Entity Framework Interview Questions and Answers
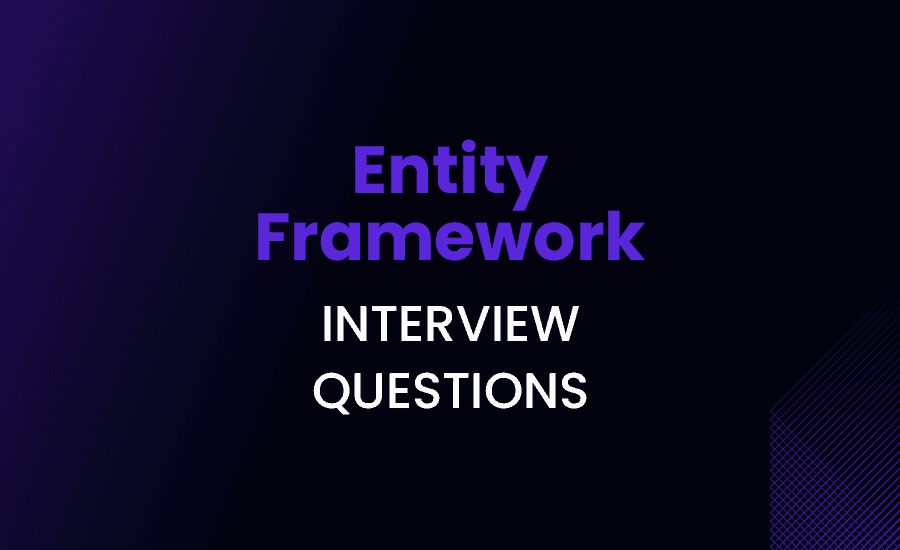
Hello to all developers, tech enthusiasts, and aspiring coders! We’re about to dive into the compelling world of C# and Entity Framework, the dynamic duo that is increasingly shaping the landscape of .NET development.
Preparing for a crucial job interview or merely looking to challenge and expand your knowledge? We’ve got your back. With this comprehensive list of C# Entity Framework interview questions, you’ll get a glimpse into the practical, problem-solving aspects of this powerful technology.
These well-curated EF Core interview questions will certainly widen your knowledge base and also demonstrate how to explain intricate concepts in the simplest manner.
So, let’s set the gears in motion – your journey into Entity Framework awaits!
What is the Entity Framework in C#?
Answer
Entity Framework (EF) is an Object-Relational Mapping (ORM) framework for .NET. It enables developers to work with data as strongly typed objects, abstracting the underlying database where the actual data is stored. This means that as a developer, you can focus on the business logic of your application, rather than on data access fundamentals.
Entity Framework supports a wide array of database systems, from SQL Server and MySQL to non-relational databases. It provides many services, including change tracking, identity resolution, lazy loading, and query translation. With Entity Framework, you can interact with your data using LINQ queries, strongly typed C# code, and even raw SQL.
In a nutshell, Entity Framework is a set of technologies in ADO.NET that support the development of data-oriented software applications.
How does Entity Framework simplify data access in .NET?
Answer
Entity Framework simplifies the process of data access in .NET in several ways:
- Abstraction of Database Code: Entity Framework abstracts the database code. Developers can perform CRUD operations without having to write SQL queries.
- SQL Injection Protection: With Entity Framework, data access is performed through a model, so there’s little risk of SQL Injection attacks.
- Database Schema Changes: EF allows developers to manipulate database schema through the model, making changes easier to handle.
- LINQ Support: Entity Framework provides support for Language Integrated Query (LINQ), making it possible to use C# syntax to query the database.
- Migration Support: Entity Framework has built-in tools for database migration, which can prove very handy in managing schema changes over time.
What are the key components of the Entity Framework?
Answer
Entity Framework comprises several key components:
- Entity Data Model (EDM): This is the core of Entity Framework, representing the data using the model classes.
- DbContext: This class is responsible for managing entity objects during run time, including change tracking and database persistence.
- DbSet: Each DbSet represents a table in the database.
- LINQ to Entities: This is a query language that allows querying data in the EDM model using .NET language syntax.
- Entity SQL: This is a storage-independent query language, similar to SQL but designed to manipulate entities defined in the EDM.
