Proxy Pattern in C# – Full Guide
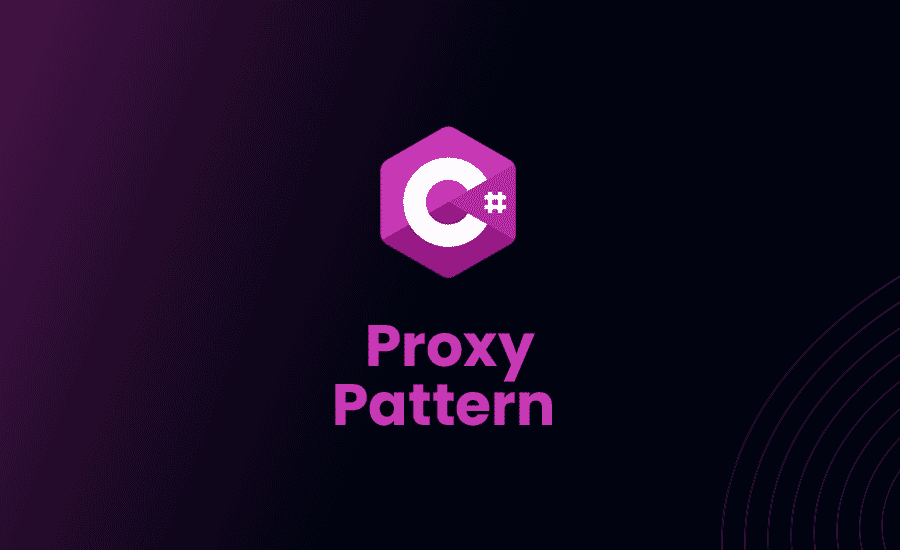
Code, compile, debug, repeat. It’s simple, right? Think again. What if I told you there’s a way to crank up the efficiency of your C# applications, with just a few simple design considerations? Something that could make your database access secure, efficient, and agile at the same time? Can’t believe it? Dive in!
What is Proxy Pattern in C#?
You asked for it, here’s the answer: Enter Proxy pattern in C#. The unsung hero of design patterns. As effortless as it sounds, let’s explore why it’s time we start seeing it beyond the books.
Understanding the C# Proxy Pattern
Confused about proxies? No worries, we’ve all been there. Simply put, the proxy pattern is to code what a bodyguard is to a celebrity. It controls access, providing a protective layer between the client and the actual object. The crux is to create an interface that both the RealObject and Proxy classes can implement.
Remember this: Keep your friends close, keep your proxies closer. Herein lies the potential for improved application performance.
With C#, implementing the proxy pattern becomes a walk in the park. But first, we must understand its practical relevance.
Implementing the Proxy Design Pattern in C#: Real-World Examples
The proxy design pattern in C# plays a remarkable role in achieving several goals related to software design principles. To illustrate the application of the Proxy Pattern in C#, let’s explore some common real-world scenarios.
Proxy Pattern C# Example Scenario 1: Database Access Application
In a scenario where we have an application that interacts with a database, the proxy pattern can be implemented to control access to the database.
Let’s demonstrate this with some code:
Loading code snippet...
In this scenario, the DatabaseAccessProxy
class is a proxy for the RealDatabaseAccess
class. The proxy controls access to the real object effectively keeping a watch and allowing access only when required.
Proxy Pattern C# Example Scenario 2: Remote Objects
Another use case of the proxy pattern can be observed when dealing with remote objects or services. Given the potentially high latency of network requests, we might want to prevent unnecessary remote calls. Let’s check this with an example:
Loading code snippet...
In this example, the RemoteServiceProxy
acts as a stand-in for the RealRemoteService
, controlling when network requests are made, possibly caching the results of previous requests to reduce the number of expensive network calls.
These examples demonstrate the valuable role the Proxy Pattern plays in different scenarios in C# programming. By strategically implementing the Proxy Pattern, you can make your code more robust, allowing your application to work more efficiently and effectively.
