ASP.NET Session Management Interview Questions and Answers
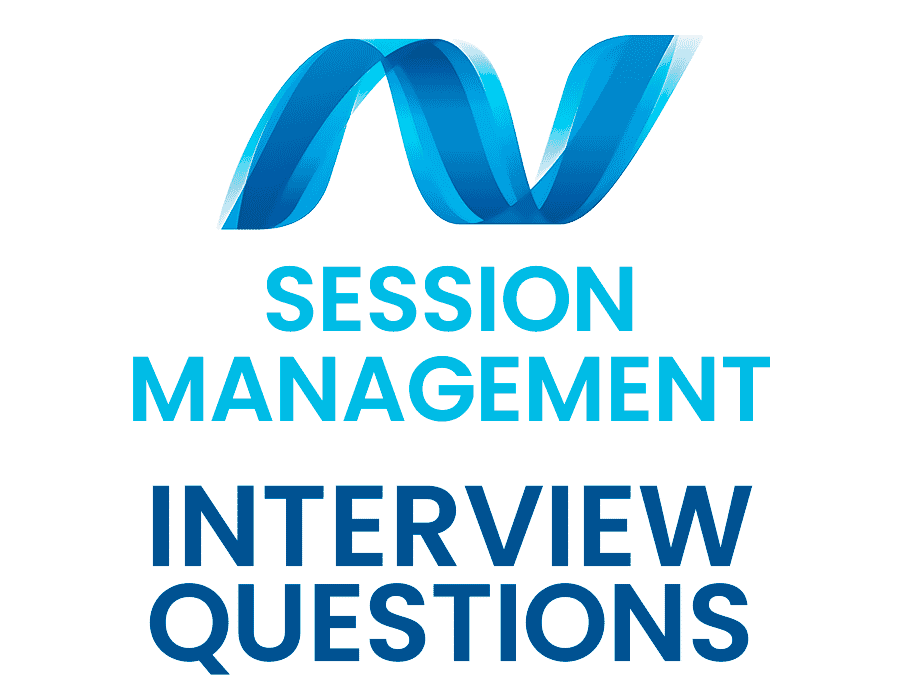
As a seasoned ASP.NET developer, you know the importance of session management in crafting robust and efficient web applications. No matter your expertise, preparing for job interviews can be daunting, and making an impression on your interviewer can be the key to landing your dream role.
To help you stand out, we’ve compiled the essential session management in ASP.NET interview questions and answers that showcase your depth of knowledge and problem-solving skills.
Whether you’re a junior, mid-level, or senior developer, this comprehensive guide will ensure you’re ready to tackle any question about ASP.NET session management with confidence.
What is the primary advantage of using InProc session state mode in ASP.NET compared to using a dedicated external session state server or a database?
Answer
The primary advantage of using InProc session state mode in ASP.NET compared to using a dedicated external session state server or a database is:
- Performance: InProc session state mode stores session data in the web server’s memory. This approach provides faster access to data when compared to external session state storage options (like StateServer or SQLServer), which require additional network calls and the overhead of data serialization and deserialization.
However, there are some drawbacks to using the InProc mode:
- Scalability: InProc is not the best choice for web applications that need to run in a web farm or a load-balanced environment, as session data is stored locally on each web server. This could lead to inconsistencies between servers and loss of session data if one of them fails.
- Memory usage: Since session data is stored in the web server’s memory, a large number of concurrent users with significant session data can consume substantial memory resources, potentially affecting the overall performance of the web application.
Explain the process of serializing and deserializing session data in ASP.NET when using StateServer or SQLServer mode for session management.
Answer
When using StateServer or SQLServer mode for session management, session data needs to be serialized and deserialized when saving and retrieving values. This allows the session data to be transmitted and stored across network and databases, respectively.
Serialization process:
- When the session data is about to be saved (e.g., at the end of a request), the session data objects are serialized into binary format using a formatter such as the
BinaryFormatter
. - Serialized data is then transmitted and stored in the State Server (for StateServer mode) or SQL Server (for SQLServer mode).
