Selenium Interview Questions and Answers
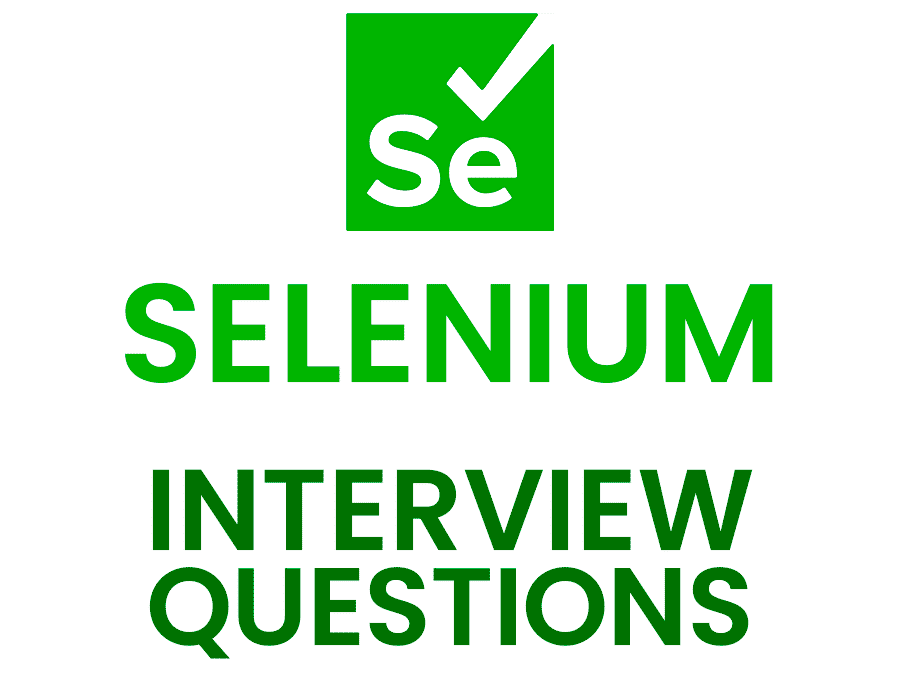
Are you preparing for a Selenium C# interview and looking for some challenging questions to test your knowledge? You’ve come to the right place! In this article, we will discuss some of the most commonly asked C# interview questions for Selenium testers.
These questions will help you gain a better understanding of Selenium, C#, and web automation testing concepts, and give you the confidence to excel in your interview. So, let’s dive into the world of Selenium C# interview questions and answers and learn how to tackle these questions effectively!
What is Selenium, and can you explain its main components?
Answer
Selenium is an open-source web testing framework that allows you to automate browser actions and test web applications. It supports various programming languages, including C#, Java, Python, and Ruby. Selenium has become a popular choice for web automation testing due to its flexibility and ease of use.
The main components of Selenium are:
- Selenium WebDriver: It is a browser automation API that allows you to interact with web elements, perform actions like clicking, typing, and navigating through web pages. WebDriver supports multiple programming languages and browsers, including Chrome, Firefox, Edge, and Safari.
- Selenium IDE: It is a browser extension that provides a record-and-playback functionality for creating and editing test scripts. Selenium IDE allows you to record your browser interactions and export the test script in various programming languages. However, it has limited capabilities compared to WebDriver and is not suitable for complex test scenarios.
- Selenium Grid: This component allows you to run tests in parallel across multiple browsers and platforms. Selenium Grid helps in reducing the overall test execution time by distributing test cases across various machines. It is particularly useful for large-scale test automation projects and cross-browser testing.
- Selenium RC (Remote Control): It is a deprecated component of the Selenium suite, and it has been replaced by Selenium WebDriver. Selenium RC was used for executing test scripts in multiple browsers by launching them as separate processes. However, due to its limitations and the introduction of WebDriver, Selenium RC is no longer in use.
How does Selenium WebDriver work in C#?
Answer
Selenium WebDriver is a browser automation API that allows you to control browser actions programmatically. In C#, you can use the Selenium WebDriver library to interact with web elements and automate web application testing. Here’s a high-level overview of how Selenium WebDriver works in C#:
- Install Selenium WebDriver: To start using Selenium WebDriver in your C# project, you need to install the
Selenium.WebDriver
package from NuGet Package Manager. You also need to install the WebDriver for the specific browser you want to use, such asSelenium.WebDriver.ChromeDriver
for Chrome. - Create an instance of WebDriver: After installing the necessary packages, you can create an instance of the WebDriver for the desired browser. For example, to create an instance of Chrome WebDriver, you would write:
Loading code snippet...