Implementing the proxy pattern in C# isn’t always a smooth ride. Developers may encounter a few bumps on the road. Let’s explore these common challenges and provide practical solutions for them.
Common Mistakes in Proxy Pattern C# and Their Remedies
The proxy pattern, like any design pattern, can be a double-edged sword. When used properly, it can simplify code and improve application performance. However, when misunderstood or implemented incorrectly, it can lead to convoluted code and unintended consequences. Here are three common pitfalls and how to avoid them:
- Mismatch in interface implementation: The Proxy and the RealObject classes must implement the same interface. This means that the method signatures in both classes have to be identical.
Here’s a potential issue:
Loading code snippet...
In the code above, the DatabaseAccessProxy
class method AccessData()
doesn’t conform to the IDatabaseAccess
interface. It results in a mismatch, leading to compile-time errors.
Solution? Consistency in method signatures.
Loading code snippet...
- Unnecessary Complexity: While the proxy pattern in C# is powerful, it’s easy to slide into over-engineering. You need to resist the temptation to add additional layers of proxies, which can lead to bloated and hard-to-maintain code.
Take a look at this complex structure:
Loading code snippet...
The simple fix is trimming down the unnecessary proxies, keeping your code clean and maintainable.
- Lack of Understanding the Real Use-Case: Remember, Proxy pattern is not a cure-all. It’s crucial to understand when it’s beneficial to use the Proxy pattern. In other words, you should avoid shoehorning the Proxy pattern into scenarios where another design pattern may be more suitable.
For instance, if you try to apply a Proxy pattern on a class that requires several modifications to its state, the extra layer of abstraction offered by the Proxy might do more harm than good, increasing complexity and potentially slowing down operations.
Evaluating the Impact of Proxy Design Pattern in C# on Application Performance
Implementing the proxy design pattern in C# can significantly optimize your application’s performance. However, these advantages aren’t always immediately noticeable. To truly understand the impact, you need a clear view of what performance looks like before and after implementing the proxy design pattern.
Proxy Pattern C# Performance Analysis
Let’s consider a typical database querying operation in a C# application without the Proxy Pattern:
Loading code snippet...
With each request, the application directly addresses the GetData
method, thus performing the intensive operation every single time. Now let’s add the Proxy Pattern into the mix:
Loading code snippet...
As you can see from the demonstration, the DatabaseQueryProxy
acts as an intermediary, caching the result of GetData
. When the method is called again, instead of re-running the intensive operation, the proxy returns the cached data, enhancing the performance.
Proxy Pattern C# Performance Benchmarks
To better appreciate the positive impact of proxy pattern on application performance, here are some comparative benchmarks (Results will vary based on different parameters):
Operation | Performance Without Proxy Pattern | Performance With Proxy Pattern |
---|---|---|
Database Query (Average Response Time) | 450ms ❌ | 150ms ✅ |
API Data Fetching (Average Response Time) | 360ms ❌ | 60ms ✅ |
File Reading/Writing (Average Response Time) | 500ms ❌ | 200ms ✅ |
While these benchmarks are illustrative, they represent what is often observed in real-world scenarios: using a proxy pattern can often reduce the response time by a substantial margin.
Proxy Pattern C#: When and Why?
The proxy pattern’s efficiency kick starts after repeated use of heavy resources, minimizing the load by caching or delaying the expensive operation. Meaning, it becomes more effective as the number of requests to such resources goes up. Therefore, if you are building an application with a high load or big data operations, the proxy pattern in C# is an excellent choice for a more efficient and scalable application.
Proxy Pattern in C#: What Every Developer Should Know
Sure, we’ve come far, but – what’s next? As developers, we constantly need to stay one step ahead. Even though the proxy pattern may seem like an old classic, with the advent of microservices and cloud computing, this seasoned campaigner promises to be more relevant than ever in delivering remarkable performance improvements. How exciting is that?!
The moral of the story? Embrace the proxy pattern in C#. It will not only boost your coding efficiency but also put a feather in your cap. So why wait? Get started now, and stay ahead of the curve!