Understanding Transactions in Entity Framework Core
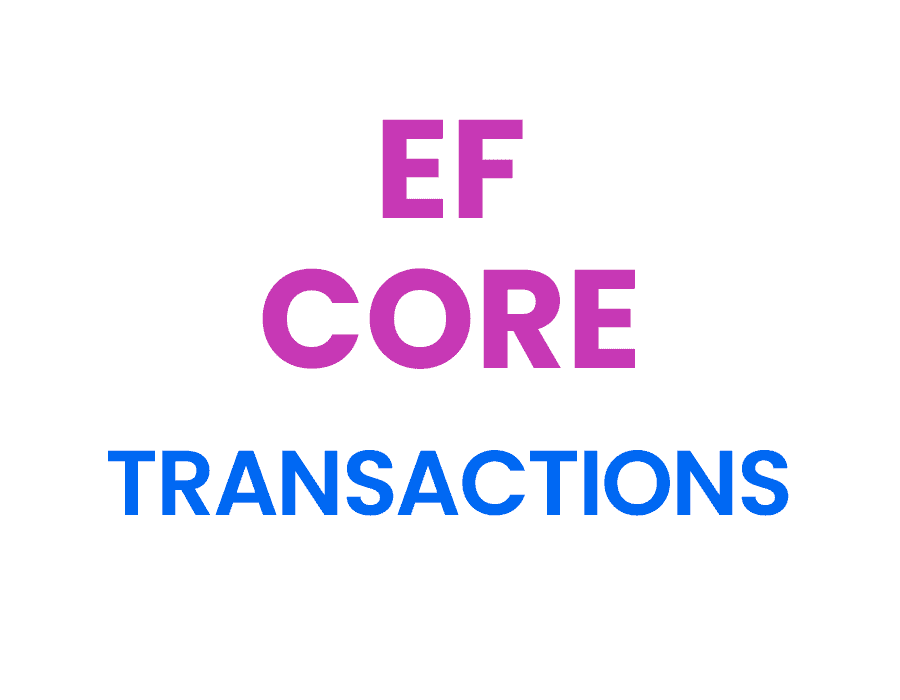
Entity Framework Core (EF Core) is a powerful Object-Relational Mapper (ORM) that simplifies data access by abstracting the complexities of relational databases. One of the most important aspects of working with databases is managing transactions.
This article covers the fundamentals of transactions in Entity Framework Core, how to work with them, different strategies to implement, handling errors and rollbacks, transaction isolation levels, and performance considerations. By the end of this article, you will have a strong understanding of how to effectively manage transactions in EF Core.
Introduction to Transactions
What are Transactions?
A transaction is a sequence of one or more database operations (such as insert, update, delete or select) that are executed as a single unit of work. Transactions allow multiple users to work on a database concurrently without corrupting the data or causing inconsistency.
They maintain the ACID properties (Atomicity, Consistency, Isolation, and Durability), ensuring that the database remains in a consistent state even in the event of errors or system failures.
Atomicity guarantees that either all operations in a transaction are executed, or none are. Consistency ensures that the database transitions from one consistent state to another. Isolation ensures that the execution of individual transactions is isolated from other ongoing transactions. Durability guarantees that once a transaction is committed, its effects persist in the database even in the case of system failures.
For example, imagine a banking application where a user transfers money from one account to another. This transaction involves two operations: withdrawing money from one account and depositing it into another. If one of these operations fails, the entire transaction should be rolled back to maintain the consistency of the data.
Why are Transactions Important?
Transactions are crucial for maintaining data integrity and consistency in a multi-user environment. They prevent data corruption and undesired side effects by ensuring changes made by one transaction are not visible to others until the transaction is completed. Additionally, transaction management plays an essential role in handling errors and rollback scenarios, allowing applications to recover gracefully from failures or unexpected events.
Imagine a scenario where two users are trying to update the same record simultaneously. Without transactions, it’s possible for one user’s changes to overwrite the other user’s changes, leading to data corruption and inconsistency. Transactions prevent this by ensuring that each user’s changes are isolated from one another until they are completed.
Understanding how to manage transactions effectively in EF Core is essential for developers to build robust, scalable, and maintainable applications that interact with relational databases. By using transactions, developers can ensure that their applications maintain data integrity and consistency, even in the face of errors or unexpected events.
Overall, transactions are a critical component of any database-driven application, and understanding how they work is essential for building reliable and robust software.
Entity Framework Core Overview
Entity Framework Core is a powerful Object-Relational Mapping (ORM) framework for .NET that provides a simple way to work with databases. It allows developers to work with databases using .NET objects, eliminating the need to write complex SQL queries. EF Core supports a wide range of database providers, including Microsoft SQL Server, MySQL, PostgreSQL, and SQLite.