- Interact with web elements: Once the WebDriver instance is created, you can use various methods and properties to interact with web elements on a web page. For example, you can navigate to a URL using the
driver.Navigate().GoToUrl()
method, find web elements using thedriver.FindElement()
method, and perform actions like clicking, sending keys, and more. - Perform : In a test scenario, you would typically perform assertions to verify whether the application is working as expected. You can use any C# testing framework, such as MSTest or NUnit, to write and execute your test cases.
- Close the browser: After completing the test, you should close the browser and dispose of the WebDriver instance using the
driver.Quit()
method.
What are the main advantages of using Selenium for web automation testing in a C# environment?
Answer
Selenium offers several advantages for web automation testing, particularly in a C# environment. Some of the main benefits are:
- Cross-browser and cross-platform support: Selenium supports multiple browsers, including Chrome, Firefox, Edge, and Safari, allowing you to run tests across different browsers and platforms. This ensures the compatibility of your web application across various environments.
- Language support: Selenium supports various programming languages, including C#. This allows you to write and maintain your test scripts in the same language as your application, making it easier for development and testing teams to collaborate.
- Integration with testing frameworks: Selenium can be easily integrated with popular C# testing frameworks like MSTest and NUnit. This enables you to write and execute test cases using the familiar syntax and features of these testing frameworks.
- Flexible and extensible: Selenium provides a wide range of methods and properties for interacting with web elements, making it suitable for various types of web automation testing scenarios. It is also extensible, allowing you to combine it with other tools and libraries to further enhance its capabilities.
- Open-source and actively maintained: Selenium is an open-source project with an active community, ensuring that it stays up-to-date with the latest browser technologies and features. This also means that there are plenty of resources, tutorials, and support available for learning and troubleshooting.
- Parallel test execution: With the help of Selenium Grid, you can run your tests in parallel across multiple browsers and machines, reducing the overall test execution time and increasing testing efficiency. This is particularly useful for large-scale test automation projects and cross-browser testing.
Can you list some of the commonly used Selenium WebDriver commands in C# for interacting with web elements?
Answer
Here are some commonly used Selenium WebDriver commands in C# for interacting with web elements:
- Navigating to a URL:
Loading code snippet...
- Finding a web element:
Loading code snippet...
- Sending text to a text field:
Loading code snippet...
- Clicking a button or link:
Loading code snippet...
- Clearing the text in a text field:
Loading code snippet...
- Getting an value:
Loading code snippet...
- Getting the text of an element:
Loading code snippet...
How do you handle different types of web elements such as checkboxes, dropdowns, and text fields using Selenium C#?
Answer
In Selenium C#, you can interact with different types of web elements using various WebDriver commands. Here’s how to handle some common web elements:
- Text fields: To interact with a text field, you can use the
SendKeys
,Clear
, andGetAttribute
methods. For example:
Loading code snippet...
- Checkboxes: To interact with a checkbox, you can use the
Click
method to check or uncheck it, and theSelected
property to determine its state. For example:
Loading code snippet...
- Dropdowns: To interact with a dropdown (select) element, you can use the
SelectElement
class from Selenium’s support library. For example:
Loading code snippet...
These are just a few examples of how to interact with different web elements using Selenium C# WebDriver. You can use similar methods and properties to interact with other web elements like radio buttons, buttons, links, and more.
What are the different ways to locate web elements in Selenium C#? Can you provide a few examples?
Answer
In Selenium C#, you can locate web elements using various strategies. Here are some common ways to locate web elements along with examples:
- By ID: Locates an element by its “id” attribute.
Loading code snippet...
- By Name: Locates an element by its “name” attribute.
Loading code snippet...
- By Class Name: Locates an element by its “class” attribute.
Loading code snippet...
- By Tag Name: Locates an element by its HTML tag name.
Loading code snippet...
- By CSS Selector: Locates an element using a CSS selector.
Loading code snippet...
- By XPath: Locates an element using an XPath expression.
Loading code snippet...
These are some of the most common ways to locate web elements in Selenium C#. Depending on your specific use case, you can choose the most appropriate strategy that suits your web application’s structure and elements.
Now that we’ve covered some of the fundamental Selenium C# interview questions, let’s move on to more advanced topics. These next few questions will focus on the practical aspects of using Selenium, such as handling different web elements, locating elements, and managing browser windows or tabs.
These questions will help you showcase your hands-on experience with Selenium C# and demonstrate your problem-solving skills to the interviewer.
How do you switch between multiple browser windows or tabs in Selenium C#?