Deserialization process:
- When session data is required during the request processing, the data is retrieved from the State Server or SQL Server.
- The binary formatted session data is deserialized back into objects using the same formatter (e.g.,
BinaryFormatter
), making the session data available to the web application.
It’s essential to ensure that any custom objects stored in the session are marked with the [Serializable]
attribute, so they can be correctly serialized and deserialized.
How does cookieless session state management differ from standard session state management in ASP.NET, and what are its main drawbacks?
Answer
In standard session state management, ASP.NET uses cookies to store and retrieve a unique session identifier (SessionID) to associate with each user’s session data on the server.
Cookieless session state management, on the other hand, does not rely on cookies for maintaining the SessionID. Instead, it appends the SessionID directly to the URL of the web application.
Here are the main drawbacks of using cookieless session state management:
- URL manipulation: The visibility of SessionID in the URL makes it prone to manipulation by users or exposure through sharing or browser history, potentially creating security risks.
- Usability: Having long, cryptic SessionID values in the URL can make the web application’s URLs look less user-friendly and harder to share or bookmark.
- Search engine indexing: Search engine crawlers might index multiple URLs with different SessionIDs, leading to duplicate content issues and inefficient use of crawl budgets.
What are the critical factors to consider when choosing the session state mode (InProc, StateServer, SQLServer, or Custom) for a high-performance, scalable web application?
Answer
When choosing the session state mode for a high-performance, scalable web application, these critical factors should be considered:
- Performance: InProc offers better performance as it stores session data in the web server’s memory, providing faster access compared to external storage options.
- Scalability: For web applications in a web farm or load-balanced environment, consider using StateServer or SQLServer to manage session data consistently across multiple servers.
- Reliability: SQLServer mode offers a higher level of data persistence and recovery in the event of server failures compared to StateServer. However, it comes at the cost of increased complexity and potential performance degradation.
- Memory usage: Be cautious with InProc mode for applications with large session data or many concurrent users, as it can consume significant server memory resources, affecting overall performance.
- Data security: When using out-of-process session storage such as StateServer or SQLServer, it’s important to consider data encryption and proper network security to protect sensitive session data.
- Custom requirements: If none of the built-in session state providers meet your specific requirements, consider implementing a custom session state provider for storing session data in a different data store, using tuneable serialization methods, or applying custom caching policies.
How can you implement a custom session state provider in ASP.NET to store session data in a data store other than SQL Server or a State Server?
Answer
To implement a custom session state provider in ASP.NET, follow these steps:
- Create a new class that inherits from the
SessionStateStoreProviderBase
abstract base class. - Override the necessary methods, including:
Initialize()
: Initialize the provider with any required configuration settings.CreateNewStoreData()
: Create an emptySessionStateStoreData
object for a new session.GetItem()
,GetItemExclusive()
: Retrieve session data from the custom data store.SetAndReleaseItemExclusive()
: Save session data to the custom data store and release any locks on the item.ReleaseItemExclusive()
: Release any locks on the item in the custom data store.RemoveItem()
: Remove session data from the custom data store.ResetItemTimeout()
: Update the session timeout value in the custom data store.CreateUninitializedItem()
: Create an uninitialized session data item in the custom data store.
- Implement any additional methods or properties as required by your custom session state provider.
- In the
Web.config
file, configure the custom session state provider by specifying the provider’s fully qualified type name and assembly in the<providers>
element of the<sessionState>
section.
Example configuration:
Loading code snippet...
In this configuration, the mode
attribute is set to "Custom"
and the customProvider
attribute points to the custom session state provider implementation.
Now that we’ve explored various session state providers, let’s delve deeper into the intricacies of ASP.NET’s powerful session management classes.
Understanding the differences in these classes demonstrates not only your technical knowledge but also your ability to leverage the right tools for any situation.
Compare HttpSessionState and HttpSessionStateBase classes in ASP.NET. Why might you choose one over the other?
