How to Use TextWriter in C# for Writing Text Files
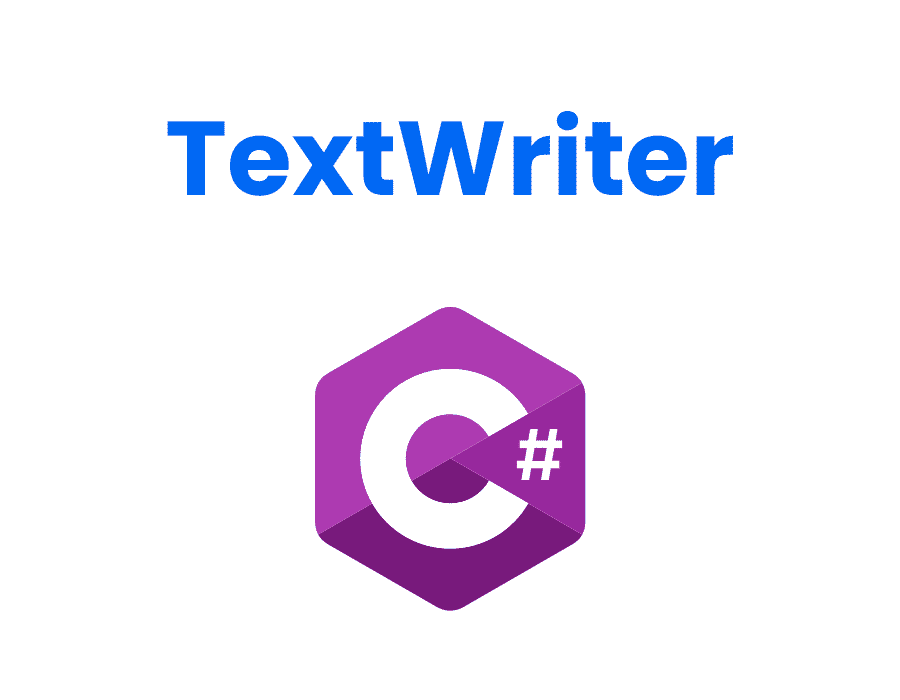
When working in C#, one of the fundamental activities is writing to and reading from text files. TextWriter provides an efficient and flexible way to write text data to a file. In this article, we will explore TextWriter and see how to use it effectively.
Introduction to TextWriter and its Functions
TextWriter is an abstract class in C# that provides methods for writing to text data to a stream. It serves as the base class for StreamWriter and StringWriter, which provide concrete implementations for writing to files and strings, respectively. The primary function of TextWriter is to write text data using an encoding that converts characters into a sequence of bytes. This conversion helps ensure that the written data can be decoded accurately when read by other programs.
One of the key advantages of using TextWriter is that it allows for efficient writing of large amounts of text data. This is because it buffers the data in memory before writing it to the stream, which reduces the number of write operations required. Additionally, TextWriter provides methods for formatting text data, such as WriteLine and Write, which allow for easy insertion of line breaks and other formatting options. Overall, TextWriter is a powerful tool for working with text data in C# and is widely used in a variety of applications.
Understanding the Difference between StreamWriter and TextWriter
StreamWriter is a concrete implementation of TextWriter that is specifically designed to write text data to a file. It inherits from TextWriter and provides additional functionality, like automatic flushing of the buffer, which makes it easy to write to files efficiently.While TextWriter provides a base set of methods for writing data, StreamWriter provides advanced capabilities like the ability to append to an existing file or overwrite the contents of a file.
Another key difference between StreamWriter and TextWriter is that StreamWriter is a class that is used for writing characters to a stream, while TextWriter is an abstract class that provides a set of methods for writing text to a stream. This means that StreamWriter is more specialized and provides more specific functionality for writing text to a file.
It is also important to note that StreamWriter and TextWriter are both part of the System.IO namespace in .NET. This namespace provides a set of classes for working with files and directories, and is essential for any .NET developer who needs to read or write data to a file.
Creating a TextWriter Object in C#
To create a TextWriter object, you must first define the file path or stream that the data will be written to. You can then use the following code to create a TextWriter object:
Loading code snippet...
