IEnumerable vs List in C#: Differences and Comparison
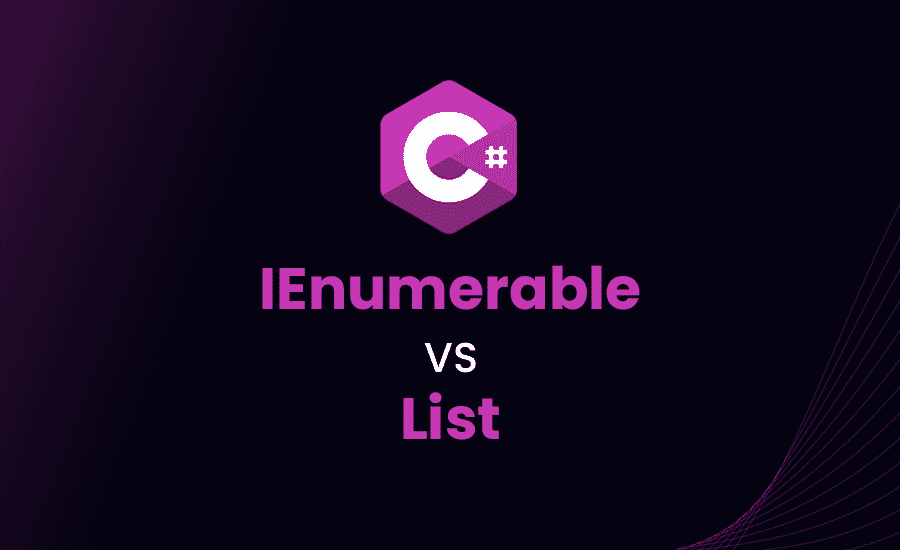
Just like how a well-organized library presents a delight to every reader, knowing about collections in C# brings joy and easiness to every programmer. Today, we’ll dive into two superstars of the C# collection world – IEnumerable and List. Buckle up, because we’re about to embark on an interesting journey!
Introduction to Collections in C#
Basic Understanding of Collections
If you’re new to C#, you might be wondering, what’s a collection? A collection in C#, my friend, is simply a group of objects. Think of it like a box where you store your favorite comic books or in our case, data. These collections come with various benefits and functionalities making our lives as developers easier. Isn’t that cool?
Importance of Collections in C#
In the labyrinth of coding, collections serve as a vital tool in managing our data. It wouldn’t be wrong to call them the heroes behind the scene of every smooth-running application. How? Well, they efficiently manage data allowing us to perform operations like adding, deleting, and modifying data easily. Would you believe me if I said that’s just the tip of the iceberg?
Understanding IEnumerable in C#
Ready to uncover the mysteries of IEnumerable
? Grab your detective hats because we’re about to delve deeper into the world of this powerful interface in C#. Let’s get started, shall we?
Definition of IEnumerable
In the realm of C#, IEnumerable
is a powerful interface that allows you to step through a collection of elements, one element at a time. To draw a picture for you, imagine you’re at a concert with a lineup of fantastic bands.
As an audience member (or programmer in our case), you would experience the concert in a particular sequence, band by band, without jumping over any performances (or in the code world, objects). That’s exactly what IEnumerable
allows you to do with your data. Here’s a brief example:
Loading code snippet...
In this code snippet, we first form a List of band names, then print out each of them sequentially on the console using a foreach
loop. As you may notice, with IEnumerable
, we cannot skip or access particular bands directly—it’s one at a time, just like at the concert.
Imagine you’re playing with your favorite toy train set. The train moves along the track one piece at a time in a specific direction. Just like the train, with IEnumerable
, we move through our data one piece at a time in a forward direction.
How IEnumerable Works
Exploring IEnumerable
is like learning about the power under the hood of a race car. When you use an IEnumerable
, it’s utilizing an IEnumerator
. It acts like a gatekeeper, marking the current position in the collection, and shifting that position for each iteration. Now, let’s see a behind-the-scenes look at our concert scenario:
Loading code snippet...