Answer
The HttpSessionState
and HttpSessionStateBase
classes in ASP.NET have slightly different purposes:
- HttpSessionState: This class is the concrete implementation of session state management in ASP.NET. It provides methods and properties to store and retrieve session data for a specific user. When working with traditional ASP.NET Web Forms applications, the
HttpSessionState
class is typically used for managing session data. - HttpSessionStateBase: This class is an abstract base class introduced with ASP.NET MVC to allow for easier unit testing and extensibility. When working with ASP.NET MVC, you should use
HttpSessionStateBase
instead ofHttpSessionState
for managing session data. In doing so, you can create testable and flexible code by creating mock implementations ofHttpSessionStateBase
for unit testing purposes, without requiring actual session state infrastructure.
In summary, the choice between HttpSessionState
and HttpSessionStateBase
mostly depends on the type of ASP.NET application you are developing:
- Use
HttpSessionState
for Web Forms applications - Use
HttpSessionStateBase
for MVC applications to support testability and extensibility
Explain the potential security risks associated with client-side session management in ASP.NET and what preventive measures can you take?
Answer
Client-side session management involves storing session data on the client (e.g., in cookies, HTML5 Local Storage, or JWT tokens). While this approach can reduce server-side resource requirements, it introduces potential security risks:
- Data tampering: Users might manipulate stored session data on the client-side, potentially modifying application behavior or gaining unauthorized access.
- Data exposure: Sensitive session data might be exposed in case of client-side security breaches, such as cross-site scripting (XSS) attacks or mishandled cookies.
- Data theft: Session data stored within the client’s browser or device can be stolen through techniques like cookie sniffing or MITM (man-in-the-middle) attacks.
To mitigate these risks, consider taking these preventive measures:
- Encryption: Encrypt session data on the server-side before sending it to the client to protect against tampering and unauthorized access.
- Integrity validation: Use digital signatures or other techniques like HMAC (Hash-based Message Authentication Code) to protect session data’s integrity.
- HTTPS: Use HTTPS to protect against MITM attacks and ensure secure data transmission between client and server.
- Secure cookies: Set the “Secure” and “HttpOnly” flags on cookies containing sensitive session data to reduce possible exposure to XSS attacks and other unauthorized access.
- Least privilege: Limit the session data stored on the client-side, especially sensitive data, and follow the principle of least privilege.
What alternatives do you have for maintaining state information for users in web applications other than using ASP.NET session management?
Answer
There are several alternatives for maintaining state information for users in web applications besides using ASP.NET session management:
- Cookies: Use cookies to store small amounts of user-specific data directly on the user’s machine. However, remember that cookies have size limitations, privacy concerns, and potential security risks.
- Query strings: Pass state information through query string parameters in the URLs. This approach is suitable for small amounts of data but can become impractical for complex state management and may also introduce privacy and security concerns.
- Hidden form fields: Store state information in hidden form fields within HTML pages. This method is typically used in combination with other state management techniques and is appropriate for specific scenarios where state data needs to be persisted across form submissions.
- View state: In ASP.NET Web Forms applications, you can use view state to maintain control’s state information across postbacks within a single page. View state stores data in a hidden field on the page and is not suitable for cross-page or multi-user scenarios.
- Application state: The
HttpApplicationState
class can store global, shared state information across all users and sessions of a web application. This approach may serve specific use cases where global data needs to be shared among users, but it should not be used for user-specific or session-specific data. - Database: Store user state information in a persistent storage such as a relational or NoSQL database. This option offers greater flexibility, security, and scalability, but requires additional infrastructure and potentially impacts performance due to database queries and network latencies.
How can you use the Session_OnEnd event in Global.asax to perform custom actions when a user’s session expires in an ASP.NET application?
Answer
The Session_OnEnd
event in Global.asax allows you to perform custom actions when a user’s session expires in an ASP.NET application. This event is triggered once the session timeout is reached.
