Access Modifiers in C# (Full Guide)
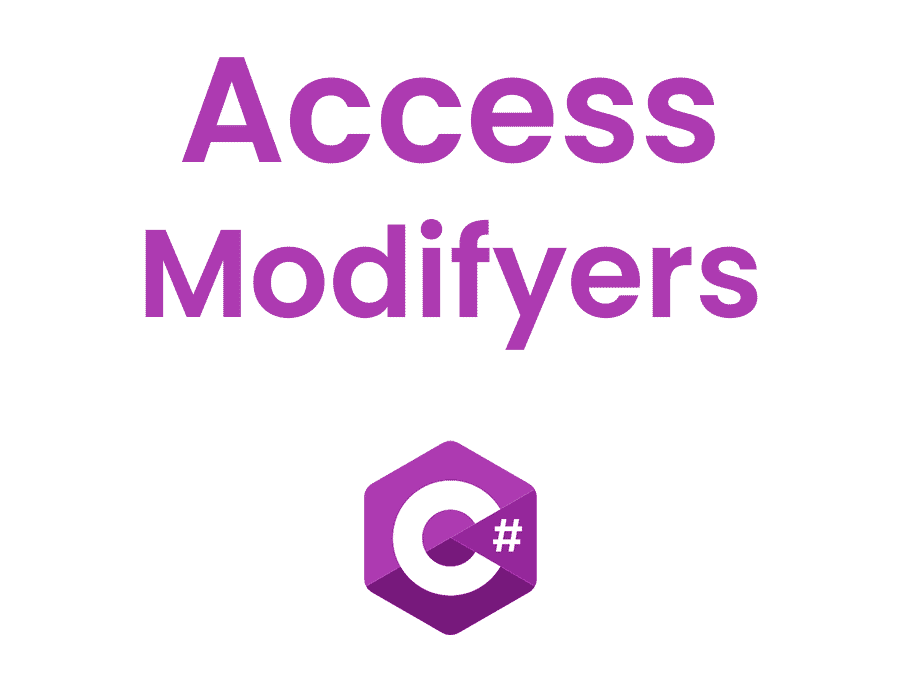
Do you wonder how top-notch C# developers control access to their class members? Buckle up because it’s time to unravel the secret: C# Access Modifiers! Not only will you learn everything about access modifiers in C#, but you’ll also level up your C# knowledge, becoming a true programming guru! So, let’s dive right into it!
Introduction to Access Modifiers in C#
What is Access Modifiers in C#?
Access modifiers in C#, sometimes referred to as c# accessibility modifiers, are keywords added to class members that dictate their visibility, essentially determining who can access them. These handy little magic words help us maintain encapsulation and prevent unintentional modification, thus keeping our code clean and secure.
Importance of Access Modifiers in C#
Using access modifiers strategically is critical in C# for the following reasons:
- It helps you to adhere to the principles of encapsulation, ensuring a better-structured code.
- It prevents unintentional changes to the internal state of an object.
- It maintains a separation of concerns between different layers of an application.
So, are you ready to harness the power of C# access modifiers? Let’s explore the different types now!
Types of C# Access Modifiers
C# offers five access modifiers, each with their unique functionality. Here, we’ll take a sneak peek into each modifier before diving into the details!
Public Access Modifier
The most permissive of all the c# access modifiers, the public
keyword unveils your class members to the world, letting everyone access them. Talk about being sociable!
Private Access Modifier
This might be called the “introvert” of the C# access modifier bunch. When you use private
, you’re hogging that class member all to yourself, restricting access only to the class where it’s defined.
Protected Access Modifier
Ah, the protected
modifier – it’s like your family in the programming world. Using this keyword, members are accessible only within the class itself and any derived subclasses. Sharing is caring, after all!
Internal Access Modifier
When you want to let everyone in the same assembly play with your class members, internal
is the way to go. This modifier is perfect for allowing access within the assembly while keeping it hidden from the outside world.