In this code, GetEnumerator
summons our gatekeeper for the concert. The MoveNext
method steers us to the next performance, while the Current
property gets us the ongoing band.
This little peek backstage uncovers how IEnumerable mechanics allows us to traverse through each element smoothly. It’s pretty much the backstage pass to your data collection concert!
Commonly Used Methods in IEnumerable
IEnumerable
is not just simple, but also very practical, bundled with various helpful methods. For instance, GetEnumerator
gets us an IEnumerator
to navigate through the collection sequentially. MoveNext
advances our hypothetical gatekeeper to the next position or element in the collection.
Last but not least, Current
serves up the current item in the collection, just as a waiter presents your favorite meal on your table. Here’s a code snippet to demonstrate:
Loading code snippet...
In this code, we use GetEnumerator
to get our meal
, then use MoveNext
to serve each dish
one by one. The Current
property fetches us each individual dish, allowing us to enjoy our meal without overloading the dinner table! Savvy, right?
That’s the charm of IEnumerable
, it allows you to have your cake (or in this case, your dish) and eat it too, but in a stepwise, efficient manner.
Advantages of Using IEnumerable
Fasten your seatbelts, dear coders. We’re now ready to dive deep into the awesome advantages of using IEnumerable
. From its flexibility to its efficient use of resources, you’ll be surprised by what this wonder tool has got in store.
Flexibility of IEnumerable
Let’s start with probably the most loved feature – the flexibility of IEnumerable
. You’re probably thinking, what’s the big deal about flexibility? Well, it’s like being able to take your bicycle almost anywhere: pavement, dirt road, or just to do neat tricks in the park because it’s so handy.
With IEnumerable
, you’re not just stuck with one type of collection, but can work with nearly every collection in C#. Yes, just like that bicycle, IEnumerable is highly versatile!
Here is a sweet code snippet for your inner nerd:
Loading code snippet...
In the above code, we can see IEnumerable
managing different types of collections such as an array, list and queue, making it into a super flexible tool.
What’s more? It supports deferred execution. This means, like that super lazy cat that only stretches when it’s time to move, IEnumerable
only executes when you ask it to. This efficient performance aspect of IEnumerable
helps to ensure that our program stays as agile and responsive as possible.
Lazy Execution in IEnumerable
What? Let’s unpack this lazy execution thing. Imagine a magical book that only opens and shows content when you want to read it, and not before. This keeps the suspense intact and also saves space. This is exactly how IEnumerable
operates with its deferred or lazy execution.
The data isn’t fetched until you begin iterating through the collection. IEnumerable, being the ever-efficient star of the show, knows how to manage resources and not exert undue effort or computation until absolutely necessary.
Let’s take a look at this marvel in action:
Loading code snippet...
In this seemingly simple code, we first create a range of numbers from 1 to 10, and then we want to find out the even numbers from this collection. But notice this: until we arrive at the foreach
loop to actually enumerate and print those even numbers, no computation or filtering takes place. IEnumerable
waits until the last possible moment before doing any work.
Memory Efficiency of IEnumerable
And ah, memory! In the vast landscapes of coding, efficient memory usage is like finding an oasis in the desert. IEnumerable, with its sage-like wisdom, only holds onto the current item in the collection during execution.
Let’s see an example:
Loading code snippet...
This code generates a huge sequence of numbers, from 1 to MaxValue of an integer. But even if we run this loop a billion times, IEnumerable
won’t bat an eyelid. Why? Because it only keeps the current number in memory and discards it as soon as the loop iterates to the next number. Just when you thought this couldn’t get any cooler, right?
When to Use IEnumerable
If you think of IEnumerable
as a wise storyteller, you’ll understand that every line of every story is kept hidden until the perfect moment. It’s a master of suspense, unraveled piece by piece when we need it. In this section, we’ll dive into the captivating tales IEnumerable
weaves in code and data management.