- Object Services: This is the main entry point for accessing an EDM and doing CRUD operations.
What is the difference between Entity Framework and ADO.NET?
Answer
Both ADO.NET and Entity Framework are data access technologies in .NET, but there are some key differences:
- Level of Abstraction: ADO.NET provides lower level of abstraction compared to Entity Framework. With ADO.NET, you often have to write SQL queries for CRUD operations. On the other hand, Entity Framework allows you to work with data at the object level, so you don’t need to write much SQL.
- Ease of Use: For applications where the model is very complex or frequently changing, using Entity Framework can save a lot of development time. ADO.NET might be simpler and more direct for certain scenarios, especially small applications or ones with stable schemas.
- Performance: ADO.NET can be faster than Entity Framework in some scenarios because it’s closer to the metal (direct to the database connection).
Can you explain the concept of DbContext in Entity Framework?
Answer
DbContext is a class in Entity Framework acting as a bridge between your domain or entity classes and the database. It is the main class that is responsible for interacting with the database.
The primary responsibilities of a DbContext include:
- Querying the Database: DbContext allows performing LINQ queries against the database using DbSet properties.
- Change Tracking: DbContext tracks changes that has been made to the data so that correct update commands can be generated when saving changes to the database.
- Persisting Data: It translates changes that you’ve made to your objects or data into database commands and sends them to the database.
- Caching: DbContext does first-level caching of the data by default. It stores the entities which have been retrieved during the life time of a context class instance.
- Managing Relationships: DbContext navigates relationships between your objects via navigation properties.
To use DbContext, we first need to create a subclass of it and expose DbSet properties to represent tables in the database.
As we delve deeper into this superabundance of C# Entity Framework interview questions, you’ll begin to realize the integral role that Entity Framework plays in software development with .NET.
It’s not merely about understanding how to utilize DbContext or how it functions. As we transition into our next set of questions, you’ll discover how EF can be used to define the structure of your database using codes, highlighting the ingenious ‘Code First’ approach.
What is Code First approach in Entity Framework and how does it work?
Answer
The Code First approach is a strategy utilized in the Entity Framework where the data access is modeled around the domain specific objects. It provides full control over the serialized code, rather than a database designer or wizard creating it for you. This is especially powerful and beneficial in Domain Driven Design. The primary workflow of Code First is:
- Define your model objects as POCOs (Plain Old CLR Objects).
- Optionally configure the model through fluent API or data annotations.
- On querying the database, EF with its DbContext object will create all the database schema automatically for you based on your models.
- You can initiate this automatic update of database schema using the
DbContext.Database.EnsureCreated()
to create new schema orDbContext.Database.Migrate()
to update existing schema.
The Code First approach is flexible and easy to control, which makes it an excellent choice for software that needs to be robust, but also easy to update and maintain over long periods of development time.
Loading code snippet...
What is Model-First approach in Entity Framework and why is it important?
Answer
Model-First is an approach to development with Entity Framework where you begin by designing your database schema in a visual designer included with Visual Studio. After creating your data model, EF generates corresponding database and classes for you.
The steps involved in Model-First approach are:
- Designing model using the designer in Visual Studio
- Generating T-SQL script that creates all the tables based on the design.
- Running the script on an actual server to create the database.
- Entity Framework generates the entities (POCO classes) for you.
The primary advantage of this approach is that you can design your database how you want it and EF will create the appropriate code to access it. This makes Model-First a good choice for applications where the database design is expected to be more static, but complex.
Can you explain the purpose of DbSet in Entity Framework?
Answer
DbSet
in Entity Framework is a class that represents a collection for a given type. You can use DbSet to query or save instances of the class. In your context class (a class deriving from DbContext
), you create properties of type DbSet
for each entity in your model you want to interact with.
DbSet
is used to perform CRUD operations (create, read, update, delete) onto the database and it’s a fundamental part of a DbContext class. Each DbSet
represents a table in the database.
Loading code snippet...
In the above example, two DbSet
properties Students
and Classes
represent two tables in a database.
How is data manipulation handled in Entity Framework?
Answer
Entity Framework provides a straightforward way to execute Create, Read, Update, and Delete operations, commonly known as CRUD operations:
- Create: For creating new records, you can add data by creating instances of your entity type and then adding them to the DbSet instance.
Loading code snippet...