To use the Session_OnEnd
event, follow these steps:
- Open or create the
Global.asax
file in your ASP.NET application. - Add the
Session_OnEnd
event handler method declaration inside theGlobal.Application
class:
Loading code snippet...
- Inside the
Session_End
method, write your custom logic to perform any desired actions, such as cleaning up resources, logging session expiration information, or updating user statistics in a database.
Note that the Session_OnEnd
event only works with the InProc session state mode. If you’re using StateServer or SQLServer mode, you need to implement a different mechanism to handle session expiration events.
How does the use of readonly and write-locking session behavior in an ASP.NET application help to optimize performance during session management?
Answer
In an ASP.NET application, you can utilize readonly and write-locking session behavior to improve your application’s performance during session management. These approaches minimize contention between concurrent requests trying to access the session state.
- Read-Only Session State: Declare the session state as read-only for pages that don’t need to modify session data. In this mode, multiple concurrent requests for the same session can access the session state data simultaneously without locking or blocking each other. To configure read-only session state, add the
ReadOnly
attribute to the@Page
directive in an ASP.NET page as follows:
Loading code snippet...
- Write-Locking Session State: When a page modifies the session state, it acquires an exclusive lock to prevent other requests from concurrently accessing the session data until the lock is released. This behavior is the default for pages that require full session state access (read and write). When using write-locking session state, be mindful to keep page processing time as short as possible to minimize lock times and potential performance issues.
By leveraging read-only and write-locking session state behavior appropriately, you can prevent unnecessary blocking and improve your ASP.NET application’s performance when managing user sessions.
Great job in exploring session behavior optimization techniques! With this solid foundation, you’re ready to tackle more advanced scenarios.
Up next, we’ll discuss how to handle specific user sessions in a variety of ASP.NET session state provider contexts, demonstrating your adaptability and expertise in creating customized solutions.
How can you force an immediate expiration for a specific user’s session in ASP.NET, considering that there might be an InProc, StateServer, or SQLServer session state provider?
Answer
To force an immediate expiration for a specific user’s session in ASP.NET, regardless of the session state provider (InProc, StateServer, or SQLServer), you can use the Session.Abandon()
method. This method ends the current session, releases the session state lock, and raises the Session_End
event in the Global.asax file (only for InProc mode).
Here’s an example of how to abandon a specific user’s session:
Loading code snippet...
However, it’s essential to note that using Session.Abandon()
does not immediately remove the session data from the session store (such as StateServer or SQLServer). The session data will be removed only when the session state provider’s cleanup mechanism clears the expired sessions, which usually happens periodically based on a configured interval or during the next request with the same session ID.
In the context of session management, how do you ensure data consistency in an ASP.NET web application running on a load-balanced server cluster?
Answer
Ensuring data consistency in an ASP.NET web application running on a load-balanced server cluster involves using a session state management mechanism that can share session data across all instances of the application on different servers. Here are a couple of approaches to achieve consistent session management in load-balanced environments:
- StateServer: Use the StateServer mode to store session data in a separate server process called the “ASP.NET State Service.” This service is accessible by all servers in the load-balanced cluster, allowing the sharing of session state data across multiple instances of the application.
Loading code snippet...
- SQLServer: Use the SQLServer mode to store session data in a central SQL Server database. All servers in the load-balanced cluster can connect to this database to access shared session state data.
Loading code snippet...
In addition to these session state management approaches, consider implementing best practices for data consistency in a load-balanced environment:
- Utilize sticky sessions (session affinity) to make sure users work with the same server instance during their session.
- Ensure session state data serialization and deserialization works correctly across all instances of the application.
- Monitor and configure proper timeout values for both session expiration and connection strings to avoid stale or lost session data.
- Implement retry mechanisms to handle temporary connectivity and database issues.
How can you use the sliding expiration option in the ASP.NET session state configuration to manage user session timeouts more effectively?