Key Features of Entity Framework Core
Some of the key features of Entity Framework Core include:
- LINQ support: EF Core allows you to write type-safe queries using LINQ, a powerful query language for .NET.
- Change tracking: EF Core automatically tracks changes made to entities in your application, simplifying data updates.
- Code-first development: EF Core enables developers to define their data model using C# classes, automatically generating the database schema.
- Lazy loading: With EF Core, you can easily specify which related data should be automatically loaded as needed.
- Built-in conventions: EF Core ships with sensible default conventions that facilitate a smooth development experience.
These features make Entity Framework Core a popular choice for building .NET applications that require database connectivity.
How Entity Framework Core Handles Transactions
By default, Entity Framework Core handles transactions for you, beginning a new transaction whenever you call the SaveChanges or SaveChangesAsync methods on your DbContext instance. It automatically commits the transaction if all operations succeed, or rolls back if any operation fails, ensuring data integrity and consistency.
However, EF Core also provides advanced transaction management capabilities, allowing you to work with transaction scopes, explicit transactions, and control transaction isolation levels according to your application’s requirements. This gives developers greater control over how transactions are managed in their applications.
Overall, Entity Framework Core is a powerful and flexible ORM framework that makes it easy to work with databases in .NET applications. Its rich feature set and advanced transaction management capabilities make it a popular choice for building robust and scalable applications.
Working with Transactions in Entity Framework Core
When working with Entity Framework Core, transactions are an essential part of ensuring data integrity and consistency. Transactions allow you to group a set of database operations into a single unit of work, ensuring that all operations either succeed or fail together.
Transaction Scopes
EF Core supports working with TransactionScope, a .NET class that allows you to define a block of code that participates in a transaction. TransactionScope simplifies transaction management by automatically handling the commit and rollback logic for you, depending on the success of the enclosed operations.
To use TransactionScope, wrap your database operations in a using block, and instantiate a new TransactionScope object. If the operations within the block are successful, the transaction scope will commit the transaction when it is disposed. Otherwise, the transaction is implicitly rolled back on any error, preserving data integrity.
TransactionScope also supports distributed transactions, allowing you to participate in transactions that span multiple databases or even different systems.
Explicit Transactions
EF Core also allows you to manage transactions explicitly through the DbContext.Database.BeginTransaction, DbContext.Database.CommitTransaction, and DbContext.Database.RollbackTransaction methods. Explicit transactions provide granular control over your transactions, allowing you to determine when to start, commit, or roll back transactions according to your needs.
While explicit transactions offer more control, they require careful management to avoid data corruption or undesired side effects due to incorrect transaction handling. It is essential to ensure that all operations within a transaction are consistent and that the transaction is committed or rolled back appropriately.
SaveChanges and SaveChangesAsync
In EF Core, both SaveChanges and SaveChangesAsync methods perform automatic transaction management. Whenever you call these methods, a new transaction is started, including all the operations executed in the DbContext instance. If the operations are successful, the transaction is committed, otherwise, it is rolled back.
The primary difference between SaveChanges and SaveChangesAsync is that SaveChangesAsync is an asynchronous version of SaveChanges, providing better performance and scalability in concurrent scenarios. However, it is essential to ensure that all operations are consistent and that the transaction is committed or rolled back appropriately, even with automatic transaction management.
In conclusion, transactions are an essential part of working with Entity Framework Core, ensuring data integrity and consistency. Whether you choose to use TransactionScope or manage transactions explicitly, it is essential to ensure that all operations within a transaction are consistent and that the transaction is committed or rolled back appropriately.
Implementing Transaction Strategies
When working with databases, it’s essential to consider how transactions are handled to ensure data consistency and avoid conflicts. In Entity Framework Core (EF Core), you can implement two main concurrency strategies: optimistic and pessimistic concurrency.
Optimistic Concurrency
Optimistic concurrency is a strategy where multiple transactions can access and modify the same data simultaneously without acquiring locks. It assumes that conflicts are rare, and only checks for conflicts when trying to commit changes. If a conflict is detected (i.e., another transaction has modified the same data), EF Core automatically aborts the transaction, allowing you to handle the conflict and retry the operation.
EF Core enables optimistic concurrency by default and uses a built-in mechanism called “concurrency tokens” to detect data conflicts. Concurrency tokens are special properties in your entity classes that EF Core uses to track changes. When you update an entity, EF Core compares the concurrency token value in the database with the one in memory. If they match, the update is allowed to proceed. If they don’t match, EF Core assumes that another transaction has modified the data and aborts the operation.