Use Cases of IEnumerable
IEnumerable
knows a time and a place for its tales. If you have a vast library of stories (or a large amount of data) and you want to go through them one by one, IEnumerable
is your best bet. The beauty lies in its careful conservation – no showing too much too soon, just the right amount at the right time!
Think of a customer feedback system in an e-commerce application. You have millions of feedback but you only want to show a specific number at a time – this is where IEnumerable
fits in perfectly!
Loading code snippet...
In this code, we’re using IEnumerable
to create a method that fetches a specific number of feedbacks based on the page number and size. We use IEnumerable
because we don’t have to load all feedbacks into memory – instead we skip to the specific subset of feedbacks we need to display. Imagine if we were to load all feedbacks into memory – a nightmare!
Examples of IEnumerable Usage
IEnumerable
not only tells stories but also writes a fantastic tale about lazy execution. Remember our evenNumbers
example earlier? Yes, that’s pure genius working behind the scenes. But let’s tell some new tales, shall we?
Imagine you’re working on a text processing system where you need to filter and process words from a document:
Loading code snippet...
In the above code, we’re fetching all words from a document and applying a filter to get only the words with more than four characters.
Now, keep in mind this operation could potentially be over millions of words but thanks to IEnumerable
, until you specifically ask for filteredWords
, nothing is executed – just defined. Imagine if it were a List – you’d be loading all words in memory as soon as GetWordsFromDocument
is called. Yikes!
Hold on, here’s another scenario. Suppose you are building a game score tracker for a neighborhood cricket league:
Loading code snippet...
In the example, all players’ data isn’t fetched and sorted immediately – the actual operation is deferred until we iterate over topPlayers
. Imagine doing this for hundreds of cricket matches without overloading memory. Big win!
To bring back the 8-year-old analogy, think of IEnumerable
like opening your advent calendar each day leading up to Christmas. Instead of opening all doors at once (and spoiling the surprise!), you only see what’s behind today’s door. More surprises, less memory overload. Talk about smart, huh?
Understanding List in C#
So you understand IEnumerable
, great! What’s next? Let’s dive into List
, another gem in C#. Just imagine being handed a magic box – a box that can hold anything and morphs its size according to its contents. Exciting, right? In the following sections, we’ll unravel more about how this magic box, known in programming jargon as List
, works its wonders.
Definition of List
In a more technical sense, List
in C# is like a dynamic array, allowing direct access and manipulation of data elements using an index. Unlike arrays, though, it shrinks and grows dynamically as you add or remove items. This is just like how you’d store your collection of favorite toys – adding new ones to your collection and giving away a few and still keeping them in the same storage box without needing a bigger or smaller one each time. Cool, right?
In C#, a List could be defined and initialized like this:
Loading code snippet...
In this example, we create a List
named ageList
that will store integer values, and we add 5 age values to it at the time of initialization.
How List Works
It’s fascinating how a List
manages to size itself dynamically. Under the covers, it leverages arrays but with added flexibility. When you put items into a List
, it’s stored in an underlying array. If the List
is about to exceed the size of this array (as we keep adding more items), it automatically makes a larger array and copies the elements over. The new array is typically twice the size of the old one.
Here, your favorite cartoon characters come to rescue! Just imagine Tweety Bird who eats a lot, growing bigger (and slightly scarier), to a size where even Sylvester can’t mess with. That’s how the List
works! As you keep stuffing it with more elements, it grows automatically to accommodate the new guest elements!
Commonly Used Methods in List
Akin to your multi-port USB hub connecting several devices at once, List
introduces a wide variety of methods for different operations, transforming it into a superpowered array. It’s really more than just a storage box for your elements!
Let’s take a tour of a few of these methods and how they work:
- Add(): As simple as it sounds, this adds an element at the end of a
List
. Seemingly simple, but oh-so-powerful!
Loading code snippet...