Answer
The sliding expiration option in ASP.NET session state configuration allows you to manage user session timeouts more effectively by resetting the session timeout countdown each time the user interacts with the web application, rather than fixing the timeout based on a specific expiration time.
When sliding expiration is enabled, if the user keeps interacting with the application before the session timeout elapses, their session remains active, preventing an undesired session expiration.
To enable sliding expiration, you need to set the cookieless
attribute to "false"
and configure the timeout
attribute value in the <sessionState>
section of your Web.config file.
Loading code snippet...
In this example, the session timeout is set to 20 minutes, and sliding expiration is enabled, as indicated by the cookieless="false"
attribute.
Explain the difference between the HttpApplicationState class and HttpSessionState class in ASP.NET, and discuss when to use each in managing application-level state information.
Answer
The HttpApplicationState
and HttpSessionState
classes in ASP.NET serve different purposes when it comes to managing application-level state information:
- HttpApplicationState: This class is used to store global state information that is accessible to all users and sessions throughout the lifetime of a web application. It acts as a central repository for storing shared data, such as configuration settings, global counters, or cached data. Because the data stored in
HttpApplicationState
is shared among all users, it should be used carefully and only for truly global information. Moreover, it’s essential to handle concurrency and thread-safety when accessing and modifying its data. - HttpSessionState: This class is used to maintain session-specific state information for individual users. Each user has their own instance of
HttpSessionState
, which allows storing and retrieving user-specific data while they interact with the application. The session data is only available to the user during their session and expires or is removed once the session ends.
In summary, use HttpApplicationState
for storing global, shared state information across all users and sessions, and use HttpSessionState
for storing user-specific data during an individual session.
What potential synchronization issues can arise from concurrent AJAX requests made during the same session in an ASP.NET application, and how can they be mitigated?
Answer
Concurrent AJAX requests made during the same session in an ASP.NET application can lead to synchronization issues due to conflicts in accessing and modifying session state data. When multiple asynchronous requests attempt to modify session data at the same time, the session state’s locking mechanism (write-locking session behavior) might result in unintended behavior or blocked requests, ultimately affecting the application’s responsiveness and user experience.
To mitigate potential synchronization issues from concurrent AJAX requests, consider the following approaches:
- Read-Only Session State: If your AJAX requests only need to read session data and do not modify it, make the session state read-only, which allows multiple concurrent requests to access the session data without locking or blocking each other. Configure this in your ASP.NET page by adding the
EnableSessionState="ReadOnly"
attribute to the@Page
directive. - Partition Session Data: Organize your session data into separate partitions or sections relevant to individual AJAX requests to minimize contention and avoid unnecessary locking of unrelated session data.
- Avoid Long-Running Operations: Keep the processing time of your AJAX requests and server-side code as short as possible when accessing session state to reduce the duration of session state locks and improve response times.
- Optimize Session Data Size: Minimize the size of session data stored in the
HttpSessionState
to reduce serialization overhead and improve performance when reading and writing session data, especially for external session state providers like StateServer or SQLServer. - Use Alternative State Management: Consider using alternative state management techniques for storing data relevant to AJAX requests, such as client-side storage (cookies, Local Storage) or server-side persistent storage (database, cache). This approach can help avoid conflicts with session state management while still maintaining the necessary state information for AJAX requests.
By implementing these best practices, you can mitigate synchronization issues arising from concurrent AJAX requests during the same session in an ASP.NET application and enhance overall performance and responsiveness.
Having covered potential issues with AJAX requests and session management, you’ve shown significant prowess in handling modern web application challenges.
As we dive into the next set of questions, we’ll explore the configuration options in the <sessionState>
section of Web.config, highlighting your keen eye for detail and ability to tune your application effectively.
What attributes can you configure in the section in the Web.config file for an ASP.NET application, and explain their significance?