Answer
In Selenium C#, you can switch between multiple browser windows or tabs using the SwitchTo()
method of the WebDriver. Here’s how to do it:
- Get the current window handle: Before switching to another window or tab, you should store the current window handle so that you can switch back to it later.
Loading code snippet...
- Get all window handles: Retrieve all the window handles associated with the WebDriver. This will include handles for all the opened windows or tabs.
Loading code snippet...
- Switch to the desired window or tab: Iterate through the window handles and switch to the desired window or tab using the
SwitchTo().Window()
method.
Loading code snippet...
- Switch back to the original window or tab: Once you are done with the actions in the new window or tab, you can switch back to the original window or tab using the stored window handle.
Loading code snippet...
By following these steps, you can switch between multiple browser windows or tabs in Selenium C# and perform the required actions in each of them.
What are the different types of waits in Selenium C#, and when should you use them?
Answer
In Selenium C#, waits are used to control the synchronization between the WebDriver and the web application, ensuring that the WebDriver waits for certain conditions before proceeding with the next action. There are two main types of waits in Selenium C#:
- Wait: Implicit wait is a global wait that tells the WebDriver to wait for a specified amount of time before throwing a “NoSuchElementException” if an element is not found. This wait is applied to all the elements in your script, and you only need to set it once.
Usage:
Loading code snippet...
When to use: Use implicit wait when you want to set a general wait time for all the elements in your script, and you have a predictable loading time for the web elements.
- Wait: Explicit wait is a specific wait that tells the WebDriver to wait for a particular condition to be met before proceeding with the next action. Explicit waits are more flexible than implicit waits, as they allow you to specify different wait conditions for different elements.
Usage:
Loading code snippet...
When to use: Use explicit wait when you need to wait for a specific condition to be met, such as the visibility of an element, the element to be clickable, or any custom condition. Explicit waits are recommended for handling dynamic web elements or AJAX-based applications where the loading time of elements may vary.
How do you handle alerts and pop-ups in Selenium C#?
Answer
In Selenium C#, you can handle alerts and pop-ups using the SwitchTo().Alert()
method of the WebDriver. Here’s how to handle alerts and pop-ups:
- Switch to the alert: Switch to the alert window using the
SwitchTo().Alert()
method.
Loading code snippet...
- Perform actions on the alert: Once you have switched to the alert, you can perform various actions such as accepting the alert, dismissing the alert, getting the alert text, or sending text to a prompt.
Loading code snippet...
- Switch back to the main window: After performing the required actions on the alert, you can switch back to the main window using the
SwitchTo().DefaultContent()
method.
Loading code snippet...
By following these steps, you can handle alerts and pop-ups in Selenium C# and perform the necessary actions on them. Keep in mind that while handling an alert, you may need to use explicit waits to ensure that the alert is present before trying to switch to it.
Can you explain how to implement the Page Object Model (POM) in Selenium C#?
Answer
The Page Object Model (POM) is a design pattern in Selenium that promotes maintainability and reusability of test scripts by encapsulating web elements and their interactions within separate classes, representing individual web pages or components of a web application.
Here’s a step-by-step guide to implementing the Page Object Model in Selenium C#:
- Create a class for each web page: Create a separate class for each web page or component that you want to test. This class will represent the page or component and contain all the web elements and their interactions.
Loading code snippet...
- Define web elements as properties: In each class, define the web elements as properties using the
FindBy
attribute to specify the locator strategy. This makes it easy to access the web elements and change their locators without affecting the test scripts.
Loading code snippet...
- Create methods for user interactions: Define methods in each class to perform user interactions, such as filling out forms, clicking buttons, or navigating between pages.
Loading code snippet...
- Initialize the Page Object in the test script: In your test script, create an instance of the Page Object class and initialize its web elements using the
PageFactory.InitElements()
method.
Loading code snippet...
- Use the Page Object methods in the test script: Now, you can use the methods defined in the Page Object class to perform actions and assertions in your test script.
Loading code snippet...
By following the Page Object Model, you can organize your test scripts better and make them more maintainable, scalable, and reusable. This approach helps you keep your test scripts clean and easy to understand, especially when dealing with large and complex web applications.
Conclusion
Congratulations on making it through these Selenium C# interview questions and answers! We hope that this article has provided you with valuable insights and a solid foundation for your upcoming interview. Remember, the key to acing any interview is practice and preparation.
By reviewing these questions and understanding the concepts behind them, you’ll be well on your way to impressing your interviewer and landing that Selenium testing job. Keep learning, practicing, and refining your skills, and you’ll undoubtedly succeed in your career as a Selenium tester in the C# ecosystem.
Good luck!