- Read: For reading data, you can use LINQ queries to get access to the data.
Loading code snippet...
- Update: For updating existing records, you can fetch the records, update properties, and then save changes.
Loading code snippet...
- Delete: For deleting records, you can remove the entity then save changes.
Loading code snippet...
Halfway through our journey exploring these Entity Framework interview questions and you’d agree, Entity Framework is like a one-size-fits-all shirt, accommodating a variety of relational database system nuances.
We’ve unwrapped the method of handling relationships between tables in EF and now, we transition into a more sophisticated topic – Migrations in EF.
Migrations allow you to evolve your database schema over time while preserving existing data. Read on as we unfold this remarkable feature further.
How can you handle relationships between tables in Entity Framework?
Answer
Entity Framework supports three types of relationships between entities or tables:
- One-to-One: In a one-to-one relationship, each row of data in the first table corresponds with data in a single row of another table. This is typically implemented using a foreign key in one of the tables referencing the primary key of another.
Loading code snippet...
- One-to-Many: A one-to-many relationship is defined as a single parent to many children. This is represented in Entity Framework by having a navigation property of a collection type in the ‘one’ class, and anther navigation property in the ‘many’ class.
Loading code snippet...
- Many-to-Many: In a many-to-many relationship, several records in the first table correspond to multiple records in the second table. In Entity Framework, this is typically implemented using a junction table.
Entity Framework allows you to build these relationships using navigation properties in your classes representing the tables. Moreover, by convention, Entity Framework will provide the foreign key relationships in the database for you.
What does Migration in Entity Framework imply?
Answer
Migrations in Entity Framework are a way to keep the database schema in sync with the application’s model classes. They allow a database to be updated to match the current state of the models and can also upgrade a database from an older to a newer version.
Migrations are typically created using the ‘Add-Migration’ command in the Package Manager Console. Each migration is timestamped and contains two methods: Up()
and Down()
. The Up()
method defines the operations needed to apply the migration (creating tables, adding fields, etc.), while the Down()
method defines how to undo the changes made in Up()
.
How is concurrency handling done in Entity Framework?
Answer
Entity Framework handles concurrency through optimistic concurrency. In simple terms, EF assumes that conflicts will be rare and does not lock objects when read. Instead, it checks if data has been modified when it is being saved.
This is typically performed by including a property on your model classes representing a row version (often a timestamp). When EF reads a record, it saves the current timestamp. When updating a record, it then checks that the timestamp in the database matches the one it saved. If they don’t match, a DbUpdateConcurrencyException
is thrown, signalling that the record was modified by another user.
What is Lazy Loading, and how does it interact with Entity Framework?
Answer
Lazy loading is a design pattern where entities are loaded only when they are actually needed. This can reduce initial data access times and memory usage, since entities not currently being used are not loaded into memory.
In the context of Entity Framework, lazy loading is achieved through virtual
navigation properties. When a property is marked as virtual
, the Entity Framework creates a proxy of the entity that overrides the property to add the loading behavior. Thus, the associated entities are only loaded from the database once the navigation property is accessed for the first time.
Loading code snippet...
What is the difference between Eager Loading and Lazy Loading in Entity Framework?
Answer
- Eager Loading: This is the process whereby a query for one type of entity also loads related entities as part of the query. Eager loading is achieved by use of the
Include
method. This means that when the query is executed, associated data is retrieved in the same query, thereby minimizing the number of queries to the database.
Loading code snippet...
- Lazy Loading: As stated earlier, lazy loading is the process whereby an entity or collection of entities is automatically loaded from the database when a property referring to the entity/entities is accessed for the first time. It helps in improving the application performance by avoiding unnecessary queries for related data.
Lazy loading is great for scenarios where you don’t need the related entities immediately, but it can result in more database queries overall if not used judiciously.
How does Entity Framework cater to the concept of Data Seeding?
Answer
Data seeding is the process of inserting default data into the database during the migration process. Entity Framework supports this by providing HasData
method.
HasData
method is used in the OnModelCreating
method of your DbContext
. You provide it with instances of your entity classes that represent the data you want to seed.
Loading code snippet...
The data you provide will be inserted into your database when you generate and run a migration. This is particularly useful for populating lookup tables, or providing a baseline set of data.