In this example, we create an empty list and add 10
to it using the Add
method.
- Remove(): This method removes the first occurrence of a specific object from the
List
. It’s like removing a stray cat from the line of your favorite plush toys.
Loading code snippet...
Here, we first create a list of colors and then remove the first occurrence of "blue"
from it using the Remove
method.
- Contains(): This checks if an element exists in the
List
. Can’t remember if you have that Pikachu in your Pokemon collection?Contains
is here to help.
Loading code snippet...
In this case, we first define a list of Pokemon and then use the Contains
method to check whether "Pikachu"
exists in the list.
Bear in mind, these are just the tips of an iceberg. List
has many more methods (like Sort()
, Find()
, Clear()
, Insert()
, to name a few) that can do wonders with your data. It’s like the Batman’s Utility Belt of your data management!
Advantages of Using List
Stepping into the world of Lists in C# is like acquiring a superpower, offering direct access to elements and effortless manipulation of data, and with superior performance as the cherry on top. Intriguing? Let’s delve into these super-abilities of Lists.
Direct Access to Elements in List
One of the top benefits of using a List
is the inherent capability to access elements directly. This feature of List
is like a magical doorway that brings you right in front of the item you need in an instant. How? Just by using the index of the item, akin to directly dialing up a friend on your phone!
Loading code snippet...
In the above code, we directly access the third element (0-index based) of our list using the index number [2]
. Imagine having a magic door that lets you go instantly to any room (item) in your house (list) – that’s List
for you!
Now, let’s say we want to insert an element at a specific position. That’s a breeze!
Loading code snippet...
In this example, happy-go-lucky List allows us to insert a number 3
right at the third position, restoring the natural order of our number line up.
Ease in Manipulating Elements in List
List
is not only a great collector but also a master manipulator. The level of control List
provides on the data is like building your dream LEGO castle, brick by brick.
Loading code snippet...
In the example above, we first create a grocery list. Then List
flexes its muscles – adding ‘Apples’ to the list and then removing ‘Eggs’. You can basically cook up a data feast with all these manipulations!
Better Performance in Certain Scenarios
Just like the fastest kid in the block, List
excels in performance when it comes to accessing or manipulating data. This is especially true when frequent add or remove operations are required.
Loading code snippet...
In this case, we have a list of numbers. First, we add more numbers to the list. Next, we remove the first five numbers. See how efficiently List
manages all these operations? That’s like removing and adding LEGO bricks from your castle effortlessly!
Imagine trying to drink a melted chocolate bar through a straw (hint: it’s tough!). That’s just how iterating through large lists with IEnumerable
feels like sometimes. On the other hand, List
is like having a spoon to directly gobble up that chocolate – it’s fast, efficient, and satisfying!
So, remember, whether it’s tweaking the position of your LEGO castle’s window, or fetching the red apple from a box full of fruits, List
gets the job done with speed and precision. Furthermore, similar to a child confidently constructing a LEGO masterpiece knowing that any misplaced bricks can be easily moved around, you can be equally assured knowing that manipulating data using a List
is just as straightforward!
When to Use List
Our versatile and highly customizable superhero, List
, is here. But what exactly are the perfect scenarios to call upon our hero? Let’s explore List
‘s realm in detail and understand when to use it. Also, be sure to keep an eye out for some hands-on examples. Let’s get started, shall we?
Use Cases of List
Lists are incredibly versatile and useful, similar to a Swiss Army Knife that you can rely on in numerous situations. When you’re dealing with a collection of a small to medium size and you need to frequently manipulate your data, a List
is your best ally. It comes packed with a whole suite of methods to add, remove, find, sort and much more.
Further, List
offers random access by index, meaning you can directly reach any data spot like you would switch to any TV channel in one click! It’s wonderful for those scenarios where your dataset size isn’t very large as a List
brings all data into memory.
