJAX and client-side data-binding features, providing a better user experience.
How can you implement authentication and authorization in ASP.NET Web Forms? Explain the various mechanisms such as Forms Authentication, Windows Authentication, and custom user authentication.
Answer
Authentication and authorization in ASP.NET Web Forms help secure access to application resources and functions by validating user identities and controlling access to resources based on user roles.
There are several mechanisms available to implement authentication and authorization:
- Forms Authentication:
- Uses HTML forms and cookies to store and validate user credentials entered on a custom login page.
- Ideal for internet-facing applications where users have their own login credentials.
- The
FormsAuthentication
class is used to manage the authentication process, such as encrypting/decrypting authentication tokens, setting cookies, and redirecting users to the login page. - User roles can be stored in the
RoleManager
module for controlling access to resources.
- Windows Authentication:
- Leverages the user’s Windows credentials (Domain/Active Directory) to authenticate and authorize access to application resources.
- Suitable for intranet applications where users are part of a Windows domain.
- Requires minimal application-level code to implement, as authentication and role information are managed by the operating system and the web server (IIS).
- Access to resources can be controlled using Windows groups and the
Authorization
section in the Web.config file.
- Custom User Authentication:
- Designed for applications with unique authentication requirements or when integrating with external identity providers (such as social media logins or Single Sign-On services).
- Requires implementing custom logic for user validation, role management, and session management.
- Can be combined with the ASP.NET Membership and Roles features or third-party libraries for a more comprehensive solution.
To implement authorization, you can control access to resources within your application by:
- Using the
Authorization
section in the Web.config file to define access rules based on URL patterns and user or role names. - Implementing custom logic within event handlers, such as
Page_Load
orPage_Init
, that checks user identity and roles before allowing access to certain functionality or resources.
In summary, ASP.NET Web Forms offers several mechanisms for implementing authentication and authorization to secure application resources and functions.
How does the ASP.NET routing system work in Web Forms? Explain the significance of URL Routing and its advantages over traditional URL-based navigation.
Answer
ASP.NET routing in Web Forms is a powerful feature that decouples the relationship between the URL structure and the physical file layout of an application, enabling developers to create more user-friendly and SEO-friendly URLs.
URL routing works by:
- Defining a set of route patterns that map to a specific Web Form (page) or handler.
- Intercepting incoming requests and processing them through the routing system.
- Matching the request’s URL to a defined route pattern and forwarding the request to the associated Web Form.
- Making route data (such as URL parameters) available to the Web Form for processing.
The main advantages of URL routing over traditional URL-based navigation are:
- User-friendly-URLs: Routing allows for the creation of more meaningful and easily understandable URLs, improving usability and search engine indexing.
- SEO-friendly: By using meaningful URLs and proper structure, routing can help improve the search engine ranking of the application.
- Flexibility: Routing provides the ability to update the URL structure or application layout without affecting the user-facing URLs or breaking external links.
- Abstraction: Routing helps maintain separation between the URL scheme and the application’s file structure, promoting better organization and maintainability.
To implement URL routing in an ASP.NET Web Forms application, follow these steps:
- Enable routing: Add a reference to
System.Web.Routing
in the project and enable the routing feature in the Global.asax file by callingRouteTable.Routes.Add
method in theApplication_Start
event. - Define route patterns: Create route patterns in the Global.asax file, associating them with the corresponding Web Forms (pages) and specifying any required URL parameters.
- Access route data: In the Web Form (page), use the
Page.RouteData
property to access the URL parameters and route data for processing.
By implementing the ASP.NET routing system in Web Forms, developers can achieve more user-friendly, flexible, and maintainable URL schemes for their applications.
Explain the role of Globalization and Localization in ASP.NET Web Forms. How can you create culture-specific resources and display them based on the user’s language preferences?
Answer
Globalization and localization in ASP.NET Web Forms enable developers to adapt their applications for different languages, regions, and cultures, providing a more customized and accessible experience for users worldwide.
Globalization: Refers to designing and preparing an application to support multiple cultures, languages, and regions, including handling various date formats, number formats, and text directions (LTR/RTL).
Localization: Involves translating the application’s UI, content, and resources (such as text strings, images, and error messages) into multiple languages and making them available based on the user’s language preference or browser settings.
To create culture-specific resources and display them based on user preferences, follow these steps:
- Prepare the application: Use globalized data types, such as
DateTime
,NumberFormatInfo
, andCultureInfo
, to manage culture-sensitive data and operations, accommodating different date, number, and text formats. - Create resource files: Create separate
.resx
resource files for each supported language or culture, containing translated text strings, images, and other resources. - Apply resources to controls: In Web Forms, use the
Localize
andResourceExpressionBuilder
objects to bind control properties and content to resources from the appropriate resource file. - Set the current culture: At runtime, determine the user’s language preference (using the
Request.UserLanguages
property or custom logic) and set theThread.CurrentThread.CurrentCulture
andThread.CurrentThread.CurrentUICulture
properties accordingly. - Configure the application: In the Web.config file, enable the
globalization
element and configure the desired settings, such as the default culture, resource fallback behavior, and file encoding.
