Priority Queque in C# (for High-Performance Applications)
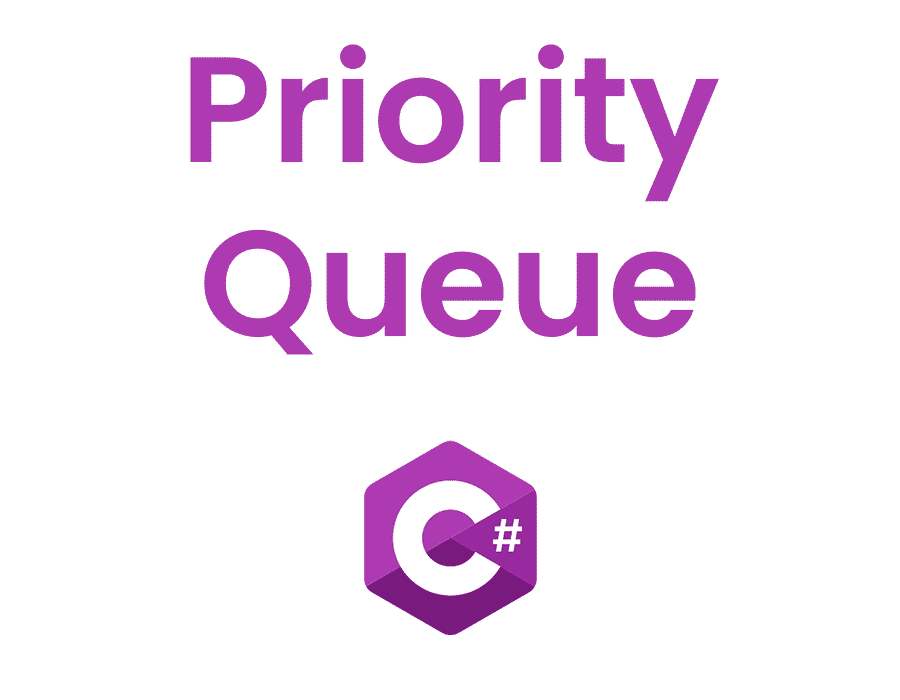
Understanding the Importance of C# Priority Queue
Priority queues play a vital role in many applications where efficiency is crucial. In this section, we’re going to dig deep into the world of priority queues in C#.
What is a Priority Queue?
A priority queue is a data structure that maintains a collection of elements, each with an associated priority, in a way that allows retrieving an element with the highest or lowest priority. It’s like a regular queue, but elements are dequeued based on their priority rather than the order they were added.
C# Priority Queue: A Key to High-Performance Applications
Using a priority queue in C# can significantly optimize the performance of your applications. By handling elements based on priority, you can ensure timely processing of critical tasks, improve resource management, and maintain efficient code execution.
Common Use Cases of Priority Queue in C#
Some typical use cases where priority queues shine include:
- Task scheduling or event handling systems
- Pathfinding in games and simulations
- Network packet management
- Medical systems for prioritizing patients
How to Implement PriorityQueue in C#
Now let’s dive into some options for implementing priority queues in C# and explore their pros and cons.
Inbuilt .NET Option: PriorityQueue C#
Starting with .NET 6, PriorityQueue<TElement, TPriority>
is part of the System.Collections.Generic namespace. This implementation is a versatile and efficient way to manage a c# priority queue. Let’s see how to use it:
Loading code snippet...
In this example, we created a simple task scheduler. Tasks are enqueued with a priority value, and as we dequeue tasks, the highest priority task is processed first.
Implementing a Custom Max Priority Queue
Sometimes, you may need additional control or functionality that the built-in class doesn’t provide. In that case, you can create a custom priority queue using a SortedDictionary<TKey, TValue>
.
C# Dequeue, Enqueue, and Peek Operations
In this custom implementation, you’ll need to handle enqueue, dequeue, and peek operations. Here’s an example implementation of a max priority queue:
Loading code snippet...
Now you have a flexible max priority queue that can be extended to include additional functionality as needed.
Getting Started with C# Priority Queue Example
To really drive home the concepts, let’s take a look at a real-world example of using priority queues in C#.
Priority Queue C# Example: Building a Task Scheduler
Suppose you’re building a task scheduler that processes tasks based on their priority. You can use a priority queue to efficiently handle these tasks. We already saw the basics in the inbuilt .NET option example. You can extend that approach to handle more complex tasks or scenarios.