In contrast to IEnumerable
, List
does not implement deferred execution. Each time you query it, the whole data set gets loaded immediately. If your application demands data to be readily available with frequent manipulation, call upon our hero List
!
What’s more, if you’re working with LINQ operations and you’re certain to enumerate the data more than once, it’s more efficient to use List
as it prevents multiple enumerations.
Examples of List Usage
Let’s dig into some hands-on examples of List
, so strap in, folks! We’re about to roll!
Here’s an example of adding numbers to the List
and removing specific numbers:
Loading code snippet...
In the code above, we first create a list with numbers 1, 2 and 3. We then add the numbers 4 and 5 to the List. We proceed to remove the number 1 from the list and finally use a foreach
loop to print the remaining numbers.
But what if you want to clear the entire list? Or what if you’re not sure whether the data exists and you want to avoid duplication? Or how about sorting your data or reversing it? Well, List
has got you covered. Check this out in the following code:
Loading code snippet...
In this code snippet, we first create a list of numbers, 3, 1 and 2. We check if the list contains the number 4 and if it doesn’t, we add it to the list. Next, we use the Sort
method to order the numbers in ascending order and the Reverse
method to reverse the order of the data. Finally, we use Clear
to erase all the elements and print the count, which remains 0 after clearing.
Here’s a simpler analogy, consider you’re playing with numbered blocks with an 8-year old. By using List functionalities, you are able to place new numbers or blocks (Add
), remove any specific block (Remove
), clear the table (Clear
), or set the blocks in rising or falling order (Sort
& Reverse
). Isn’t it pretty convenient and creative?
Key Differences Between IEnumerable and List
The complex but fascinating world of IEnumerable and List invite us to explore them in more detail. This journey will unveil the significant differences that lie beneath their surface, making them distinct yet equally powerful. Ready to examine their iteration, access to elements, memory usage, and performance? Let’s dive right in!
Iteration in IEnumerable and List
Iteration is an essential aspect when dealing with collections, making it the linchpin in differentiating IEnumerable and List. With IEnumerable, it’s a forward-only journey, almost like being on a moving walkway at the airport. You’re moving one step at a time, keeping your foot only on the currently visible step.
In contrast, List gives you the luxury to randomly access elements. Imagine IEnumerable is like using a standard video player, where you can only fast forward or rewind one frame at a time. But List, it’s like a fancy video player with thumbnails preview and random access. You can directly hop on to any frame you want without any detour!
Let’s check this out with an example:
Loading code snippet...
In the above code, with IEnumerable, you can only go from 1-5 in order, but with List, you can directly access the third number, which is ‘3’.
Reminds you of a cute little kid playing hopscotch, doesn’t it? With IEnumerable it’s like the standard way of playing, hopping from one square to the next in order. But with List, the kid can jump straight to the third square (or any square) directly. In coding terms, that’s our superpower of random access!
Access to Elements in IEnumerable and List
Access to elements signifies how conveniently you can reach your data while coding. IEnumerable offers cursor-like access, just like using a joystick in a video game where you can only move forward.
On the other hand, List extends the console (pun intended) – it’s like you’ve unlocked a cheat code that allows you to teleport anywhere in the game (collection). You get direct access to elements using an index, making data manipulation fast and straightforward.
Loading code snippet...
In the code above, to access an element in an IEnumerable, we need to use a method like ElementAtOrDefault
. In contrast, Lists provide us direct access using the index. It’s like flipping directly to a page in a book, thanks to the page number!
Memory Usage of IEnumerable vs List
Memory usage is another parameter where IEnumerable and List show substantial differences. IEnumerable is economical in terms of memory usage, only loading the required information into memory when required. Imagine having a music player that only downloads the current track for playing instead of downloading the entire playlist. That’s what IEnumerable does!
In contrast, List is like a DJ who downloads the entire playlist beforehand so that they can quickly skip and play any song directly. Therefore, Lists can offer better performance at the cost of increased memory use.
Loading code snippet...
