How to Use C# StreamWriter for File Writing
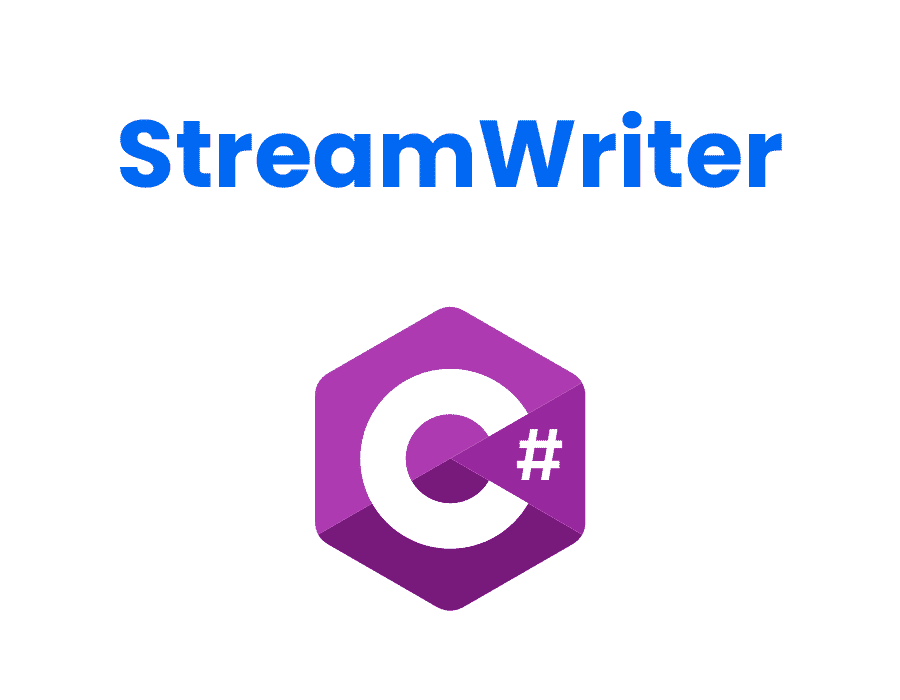
If you are a software developer working with C# or .NET, chances are that you need to save data in files as your application runs. This can be achieved using StreamWriter, a C# class that provides a streamlined way of writing text data to files. In this article, we will delve into the nitty-gritty of StreamWriter and discuss how to implement it in your code.
Introduction to StreamWriter and File Writing in C#
StreamWriter is a class in the System.IO namespace that facilitates writing text data to files. In C#, it is one of the readily available options to perform file writing operations. Similar to other file writing mechanisms, StreamWriter enables you to create, read, write, and append text data to a file. However, StreamWriter’s primary strength lies in its capacity to convert the text into bytes and write them to a file.
StreamWriter also provides a variety of options for formatting the text data before writing it to a file. For instance, you can specify the encoding type, which determines how the text is converted into bytes. Additionally, you can set the buffer size, which determines how much data is written to the file at once. These options can significantly impact the performance and efficiency of your file writing operations.
Another advantage of using StreamWriter is that it automatically handles the file stream’s opening and closing, making it easier to manage file operations. StreamWriter also provides error handling mechanisms, such as exceptions, to help you identify and resolve any issues that may arise during file writing operations.
Understanding the StreamWriter Class and its Properties
The StreamWriter class is a powerful tool that comes packed with many properties, making it convenient to handle different situations while writing data to files. Some of the most common properties of StreamWriter include AutoFlush, BaseStream, and NewLine among others. AutoFlush automatically writes any buffered data to the stream after each writeln command, ensuring that the class does not accidentally close the file. BaseStream, on the other hand, returns the underlying stream, while NewLine sets up the line separator of your choice, by default being /n.
Another important property of the StreamWriter class is the Encoding property. This property allows you to specify the character encoding used when writing to the file. By default, StreamWriter uses UTF-8 encoding, but you can change this to any other supported encoding such as ASCII, Unicode, or UTF-32. This is particularly useful when working with files that require a specific encoding, such as international text files.