This code creates a new instance of StreamWriter, which inherits from TextWriter, and specifies the file location where the data will be written.
It is important to note that when you are finished writing to the file, you should always close the TextWriter object to ensure that all data is written and the file is properly saved. You can do this by calling the Close() method on the TextWriter object, like so:csharp tw.Close();
By closing the TextWriter object, you also release any system resources that were being used to write to the file, which helps to prevent memory leaks and other issues.
Writing Text to a File using TextWriter
To write text data to a file using TextWriter, you can use the WriteLine method, which writes a string followed by a line terminator. You can also use the Write method, which writes a string without a line terminator:
Loading code snippet...
It is important to note that when using TextWriter to write to a file, you must remember to close the file after you are finished writing to it. This can be done using the Close method, which ensures that any remaining data is written to the file and that system resources are released.
Reading Text from a File using TextReader
To read text data from a file, you can use the StreamReader object, which is a concrete implementation of TextReader. You can then use the ReadLine method to read the file line by line:
Loading code snippet...
It is important to note that when using the StreamReader object, you should always wrap it in a using statement. This ensures that the object is properly disposed of and any resources it was using are released. Failure to do so can result in memory leaks and other issues.
Using TextWriter with StreamWriter to Append or Overwrite Files
You can use the StreamWriter object to append or overwrite text data to a file. To append to an existing file, you can use the following code:
Loading code snippet...
The ‘true’ argument in the constructor specifies that the StreamWriter object should append to an existing file instead of overwriting it.
If you want to overwrite an existing file instead of appending to it, you can simply omit the ‘true’ argument in the constructor. This will create a new file with the same name and overwrite the existing file with the new data. However, be careful when using this method as it will permanently delete the original file and replace it with the new data.
Understanding the Role of Encoding in Writing and Reading Files with TextWriter
Encoding is an essential concept when writing and reading files with TextWriter. It enables the conversion of characters into a sequence of bytes that can be accurately decoded by other programs.Text encoding determines how the written data is represented in the resulting file. Common encoding formats include UTF-8, ASCII, and Unicode. The choice of encoding format depends on the type of data being written or read and the nature of the application.
It is important to note that using the wrong encoding format can result in data corruption or loss. For example, if a file is written in UTF-8 encoding but is read using ASCII encoding, certain characters may not be recognized and could be replaced with question marks or other symbols. Therefore, it is crucial to ensure that the encoding format used for writing and reading files is consistent and appropriate for the data being processed.
Best Practices for Handling Exceptions when Working with TextWriter
As with any programming task, it’s essential to handle exceptions that may arise when working with TextWriter. This will help ensure that any errors are appropriately handled, and that data is written or read correctly.One best practice is to use the try-catch-finally block to catch any exceptions that may occur. Additionally, you should always close the TextWriter object after writing data to ensure that it is flushed to the file and that any associated resources are released.
Another best practice is to use the using statement when working with TextWriter. This will automatically dispose of the TextWriter object and release any associated resources when the code block is exited, even if an exception occurs. This can help prevent memory leaks and ensure that your code is more efficient and reliable.
Advanced Techniques for Writing and Formatting Data with TextWriter
Text writer provides several advanced techniques for writing and formatting data to a file. These include specifying formatting options like padding and alignment or using string interpolation.
Loading code snippet...
The above code uses placeholders to specify padding and alignment when writing data to a file.
Another advanced technique that TextWriter provides is the ability to write data to a file asynchronously. This can be useful when dealing with large amounts of data or when performance is a concern. By using the WriteAsync
method, you can write data to a file without blocking the calling thread.
Additionally, TextWriter allows you to specify the encoding to use when writing data to a file. This can be important when dealing with non-ASCII characters or when working with files that need to be compatible with specific systems or applications. The Encoding
property of the TextWriter class can be used to set the encoding to use when writing data to a file.
Conclusion
TextWriter is an essential class when working with text data in C#. It provides an efficient and flexible way to write data to a file, and with its various advanced features, can be used to perform complex text-based I/O operations with ease. By employing the best practices outlined in this article, you can effectively use TextWriter to create powerful text-based programs in C#.