Answer
Several attributes can be configured in the <sessionState>
section of the Web.config file for an ASP.NET application. These attributes control session state behavior and settings:
mode
: Determines the session state storage mode. Options includeInProc
(in-memory within the application),StateServer
(external state service),SQLServer
(database), orCustom
(custom session state provider). Default isInProc
.stateConnectionString
: Specifies the connection string for the external state service when usingStateServer
mode.sqlConnectionString
: Specifies the connection string for the SQL Server database when usingSQLServer
mode.cookieless
: Specifies whether to use a cookie-based session ID or encode the session ID in the URL. Options includetrue
,false
,UseCookies
,UseUri
,AutoDetect
, orUseDeviceProfile
. Default isUseCookies
.timeout
: Specifies the session timeout in minutes. Default is 20 minutes.allowCustomSqlDatabase
: When usingSQLServer
mode, setting this totrue
enables a custom database name for session state storage instead of the defaulttempdb
database. Default isfalse
.partitionResolverType
: Specifies the type of a custom partitioning resolver class for partitioning session state data in a web farm setup with multiple back-end databases or state servers.customProvider
: Specifies the name of a custom session state provider when usingCustom
mode.regenerateExpiredSessionId
: Specifies whether to generate a new session ID when the expired session ID is requested. Default isfalse
.useHostingIdentity
: When using an external state service or database, setting this totrue
applies the ASP.NET application’s process identity for authorization check. Default isfalse
.
Here’s an example of a <sessionState>
section with some attributes configured:
Loading code snippet...
In this example, the session state is configured to use the StateServer mode and specifies the stateConnectionString, cookieless, and timeout attributes.
How can you optimize the performance of an ASP.NET application when using StateServer or SQLServer session state modes?
Answer
To optimize the performance of an ASP.NET application when using StateServer or SQLServer session state modes, consider the following best practices:
- Minimize Session Data Size: Storing a large amount of data in session state can impact the performance due to increased serialization and network overhead. Keep your session data as small as possible.
- Use Data Compression: If your session data is large, consider using data compression techniques to compress the data before storing it in the session.
- Optimize Serialization: Use efficient serialization and deserialization libraries to reduce the overhead of storing and retrieving session data in external state providers.
- Concurrency Control: Limit the concurrent access to session data by designing your application with proper data partitioning or caching strategies. This will prevent excessive locking or blocking of session data.
- Optimize Connection Strings: When using SQLServer mode, ensure that your connection strings are optimized for performance by specifying appropriate timeout values, pooling settings, and other performance-related options.
- Database Indexing and Partitioning: When using SQLServer mode, optimize your session state database by applying proper indexing and partitioning strategies.
- Monitor and Tune State Service or Database: Monitor the performance of the State Server service or SQL Server database and apply necessary tuning to ensure optimal performance.
- Use Load Balancing with Session Affinity: If your application runs in a web farm environment, use load balancing with session affinity (sticky sessions), ensuring that users are routed to the same server instance during their session.
- Choose Appropriate Timeout Values: Set appropriate session timeout values to balance the user experience and resource use. Consider using a sliding expiration approach for extending session lifetime with user activity.
By implementing these best practices, you can optimize the performance of your ASP.NET application when using StateServer or SQLServer session state modes and enhance the overall user experience.
What is the impact of session state management on server and network resources, and how can ASP.NET developers minimize its negative effects on performance?
Answer
Session state management can have a significant impact on server and network resources, potentially affecting the performance and responsiveness of your ASP.NET application. Some of the effects of session state management include:
- Increased memory usage: Storing session state data, particularly in the
InProc
mode, requires additional memory resources on the web application server. Large session data structures can quickly consume server memory, leading to performance issues. - Increased CPU usage: Session state management activities like synchronization, locking, serialization, and deserialization can consume CPU resources, affecting the server’s overall processing capacity.
- Network overhead: When using external session state providers like
StateServer
orSQLServer
, session data needs to be transmitted between the web server and the external server, adding network overhead.
To minimize the negative effects of session state management on server and network resources, ASP.NET developers can:
- Limit the amount of data stored in session state, keeping it lightweight.