Setting up a New StreamWriter Object in Your Code
To use StreamWriter in your application, the first step is to create a new object of the StreamWriter class. This is done by invoking one of its constructors that define its instance parameters. At this stage, you are required to provide details of the file path where you intend to write the data. This is achieved by creating a new instance of the StreamWriter class and passing the file path as its parameter. The file path can be defined in various ways, such as an absolute directory, a relative directory, or a combination thereof.
Once you have created a new StreamWriter object, you can start writing data to the file. To do this, you can use the various methods provided by the StreamWriter class, such as Write, WriteLine, and WriteAsync. These methods allow you to write data to the file in different formats, such as text, binary, or XML.
It is important to note that when you are done writing data to the file, you should always close the StreamWriter object. This is done by calling the Close method of the StreamWriter class. This ensures that any remaining data in the buffer is written to the file and that the file is properly closed. Failure to close the StreamWriter object can result in data loss or corruption.
Writing Text to Files using StreamWriter: Basics and Syntax
After setting up a new StreamWriter object, you can proceed to write text to the file using the Write() and WriteLine() methods. WriteLine() automatically adds a new line character at the end of the line, making it a more convenient option when writing multiple lines of text. On the other hand, Write() does not add the newline character unless stated, making it more suitable for writing words and phrases without starting a new line. Although the syntax of StreamWriter is simple and straightforward, it still calls for some level of experience to use effectively.
It is important to note that StreamWriter also allows you to specify the encoding of the file being written. This is particularly useful when dealing with files that require a specific encoding, such as UTF-8 or UTF-16. To specify the encoding, simply pass it as a parameter when creating the StreamWriter object. Additionally, StreamWriter also provides methods for flushing and closing the file after writing, ensuring that all data is written to the file and that it is properly closed to prevent any data loss or corruption.
Creating and Writing to New Files with StreamWriter
The primary role of StreamWriter is to enable you to create new files and write data to them. To create a new file, simply specify the name of the file and the extension you want to use. This can be done using the File>Create method, which creates a new file if one does not already exist in the specified location. Afterward, use the StreamWriter object to write text to the newly-created file using the Write() or WriteLine() methods.
It is important to note that when using StreamWriter to write to a file, you must remember to close the StreamWriter object once you are finished writing. This is because the data you write is often buffered in memory, and may not be written to the file until the StreamWriter object is closed. Failure to close the StreamWriter object can result in data loss or corruption.
Additionally, StreamWriter can be used to append data to an existing file, rather than creating a new file. To do this, simply specify the name of the existing file when creating the StreamWriter object, and set the append parameter to true. This will allow you to add new data to the end of the existing file, without overwriting any existing data.
Appending Text to Existing Files using StreamWriter
StreamWriter also facilitates appending data to existing files. This is achieved by setting the second parameter of the StreamWriter constructor to the Boolean value ‘true.’ Doing so sets the StreamWriter object to open the existing file and position the write pointer at the end of the file, which ensures that the new text gets added to the end instead of overwriting the existing contents.
Reading and Writing Data from Text Files using StreamReader and StreamWriter
While StreamWriter enables you to write data to a file, StreamReader facilitates reading data from a file. When implemented together, the two classes allow you to implement a simple file reader and writer. To read data from a file using StreamReader, create a new instance of the StreamReader class and use its ReadLine() method to read the content line by line. Once you’ve read the data, use StreamWriter to write the modified data back to the same file or a new one.
Handling Exceptions while Writing to Files with StreamWriter
When writing data to a file, several exceptions can occur. For instance, in the case where the file path is incorrect or the program does not have write access to the specified directory. To prevent the application from crashing, you need to handle such exceptions properly. StreamWriter’s primary exception is the IOException, which it throws whenever it encounters a problem while writing data to a file. To handle exceptions effectively, use try-catch statements to capture the exception and provide a user-friendly error message.
Best Practices for Using C# StreamWriter for File Writing
While StreamWriter is easy to use, there are several best practices that will help you get the best results. These include flushing the buffer each time you write data to the file and closing the StreamWriter instance whenever you are done writing the data. Doing so enhances the performance of both your application and the file systems, as it frees up the resources being used by your application.
In conclusion, C# StreamWriter is a powerful and straightforward way of writing text data to files, and it is suitable for projects of any size. By following the steps outlined in this article, you’ll be well on your way to using StreamWriter effectively and delivering excellent results with your applications.