Minimum Priority Queue: Solving the Minimum Increment to Make Array Unique Problem
Consider a variation of the popular “Minimum Increment to Make Array Unique” problem. You have an array of integers, and you want to make all elements unique by incrementing them. You need to calculate the minimum number of increments required.
PriorityQueue Comparator: Creating a Custom Comparator for Our Example
Here’s how you can handle this problem with a minimum priority queue using a custom comparer:
Loading code snippet...
Priority Queue Custom Comparator: Addressing Different Requirements
In the example above, we used a custom comparator to create a minimum priority queue that can handle different scenarios. This flexibility allows you to handle various problems efficiently.
Delving Deeper into C# Priority Queue Examples
Let’s explore more examples and dive deeper into the applications of C# priority queues. We’ll revisit our task scheduler example, and then look at solving a more advanced instance of the “Minimum Increment to Make Array Unique” problem.
Extended Priority Queue C# Example: Building a Versatile Task Scheduler
Building on our previous task scheduler example, we’ll now extend it to better handle various priority levels using a custom task class and comparator.
Consider a scenario where you have tasks with a string description, an integer priority, and a timestamp indicating when the task was added.
Let’s define our custom Task
class:
Loading code snippet...
Now, we create a custom task priority comparator that not only compares tasks based on priority levels but also considers their timestamps in case of equal priorities:
Loading code snippet...
With these custom classes in place, we can now implement our versatile task scheduler using the built-in PriorityQueue class:
Loading code snippet...
Tackling a More Advanced Minimum Increment to Make Array Unique Problem
Let’s take a look at a more complex version of the “Minimum Increment to Make Array Unique” problem. Imagine you need to make all elements unique by incrementing them optimally while considering a limit for each value. In this case, each integer element can only be incremented up to a certain maximum value.
To handle this scenario, we’ll use a minimum priority queue with a custom element class containing the current value and the maximum limit.
Loading code snippet...
Next, we create a method to calculate the minimum number of increments required while respecting the max limit for each element:
Loading code snippet...
With this method, you can efficiently solve a more challenging variation of the problem while considering maximum limits for each integer element.
These extended examples demonstrate the versatility and practicality of priority queues in C#. By implementing custom classes and comparators, you can further enhance the applicability of C# priority queues to a wide array of situations, allowing you to solve complex problems with ease.
Extending the Functionality: C# Deque and Queue.PriorityQueue
Beyond priority queues, let’s take a look at additional data structures that can improve your code’s efficiency and flexibility.
Utilizing Deque for Flexible Operations
A double-ended queue (or deque) allows you to add and remove items from both ends. This functionality can be valuable in certain algorithms or data manipulation scenarios. In C#, you can use a LinkedList<T>
to implement a deque.
Taking Advantage of Queue.PriorityQueue for Advanced Applications
If you’re looking for even more advanced priority queue options and functionality, the Queue.PriorityQueue
library provides an extensive set of features and flexibility. This library can enhance your applications and further optimize performance.
C# Priority Queue Best Practices
Mastering priority queues in C# will empower you to tackle complex problems with ease. But, before we go, let’s take a quick peek at some best practices that will elevate your priority queue game to new heights!
How to Get Out of Lower Priority Queue for Efficient Processing
Focusing on high-priority items is great, but sometimes low-priority tasks can be left to linger. To avoid this, periodically give low-priority items a temporary priority boost, ensuring they’re not forgotten. Balance is key!
Optimizing Your Priority Queue Implementation for High-Performance Solutions
To get the most out of your priority queue, follow these tips:
- Choose the right data structure and implementation for your specific use case
- Use custom comparators to adapt priority queue behavior as needed
- Regularly maintain and update your priority queue, removing obsolete data
- Create a balance between processing high-priority and low-priority items
And just like that, poof, you’ve got a high-performance priority queue in C#!
Now, let’s try to wrap our heads around all the awesomeness that we’ve uncovered. C# priority queues can be a secret weapon for dealing with complex, real-world scenarios where the efficient management of tasks, requests, or data is essential. And guess what? You can impress everyone with your shiny new priority queue skills. Be it the powerful built-in .NET PriorityQueue class or a custom masterpiece, your applications will see a significant boost in performance.
So, don’t wait any longer! Go forth and conquer the world of C# priority queues, and let your code shine like the high-performance machine it can be. Happy coding, fellow C# enthusiasts!