Protected Internal Access Modifier
Remember how I said protected
is like your family? Well, this one’s your family and close friends! protected internal
combines the best of both worlds: members can be accessed within the same assembly or from derived subclasses in any other assembly.
Excited much? It’s finally time to dive deeper into these modifiers and witness their magic in action!
C# Accessibility Modifiers: Explained with Examples
Using Public Access Modifiers in C#
Understanding Public Members in C# Classes
When you declare a member as public
, it can be accessed from anywhere: within the class, derived classes, and even other assemblies. It’s the most open of all the c# class modifiers.
Public Modifier Example: C# Class Modifiers
Loading code snippet...
Are you feeling the openness of the public
modifier? Great! Let’s move on to the next one – it’s time to get up close and personal!
Using Private Access Modifiers in C#
Understanding Private Members in C# Classes
When you use the private
keyword, you’re restricting access to the member only within the class it’s defined. It’s the most restrictive of all accessibility modifiers.
Private Modifier Example: C# Class Modifiers
Loading code snippet...
See how we kept our age
property hush-hush with the private
modifier? Private indeed! Next up, the protected
modifier prepares for some family time!
Using Protected Access Modifiers in C#
Understanding Protected Members in C# Classes
The protected
access modifier allows member access only within the declaring class and any derived subclasses. Now you’re sharing, but only with your extended programming family!
Protected Modifier Example: Protected Private Public C#
Loading code snippet...
Phew! Just as we thought, the protected
modifier lets our Dog
class inherit the sound
property. Now, onto the internal
modifier and let’s keep our class members within assembly bounds!
Using Internal Access Modifiers in C#
Understanding Internal Members in C# Classes
When you designate a member with the internal
access modifier, only code within the same assembly can access it. It’s like an exclusive club for members of the same assembly!
Internal Modifier Example: C# Internal Access Modifier
Loading code snippet...
As you can see, the internal
access modifier safeguards the member assemblyText
, keeping it assembly-bound.
Using Protected Internal Access Modifiers in C#
Understanding Protected Internal Members in C# Classes
With the protected internal
access modifier, members can be accessed within the same assembly or derived classes from any other assembly. It’s like joining an exclusive assembly club and inviting the extended family!
Protected Internal Modifier Example: C# Class Modifiers
Loading code snippet...
There you have it – the protected internal
modifier allows our DerivedClass
to access the assemblyText
member from another assembly.
Default Access Modifier in C#: What You Need to Know
Discovering the Default Access Modifier
In case you haven’t specified an access modifier for a class member, C# automatically assigns the private
modifier for nested and non-nested classes, while internal
is the default for top-level classes. The more you know!
Why is it Important to Understand the Default Access Modifier?
Being aware of default access modifiers is crucial for the following reasons:
- Ensures the intended access level for class members, preventing inadvertent access.
- Protects your code from modification by other developers not familiar with your intent.
- Boosts code readability by clarifying access levels.
Now that we’re in-the-know with default access modifiers, let’s switch gears and discuss the protection levels in C#!
C# Protection Levels: An Overview
Understanding Protection Levels in C#
Protection levels in C# indicate which parts of your code can access individual class members. The levels from most to least restrictive are:
- Private
- Protected
- Internal
- Protected internal
- Public
Protected Private Public C#: A Comparison
- Private: most restrictive, members accessible only within the declaring class.
- Protected: members accessible within the declaring class and any derived subclasses.
- Public: least restrictive, members accessible from any class in any assembly.
.NET Access Modifiers: A Practical Approach
How .NET Access Modifiers are Interrelated with C# Modifiers
C# access modifiers are part of the .NET Framework, which means they also apply to other .NET languages such as Visual Basic, allowing for seamless interoperability. Consequently, you can maintain consistency in access management across different .NET languages. This ensures that you can create libraries or components that are easily portable and can be consumed by other applications regardless of the .NET language in use.
For example, consider a C# library project that exposes a public class. Because the access modifiers are consistent across .NET languages, you can easily consume this class from a Visual Basic or F# project, with the access controls still being enforced by the .NET runtime.