By incorporating globalization and localization in an ASP.NET Web Forms application, developers can create a more inclusive and accessible experience for users around the world, tailoring their applications to different languages, regions, and cultural preferences.
As we’ve delved into the role of Globalization and Localization in ASP.NET Web Forms, it’s time to shift gears towards validation mechanisms — an indispensable component of any web application.
In this part of our asp net web forms interview questions and answers, we’ll discuss Custom Validators, Custom Validation Groups, and best practices for implementing them in your application.
What is the difference between Custom Validators and Custom Validation Groups in ASP.NET Web Forms? Explain their implementation techniques and use cases.
Answer
Custom Validators and Custom Validation Groups in ASP.NET Web Forms provide enhanced validation functionality for the application, ensuring that user input complies with specific requirements and business rules before being processed by the application.
Custom Validators:
- Allow developers to create their own validation logic and error messages, which are not available in the built-in validator controls.
- Can be used for complex validation scenarios, such as regular expressions, date comparisons, or conditional validation based on multiple input fields.
- To implement a Custom Validator, add the
CustomValidator
control to the form, specify the validation control’sControlToValidate
property, and define theOnServerValidate
server-side event handler or theClientValidationFunction
client-side function to write your custom validation logic. - The custom validation function should return a boolean value indicating whether the validation passed or failed and set the
ErrorMessage
property in case of a failure.
Custom Validation Groups:
- Allow developers to group validator controls and associate them with a specific input field or set of input fields.
- Useful for scenarios where multiple input fields need to be validated independently or when multiple submit buttons perform different validation actions.
- To implement a custom validation group, set the
ValidationGroup
property of the appropriate validator controls and associate them with the corresponding input field(s) and submit button(s).
In summary, Custom Validators provide the ability to create custom validation logic for complex scenarios, while Custom Validation Groups allow grouping validators for better organization and control over the form’s validation.
Describe how to implement caching in ASP.NET Web Forms to improve application performance. Explain the different caching approaches such as output caching, data caching, and control caching.
Answer
Caching in ASP.NET Web Forms is used to store and reuse frequently accessed data, resources, or rendered content, reducing the time and resources required to process requests and improving overall application performance.
There are several caching approaches in ASP.NET Web Forms:
- Output Caching:
- Stores the HTML generated by a page or user control for a specified duration, reducing the need to regenerate the content for subsequent requests.
- Implemented using the
OutputCache
directive in the page or control markup, with properties such asDuration
,VaryByParam
,VaryByControl
, andCacheProfile
to control the caching behavior. - Can be further customized using substitution and post-cache substitution to dynamically update portions of the cached content.
- Data Caching:
- Stores frequently accessed data (e.g., database query results or Web API responses) in memory, minimizing the time spent on data retrieval and processing.
- Implemented using the
Cache
object or theSystem.Runtime.Caching
namespace to add, retrieve, or remove data from the cache, typically with an expiration policy or dependency. - Cache data can be stored on different levels – application, session, or per-user basis, depending on the desired scope and lifetime of the cached data.
- Control Caching (Partial Page Caching):
- Caches rendered output of individual user controls, allowing for better granularity and flexibility in caching specific sections of a page.
- Implemented using the
PartialCaching
attribute in the user control class or theOutputCache
directive within the control markup, with similar properties and behaviors as output caching above. - Suitable for scenarios where only specific parts of a page need to be cached, rather than the entire page.
By implementing caching techniques in an ASP.NET Web Forms application, developers can improve overall performance, reduce server load, and provide a faster and more responsive user experience.
What are the constraints and challenges of implementing SEO-friendly URLs in an ASP.NET Web Forms application?
Answer
Implementing SEO-friendly URLs in ASP.NET Web Forms applications is critical for improving search rankings and making the application more accessible to users. However, there are several constraints and challenges involved in achieving this goal:
- Legacy URL structure: In traditional Web Forms applications, the URL structure is closely tied to the physical file layout (e.g.,
example.com/pages/products.aspx
). Implementing SEO-friendly URLs (e.g.,example.com/products/123
) may require significant changes to the application’s structure and navigation logic. - Routing limitations: Although ASP.NET Web Forms support URL routing, it is not as robust or flexible as modern frameworks like ASP.NET MVC or .NET Core, potentially requiring custom routing logic or HttpModules to handle complex URL patterns and rewrites.