The snippet above demonstrates that the IEnumerable only loads the current “Alice” into memory during enumeration, but for List, all items {“Alice”, “Bob”, “Charlie”, “Dave”} are loaded into memory allowing direct access to any of these elements.
Performance Differences of IEnumerable and List
When it comes to performance, IEnumerable and List have their unique strategies. It’s a titanic clash between efficiency and swift execution, each displaying their might in different scenarios. Uniting their differences in two distinct categories – loading and accessing data can give us a clear comparison.
Where does each shine brightest?
Loading Data
IEnumerable
: This is where IEnumerable plays its trump card known as lazy or deferred execution. It doesn’t panic and load all the data at once, but rather calmly loads one item at a time when required. This strategy allows IEnumerable to run long marathons (large datasets) without breaking a sweat (consuming less memory resources).List
: In contrast, a List is like the excited kid that can’t wait to open all its presents. It eagerly loads all the data at once into memory. While this might seem intensive, it’s crucial for its fast and direct data retrieval power.
Accessing Data
IEnumerable
: With its forward-only approach to access the elements, IEnumerable is like the cautious explorer carefully moving ahead one step at a time. It might seem slow but is really efficient when the path (data size) is long and winding.List
: On the flip side, List is like a daring adventurer with the power to instantly teleport to its destination. It leverages this power to randomly access any element in a jiffy, offering superior speed in scenarios requiring frequent data manipulation.
Excited for a quick glance over their distinctive performance? Here’s a handy table for you:
Aspect | IEnumerable | List |
---|---|---|
Loading Data | Lazily loads each item when required | Promptly loads all items at once |
Accessing Data | Forward-only, one item at a time | Direct and random access to any item |
Best for | Large datasets due to its efficient resource usage | Small to medium datasets requiring frequent data manipulation |
Therefore, whether you choose IEnumerable or List entirely depends on your performance needs and resources constraints. It’s not really about which one is the winner but about which one wins for you. Remember, they may have different strengths, but they are both champions, each in their own right!
Selecting Between IEnumerable and List
Choosing the right collection for your C# application can sometimes feel like choosing the winning team in a soccer match. Will it be IEnumerable? Or will the List take the trophy home today? No worries! Let’s debunk this mystery together. In the following sections, we’ll dissect when to use which, based on the demands of our programming tasks. Trust me, this journey is going to be as fun as a rollercoaster ride!
Decision Based on Use Cases
You’ve often heard that for every problem, there’s an appropriate tool. It’s like using a paintbrush for an artistic painting and using a hammer for nailing. The same wisdom applies to programming and picking between IEnumerable
and List
.
Let’s consider the scenario of calculating the total number of characters from a collection of large books. If you use a List
, it will load the entire collection in memory just to count the characters, which might be inefficient. Here, IEnumerable
is your true savior:
Loading code snippet...
The example code retrieves all books from a library (in reality, a massive database) one by one, counts the characters, and does it efficiently.
Consider another scenario. Suppose you’re building a scoring system for a game. You’ll constantly need to add, update, or remove scores, in which case using a List
would be much more convenient:
Loading code snippet...
Just like Lego blocks, we easily manipulated the scores collection to meet the game requirements.
Choosing between IEnumerable
and List
is all about understanding your needs and picking the right tool that addresses those needs efficiently.
Decision Based on Performance Requirement
Much like picking the fastest car for a racing game, performance often governs our choice of collections in C#.
If you’re building a real-time app showing the available seats in a movie theatre, choosing List
can offer the ability for quick, immediate updates:
Loading code snippet...
Here, a List
lets us instantly update the status of available seats, keeping audiences up to date precisely when they’re booking.
On the flip side, consider you’re building an appliction to analyze huge volumes of historical weather data. In this case, IEnumerable
is your solution. With its magic of deferred execution, it ensures that memory usage is as efficient as possible:
Loading code snippet...