Fantastic progress through these C# Entity Framework interview questions so far!
We’ve covered how EF seamlessly caters to the concept of Data Seeding, enabling pre-population of database data. This leads us smoothly to our next ensemble of questions that unravel the interaction between EF and Stored Procedures.
It’s time to delve into how EF utilizes pre-prepared SQL command structures in the form of Stored Procedures and the utility of .tt files in EF.
Can you explain how to use Stored Procedures in Entity Framework?
Answer
In Entity Framework, you can use Stored Procedures by following these steps:
- Create the Stored Procedure in the database.
- Then, import the Stored Procedure into the Entity Framework model.
- After that, create a Function Import in the model, which will allow you to call the Stored Procedure from your code.
- Finally, use the Function Import in your C# code to execute the Stored Procedure.
This will look something like this in code:
Loading code snippet...
This example calls a Stored Procedure called “GetCourses”.
What’s the function of .tt files in Entity Framework?
Answer
The .tt files in Entity Framework are T4 template files. They are used for code generation by the Entity Framework. These template files are responsible for generating the C# or VB.Net classes that represent the entities (tables) in your database. This code generation occurs during compile-time, whenever you save changes to the entity data model (.edmx file) or manually run the “Run Custom Tool” command. If you need to customize the generated code, you can edit these .tt template files.
How does the Entity Framework work with LINQ to perform CRUD operations?
Answer
Entity Framework works with Language Integrated Query (LINQ) to simplify CRUD (Create, Read, Update, Delete) operations. This is how it works:
- Create: To create a new record, you create a new instance of the entity class and add it to the DbContext. Entity Framework will translate this into a SQL INSERT statement.
Loading code snippet...
- Read: LINQ queries are used to read data. Entity Framework will translate LINQ queries into SQL SELECT statements.
Loading code snippet...
- Update: To update a record, you retrieve an instance of the entity, make the changes to its properties, and then call SaveChanges on the DbContext. Entity Framework will generate a SQL UPDATE statement.
Loading code snippet...
- Delete: To delete a record, you retrieve an instance of the entity and call the Remove method on the DbSet.
Loading code snippet...
How to handle transactions in Entity Framework?
Answer
Entity Framework automatically handles transactions, starting a new one for each operation against the database (like calling SaveChanges()) and committing it when the operation ends.
However, if you want more granular control over transactions, you can use the Database.BeginTransaction()
method to manually start a transaction and the Commit()
or Rollback()
methods to end the transaction.
Below is a simple example:
Loading code snippet...
In the above example, if any exception occurs during the operation, the changes are rolled back and nothing is saved to the database.
How can you improve the performance of Entity Framework in an application?
Answer
Here are several ways you can improve the performance of Entity Framework in an application:
- Eager Load: If you know you will need related data for every entity retrieved, use eager loading to retrieve everything with a single database query.
- Use Compiled Queries: Compiling the query once and reusing the compiled version can improve performance for repetitive queries.
- Disable Change Tracking For Read-Only Operations: If you’re fetching data only for read purposes, no need for change tracking.
- Use Projections: Use projections to select only the specific columns you need.
- Batch Operations: If you need to insert, update, or delete a large number of records, consider using a library that supports batch operations, as Entity Framework processes each row individually.
- Turn off AutoDetectChanges: If you’re dealing with a lot of entities, consider turning off AutoDetectChanges during the read and manipulate phase and turn it back on at the point you are ready to persist the data.
- Indexing: Apply indexes on the columns that you often use in your queries, especially columns in WHERE, ORDER BY, JOIN conditions.
Remember, improving performance is a complex task, and it requires a thorough understanding of both the application and the Entity Framework. Always measure the performance before and after making changes, so you can accurately assess their impact.
We’ve reached the end of an immersive journey through a comprehensive list of EF Core interview questions, packed with practical guidance, code references, and expert tips.
From a simple definition of Entity Framework to performance enhancement techniques, this wide-ranging exploration should give you a solid understanding of the Entity Framework’s scope and remarkable capabilities.
Whether you’re a developer prepping for the big interview or just someone keen on learning more about this popular .NET technology, we hope we’ve helped you unlock new knowledge and confidence.
Keep exploring. Keep coding. The world of C# and Entity Framework continues to expand, and so should your expertise!