Optimistic concurrency is a good choice for most scenarios, as it provides better performance by avoiding lock contention. However, it may not be ideal for systems with frequent conflicts, as it can lead to a high number of retries and increased complexity in conflict resolution.
Pessimistic Concurrency
Pessimistic concurrency, on the other hand, is a strategy where a transaction acquires locks on the data it accesses, preventing other transactions from modifying the locked data until the lock is released. While this approach guarantees data consistency, it can lead to performance issues and deadlocks, particularly in high-concurrency scenarios.
EF Core does not support pessimistic concurrency out of the box, but you can implement it using custom SQL queries and database-specific locking mechanisms. For example, you can use the SELECT ... FOR UPDATE
statement in PostgreSQL to lock rows for update, or the sp_getapplock
stored procedure in SQL Server to acquire application locks.
When implementing pessimistic concurrency, it’s essential to consider the potential performance pitfalls and increased complexity that comes with lock management. You should also be aware that pessimistic concurrency can lead to reduced concurrency and increased contention, as transactions may need to wait for locks to be released.
Choosing the Right Strategy
When deciding which concurrency strategy to implement in your EF Core application, consider the characteristics of your specific use case, balancing performance and data consistency requirements. Optimistic concurrency is preferable in most situations, as it provides better performance by avoiding lock contention, but may not be ideal for scenarios with frequent conflicts.
Pessimistic concurrency can be more appropriate for systems where data consistency is of paramount importance, and conflicts are common. However, beware of the potential performance pitfalls and increased complexity that comes with lock management.
Handling Transaction Errors and Rollbacks
Transactions are a crucial aspect of database management, ensuring that data changes are atomic and consistent. However, when working with transactions, you might encounter various errors that can cause data integrity issues. It’s essential to handle these errors correctly to maintain data consistency and avoid potential data corruption.
Common Transaction Errors
When working with transactions in EF Core, you might encounter various errors, such as:
- Concurrency conflicts: This error occurs when multiple transactions attempt to modify the same data simultaneously, causing a violation of data integrity.
- Deadlocks: In cases where multiple transactions lock resources in a circular manner, a deadlock can occur, causing all involved transactions to be blocked indefinitely.
- Timeout errors: Long-running transactions may exceed the configured timeout period, resulting in an error and potential data corruption.
- Foreign key constraint violations: Errors can arise when inserting or deleting data that violates foreign key constraints, causing transactional conflicts.
It’s crucial to handle these errors appropriately to maintain data consistency and avoid potential data corruption.
Implementing Rollbacks
When an error occurs during a transaction, it’s essential to roll back the transaction to maintain data integrity and consistency. In EF Core, rollbacks are handled differently depending on the transaction mechanism being used:
- Automatic transaction management: In the case of SaveChanges and SaveChangesAsync, EF Core handles rollbacks transparently for you by automatically rolling back the transaction when an error occurs.
- TransactionScope: With TransactionScope, the rollback is implicitly performed when an error is thrown within the using block.
- Explicit transactions: When using explicit transactions, you need to call the DbContext.Database.RollbackTransaction method to perform a rollback when an error occurs.
It’s crucial to understand the transaction mechanism being used and handle rollbacks appropriately to maintain data consistency and avoid potential data corruption.
Best Practices for Error Handling
Implementing proper error handling when working with transactions is critical to ensure data consistency and robustness. Some best practices to consider include:
- Always catch exceptions and handle them appropriately, ensuring a rollback is performed when necessary. This will help maintain data consistency and avoid potential data corruption.
- Use appropriate retry logic for transient errors, such as connection timeouts or deadlocks, giving your application a chance to recover. This can help ensure that your application is robust and can handle unexpected errors.
- Keep transactions as short as possible to minimize the likelihood of conflicts, deadlocks or timeouts. This can help reduce the risk of data integrity issues and improve application performance.
- Design your application to handle potential conflicts, using an appropriate concurrency strategy. This can help ensure that your application can handle concurrent data modifications and maintain data consistency.
By following these best practices, you can help ensure that your application is robust, reliable, and can handle unexpected errors when working with transactions.
Transaction Isolation Levels in Entity Framework Core
Understanding Isolation Levels
Transaction isolation levels dictate how much one transaction is isolated from the effects of other concurrent transactions. There are five commonly-used isolation levels:
- Read Uncommitted: The lowest level of isolation, allows transactions to read uncommitted data from other transactions. This can lead to dirty reads and other issues.
- Read Committed: Transactions can only read data that has been committed by other transactions. This level avoids dirty reads but can still experience other isolation problems.
- Repeatable Read: Transactions can only read data that was committed before their execution, and all reads are repeatable. This prevents dirty reads and non-repeatable reads, but may still experience phantom reads.