When performance is a concern, think about the nature of your application and the size of data you’re dealing with. Performance requires the right balance of speed and efficiency.
Importance of Flexibility and Efficiency
In the discussion of IEnumerable
and List
, it’s not always black and white. The charm lies in the grey areas- flexibility and efficiency.
Picture this: you’re asked to color a picture but you’re undecided about whether to use watercolors (flexible) or colored markers (efficient). The C# collections quandary is similar – IEnumerable
being the flexible watercolors and List
the efficient markers.
IEnumerable is like a shape-shifter, transforming itself to work with almost any collection in C#. Additionally, its ability to execute queries only when necessary (deferred execution
) saves us valuable computation power, making it a strong choice when working with large datasets. On the other hand, if constant data changes are involved, List
dances in with its wide array of versatile List-only methods for an impeccable performance.
Converting Between IEnumerable and List
You know how you can easily switch channels on your TV? Well, C# has a similar feature that allows us to switch between IEnumerable
and List
. Pretty handy, huh? But, it’s not always a straight-forward job. A single mistake could make your application behave like a snail on a treadmill!
So, let’s take a closer look at the conversion process and make sure that doesn’t happen.
Tips on Safe and Efficient Conversion
Alright folks, we’re about to learn a cool trick! Have you ever faced a situation where you found yourself with an IEnumerable
when you really needed a List
? Don’t fret. C# has got your back! Turning an IEnumerable
into a List
is as simple as casting a spell in a Harry Potter book!
Let’s see this magical trick in the following example:
Loading code snippet...
In the above code, we first define an IEnumerable
of numbers, and then godspeed, wave our magic wand — use the .ToList()
method — and poof! It’s transformed into a List
type. We then iterate through the numbersList
and display each number on the console. How’s that for easy peasy!?
Let’s say we want to perform the inverse operation: converting a List
to an IEnumerable
. It can be done implicitly as all List
types are inherently IEnumerable
. Here’s how it works:
Loading code snippet...
In the code snippet above, we simply assign our numbersList
to an IEnumerable
type variable named numbersEnumerable
. Voila! We now have an IEnumerable
.
Common Pitfalls to Avoid
Of course, if life were all roses and rainbows, we wouldn’t need superheroes, would we? While converting is a useful trick, remember not to misuse it. It’s like overusing the Expelliarmus spell; that works against Voldemort, but it could signal a novice in other wizarding duels!
While casting an IEnumerable
to a List
— much like refilling your snack bowl — seems tempting, be aware of the underlying implications. This step moves your lazy IEnumerable
to an eager-beaver List
, loading all items in memory at once. This could be nothing short of a disaster if you’re dealing with a large amount of data. It’s as if you’re trying to cram an elephant into a suitcase — it just won’t fit!
Consider this real-world example:
Loading code snippet...
In this scenario, we’re generating a range of one million numbers using Enumerable.Range()
. The result is an IEnumerable<int>
that can handle this without blowing up the memory, thanks to its deferred execution. But when we try to convert this IEnumerable
to a List
(which attempts to load everything into memory), it raises an OutOfMemoryException
.
Conclusion: IEnumerable vs List in C#
Summary of Differences
In the race between IEnumerable
and List
, it’s a tie! Both are winners in their own unique ways. IEnumerable
is the flexible runner with its compatibility and lazy execution, while List
wins on manipulating data and quick element access.
Best Practices in Choosing Between IEnumerable and List
Just like any seasoned developer would advise, when it comes to picking IEnumerable
or List
, always consider the context, purpose, and resources. Pay attention to factors like memory usage, performance requirements, data size, and access needs. It’s like choosing the right attire for the right occasion!
Well, I hate to end our little adventure here, but remember our heroes IEnumerable
and List
are bravely serving in our codebase, making our lives easier every day. Isn’t it fascinating to know how similar yet different they are? Who knew coding could bring such deep philosophical insights! All in a day’s work for us developers, I guess. Until next time, Happy Coding!