- Dynamic content: Web Forms applications often rely on query string parameters to display dynamic content (such as
products.aspx?category=electronics
). Implementing SEO-friendly URLs for dynamic content may require changes to the data retrieval and rendering logic to support the new URL structure. - State management: ViewState and control state values, often included in the URL or submitted forms, can impact the SEO-friendliness of application URLs. Developers need to minimize the use of ViewState and ensure that important state information is included in the SEO-friendly URLs.
- Backward compatibility: When updating existing applications to use SEO-friendly URLs, developers must ensure that old URLs are properly redirected to the new format, and existing external links are maintained.
Despite these challenges and constraints, it is possible to implement SEO-friendly URLs in ASP.NET Web Forms applications by leveraging features such as URL routing, HttpModules, and custom navigation logic.
Explain how to ensure accessibility and compliance with web standards in an ASP.NET Web Forms application. What are some tools available to help developers achieve this?
Answer
Ensuring accessibility and compliance with web standards in an ASP.NET Web Forms application involves several best practices and techniques, making the application more user-friendly and accommodating a diverse range of user abilities and needs.
To ensure accessibility and compliance, consider the following steps:
- Write semantic markup: Use proper and meaningful HTML tags (e.g., headings, lists, and ARIA roles) to convey the structure and meaning of the content.
- Provide alternative content: Include alternative text for images, transcripts for audio, and captions for video content, making them accessible to users with various disabilities.
- Design for keyboard navigation: Ensure that all functionality and controls are accessible through keyboard input for users who cannot use a mouse or have limited motor control.
- Use adaptable design: Develop responsive and fluid layouts that adapt to different screen sizes, orientations, and devices, providing a consistent experience for all users.
- Test with different browsers and devices: Ensure compatibility with different browsers, devices, and assistive technologies by testing the application in various environments.
Several tools can help developers achieve accessibility and compliance with web standards:
- W3C Markup Validation Service: Validates the HTML markup of pages and identifies any issues or non-compliant elements.
- W3C CSS Validation Service: Validates the CSS stylesheets and identifies any issues or non-compliant rules.
- Web Accessibility Evaluation Tool (WAVE): Analyzes web pages for accessibility issues and provides suggestions for improvements.
- Color Contrast Analyzer: Helps ensure that text and background colors have sufficient contrast for users with different vision abilities.
By incorporating accessibility and web standards compliance in ASP.NET Web Forms applications, developers can create a more inclusive and user-friendly experience for a diverse range of users while also ensuring compatibility with various browsers and devices.
How can you integrate client-side frameworks like Angular, React, and Vue.js in an existing ASP.NET Web Forms application to leverage modern web development techniques?
Answer
Integrating client-side frameworks like Angular, React, and Vue.js in an existing ASP.Web Forms application can help improve the user interface, responsiveness, and overall user experience by leveraging modern web development techniques and patterns. Here are some steps to integrate these frameworks with your existing Web Forms application:
- Choose a specific area or functionality: Identify a specific part of the application that can benefit from using a client-side framework, such as a dynamic data grid, real-time notifications, or rich interactive forms.
- Setup the development environment: Set up the development environment and required toolchain for the selected client-side framework, such as Node.js, NPM, Webpack, or Babel.
- Configure the build process: Modify the project’s build process (e.g., MSBuild, Gulp, or Grunt) to integrate the compilation, bundling, and minification of the front-end framework assets.
- Create API endpoints: Expose server-side data and functionality through RESTful API endpoints or using services such as SignalR for real-time communication, which can be consumed by the client-side framework.
- Develop the client-side logic: Develop the required components, directives, or services using the selected client-side framework, utilizing its capabilities for data binding, componentization, and other modern web development techniques.
- Integrate with Web Forms: Embed the client-side framework’s application or components within the existing Web Form(s) using a combination of static HTML, server-side controls, and JavaScript. You may use a
Literal
control to include a placeholder in the markup for mounting the client-side framework or injecting the bundled JavaScript and CSS files.
By integrating client-side frameworks like Angular, React, or Vue.js in an existing ASP.NET Web Forms application, developers can leverage modern web development techniques and improve the user interface, responsiveness, and overall user experience, while still maintaining the existing back-end logic and infrastructure.
Congratulations! You have reached the end of our comprehensive list of ASP.NET Web Forms interview questions and answers. We hope that this resource has provided you with the necessary knowledge to excel in your next ASP.NET Web Forms interview.
Remember, being well-prepared and having a deep understanding of the ASP.NET Web Forms framework will go a long way in building confidence and showcasing your skills to potential employers.
It’s important to keep learning and improving — so keep practicing, and best of luck in your asp net web forms interview!