- Serializable: The highest level of isolation, ensuring that transactions are completely isolated from one another. This can lead to increased lock contention, potentially hurting performance.
- Snapshot: This isolation level uses row versioning to avoid locks, providing consistency without impeding concurrency.
Choosing an appropriate isolation level is a trade-off between data consistency and performance. Higher levels of isolation generally provide better data consistency guarantees but can introduce performance overhead due to increased lock contention.
For example, if your application requires high data consistency guarantees, you may want to consider using the Serializable isolation level. However, if your application requires high concurrency and performance, you may want to consider using the Snapshot isolation level.
Configuring Isolation Levels
In EF Core, you can configure the transaction isolation level when using explicit transactions or TransactionScope. To configure the isolation level with explicit transactions, use the DbContext.Database.BeginTransaction method, providing the desired isolation level as an argument. For instances using TransactionScope, provide the desired isolation level when instantiating a new TransactionScope object.
For example, to configure an explicit transaction using the Repeatable Read isolation level:
Loading code snippet...
It is crucial to choose the appropriate isolation level to meet your application’s consistency and performance requirements, as the default isolation level may not be suitable for all cases.
Conclusion
Transaction isolation levels are an important consideration when developing applications with Entity Framework Core. By understanding the different isolation levels and their trade-offs, you can choose the appropriate level to meet your application’s consistency and performance requirements. Additionally, by configuring the isolation level when using explicit transactions or TransactionScope, you can ensure that your application is using the appropriate level of isolation for each transaction.
Performance Considerations
Performance is a critical aspect of any application, and optimizing transaction performance is essential for ensuring that your application runs smoothly and efficiently. When working with transactions in EF Core, there are several guidelines you should follow to optimize performance.
Optimizing Transaction Performance
Consider the following guidelines to optimize transaction performance:
- Select the right concurrency strategy: Optimistic concurrency generally provides better performance by reducing lock contention, but may not be suitable for all use cases. Pessimistic concurrency may be a better choice if your application requires stronger consistency guarantees.
- Use appropriate isolation levels: Higher isolation levels increase data consistency guarantees but can introduce performance overhead. Choose a suitable isolation level based on your application’s requirements. For example, if your application requires strong consistency guarantees, you may need to use a higher isolation level such as Serializable.
- Keep transactions short: Shorter transactions are less likely to cause deadlocks, timeouts, or conflicts. Break long transactions into smaller units of work when possible. This can also help improve concurrency by reducing the amount of time that locks are held.
- Reduce the number of round-trips to the database: Minimizing round-trips to the database can help mitigate performance issues related to transaction management. Consider using batch updates or stored procedures to update multiple records in a single round-trip.
Monitoring and Diagnosing Performance Issues
Detecting performance bottlenecks and issues related to transaction management is an essential part of optimizing your EF Core application. Some approaches to diagnosing and monitoring transaction performance include:
- Logging and monitoring: Use EF Core’s built-in logging and diagnostic features to gain insights into the performance of your transactions and identify potential issues. This can include logging SQL queries, transaction durations, and concurrency conflicts.
- Profiling: Use database profiling tools to analyze query performance and identify slow or problematic transactions. This can help you identify areas of your application that may require optimization.
- Testing and load testing: Conduct thorough testing and load testing to identify performance bottlenecks and errors under various workloads. This can help you identify issues that may only occur under heavy load.
- Metrics and monitoring: Collect and analyze application and database metrics to detect performance issues and ensure optimal transaction management. This can include monitoring CPU usage, memory usage, and disk I/O.
By following these guidelines and monitoring the performance of your transactions, you can ensure that your EF Core application is running efficiently and providing a great user experience.
Conclusion
Key Takeaways
In summary, understanding transactions in Entity Framework Core is essential for building robust, scalable, and maintainable applications that interact with relational databases. Key takeaways from this article include:
- Transactions ensure data integrity and consistency in multi-user environments through the ACID properties.
- EF Core provides various mechanisms to manage transactions, such as automatic transaction management, transaction scopes, and explicit transactions.
- Concurrency strategies, such as optimistic and pessimistic concurrency, play a vital role in managing data conflicts and ensuring consistency.
- Error handling and rollback scenarios are crucial for maintaining data consistency in transactions.
- Transaction isolation levels impact data consistency and performance, and should be chosen according to your application’s requirements.
Further Resources
To deepen your understanding of transactions in Entity Framework Core and improve your skills in this area, explore these additional resources:
- Microsoft Docs: Entity Framework Core Transaction Management
- Pluralsight Course: Getting Started with Entity Framework Core 2
- Book: Pro Entity Framework Core 6: An Expert Guide for Developers
- GitHub Repository: Entity Framework Core Source Code and Samples