- Optimize session data structures for efficient serialization and deserialization.
- Use compression techniques to reduce the size of session data in high-latency environments.
- Partition session data to minimize contention and improve concurrency control.
- Choose the most appropriate session state mode based on your application’s requirements, scalability, and available resources.
- Implement data caching strategies to offload some session data management tasks from session state, such as caching frequently accessed data instead of storing it in session state.
- Set appropriate session timeout and sliding expiration values to balance user experience and resource consumption.
- Monitor and fine-tune the performance of the session state provider, whether it’s the ASP.NET State Service or a SQL Server database.
- Consider alternative state management techniques like client-side storage (cookies, Local Storage) or server-side persistent storage (database, cache) for specific use cases to reduce the session state management overhead.
By applying these best practices, ASP.NET developers can minimize the impact of session state management on server and network resources while maintaining the necessary state information for their web applications.
Explain how to use the HttpSessionStateWrapper class to create testable code while working with ASP.NET session management.
Answer
The HttpSessionStateWrapper
class is an adapter class that provides a way to wrap the HttpSessionState
object, enabling testable code when working with ASP.NET session management. The HttpSessionStateWrapper
class is part of the System.Web.Abstractions
namespace, and it implements the HttpSessionStateBase
abstract class.
By using the HttpSessionStateWrapper
class, developers can create unit tests for their code by mocking or faking the HttpSessionStateBase
class and injecting it into the application code.
To use HttpSessionStateWrapper
for testable code, follow these steps:
- Replace direct references: Replace the direct references to
HttpSessionState
in your code with references to theHttpSessionStateBase
abstract class or an interface that derives from it. - Wrap the object: Create an instance of
HttpSessionStateWrapper
, passing the currentHttpSessionState
object in the constructor:
Loading code snippet...
- Use the wrapped object: Utilize the wrapped
HttpSessionStateWrapper
object throughout your application code, interacting with it as if it were the originalHttpSessionState
object:
Loading code snippet...
- Create testable code: In your unit tests, create mock or fake objects that derive from
HttpSessionStateBase
or the custom interface that you created, and inject them into your application code for testing purposes.
By using the HttpSessionStateWrapper
class, you can create testable, more maintainable code by decoupling the session management functionality from your application’s core logic, allowing for better unit testing and separation of concerns.
How can you implement a real-time session timeout warning using both server-side (ASP.NET) and client-side (JavaScript) technologies, while appropriately managing the user’s session state?
Answer
To implement a real-time session timeout warning using both server-side (ASP.NET) and client-side (JavaScript) technologies, follow these steps:
- Set the session timeout value: Configure the session timeout value in your ASP.NET application’s Web.config file:
Loading code snippet...
In this example, the session timeout is set to 20 minutes.
- Create a JavaScript function: Create a JavaScript function to display a session timeout warning when the timeout is close to expiring:
Loading code snippet...
- Set a client-side timer: Implement a client-side timer using JavaScript’s
setTimeout()
function to trigger the warning function when the session is close to expiring:
Loading code snippet...
- Extend the session with server activity: If the user takes action to keep the session alive (e.g., clicking a button or interacting with your warning message), make an AJAX request to the server to extend the session. On the server side, update any necessary session data or access the session to extend the sliding expiration.
By implementing this approach, you can provide real-time session timeout warnings to users and manage the user’s session state effectively using both server-side (ASP.NET) and client-side (JavaScript) technologies.
Congratulations on making it through this comprehensive list of session management in ASP.NET interview questions and answers! By mastering these concepts, you’ve proven your expertise in one of the most critical aspects of web application development.
As a developer who can showcase a depth of knowledge and problem-solving proficiency, you’re well on your way to acing your next interview and making a significant impact in the world of web development. Keep honing your skills and exploring the ever-changing ASP.NET ecosystem, as your ongoing pursuit of mastery will drive your success in this fast-paced and competitive field.
Good luck, and may your next interview be the launching point of an incredible new chapter in your career journey!