Impact of Access Modifiers on your .NET Application
Proper usage of .NET access modifiers impacts your .NET application by:
Encouraging modular, organized code
Naturally, when you use access modifiers effectively, your code will be more modular and organized. By encapsulating implementation details using private
and protected
modifiers, you encourage the creation of robust interfaces that enable you to change the internal workings of a class without negatively affecting other parts of your application.
Ensuring security through encapsulation
Careful use of access modifiers provides a layer of security by preventing unauthorized access to sensitive class members. By enclosing data within classes and providing access only where necessary, you create a barrier between your codebase and potential security risks.
Facilitating robust code, reducing the chance of bugs
By limiting access to tightly controlled areas, you minimize the places where errors could be introduced. As a result, you’ll have a much more stable and reliable codebase that is less likely to generate unexpected bugs.
For example, by applying the internal
access modifier, you can ensure that the critical internal components of your assembly are not accessible from other assemblies, reducing the chance of accidental modification.
Accessibility Modifier: Best Practices
To make the most out of access modifiers, follow these golden rules:
Favor the principle of least privilege
Granting members only the access they require ensures that you’re adhering to the least privilege principle. The members of a class should only have the minimum necessary access to its properties and methods. This results in better-structured code and reduces potential security vulnerabilities.
For example, instead of exposing an entire collection, consider exposing methods to add or remove items, allowing you to enforce validation and maintain control:
Loading code snippet...
Use
Whenever possible, use private
for implementation details that should be restricted to a specific class. Use protected
when you need to make details available in derived classes. By keeping implementation details hidden from other parts of the application, you maintain a separation of concerns and help preserve a clean, organized structure.
For example, consider an e-commerce class where you prefer not to expose the raw list of products:
Loading code snippet...
Use
There are cases where you might have helpers or utility classes that are specific to an assembly and should not be exposed outside of it. In such situations, use the internal
access modifier to ensure that these implementation details stay within the assembly.
Loading code snippet...
Keep
Reserve the public
access modifier for exposing stable APIs that will not change frequently. Publicly accessible members have a broader surface area for interaction, which increases the risk of unintentional modification and bugs. As much as possible, limit public
usage to interfaces or APIs that you expect to be consumed by other developers.
When to use CS Modifier: A Decision-Making Guide
Seeking guidance on which C# access modifier to use? Consider these pointers:
Private: when members are exclusive to their class
Use the private
access modifier when class members should be restricted solely to their containing class. This ensures implementation details remain hidden, promoting encapsulation and cleaner code.
Loading code snippet...
Protected: if members are shared only among derived classes
Opt for the protected
modifier when class members should be accessible to derived classes. This enables class inheritance and polymorphism while still maintaining a level of encapsulation.
Loading code snippet...
Internal: to keep members visible solely within the same assembly
Choose the internal
access modifier to restrict class members’ visibility to within the same assembly. This is useful when creating frameworks or libraries with APIs that should be kept internal.
Loading code snippet...
Protected internal: when members belong to derived classes and the same assembly
Use the protected internal
modifier when class members must be accessible both to derived classes in other assemblies and classes within the same assembly. This provides greater flexibility while still constraining access.
Loading code snippet...
Public: for members intended for use throughout the entire application
Finally, utilize the public
access modifier for widely accessible class members that other developers will use. This should be reserved for stable interfaces and APIs that are less likely to be modified in the future.
Loading code snippet...
Conclusion
Advantages of using Access Modifiers in C# Coded Applications
Congratulations! You’re now a bona fide C# access modifiers expert! By implementing these modifiers, you’re ensuring encapsulation, modularity, robustness, and security in your C# code, propelling you to programming greatness! The programming world has never seen such powerful and controlled code management!
Empower Your C# Development Skills with Access Modifiers Knowledge
What are you waiting for? Start implementing access modifiers in your projects to create aura-inspiring C# code that’ll leave other developers marveling at your skills! Remember, with great power comes great responsibility, so use your newfound knowledge wisely and conquer the programming world! Good luck, and happy coding!