C# DateTime.Now vs DateTime.UtcNow: Differences and Use Cases
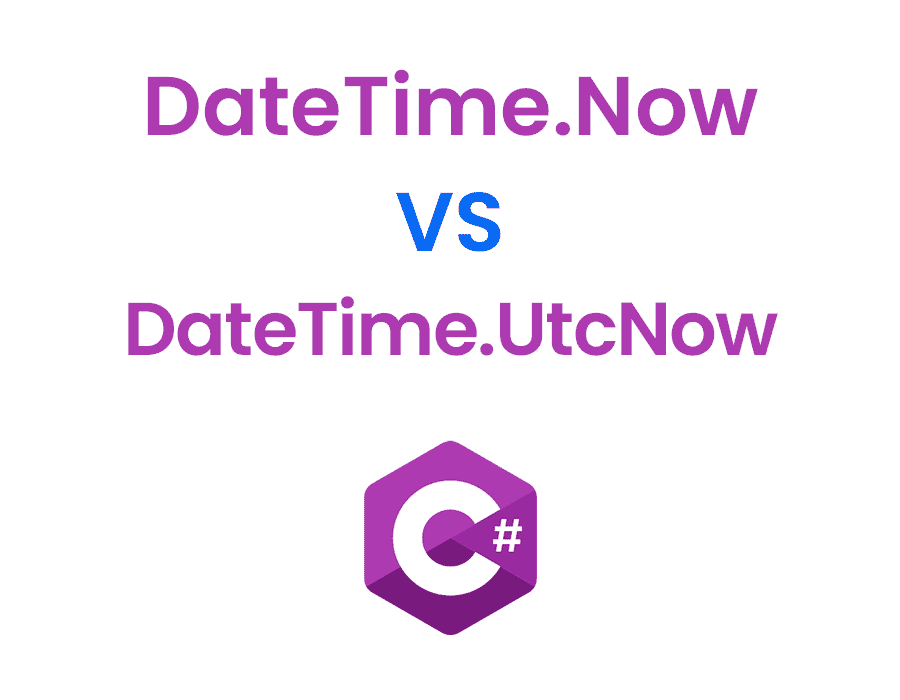
In this article, we are diving into the depths of DateTime in C#, focusing on the differences between DateTime Now and DateTime UtcNow. We’ll explore how to handle various aspects of time calculations, the power of UTC, and when you should use one or the other. Hang tight, as we embark on a thrilling journey through the world of date and time in C#!
Understanding DateTime Now in C#
Have you ever wondered how to work with the current time in your C# applications? DateTime Now is your go-to option for capturing the present moment. In this section, we’ll unwrap the concept of C# DateTime Now, discuss how it relates to time zones, and learn how to calculate time differences.
C# DateTime Now: Definition and Usage
In C#, DateTime.Now
returns the current date and time on the local system. This method accounts for the system’s time zone settings, which can be particularly useful when building applications that need to display or work with local times. Let’s have a look at an example:
Loading code snippet...
This simple code snippet captures the current local time and prints it to the console. Be mindful that changes in the system’s time zone will affect the output of DateTime.Now.
C# DateTime Formats and Time Zones
C# allows you to represent date and time values in various formats using ToString()
along with format specifiers. For instance, you can just display the date or time, or even select the long or short format versions. Check out these formatting examples:
Loading code snippet...
Unsure when to consider time zones? Picture a video conference app that has users across the globe. Coordinating a meeting time involves accounting for each user’s time zone, which makes DateTime.Now the perfect choice!
Calculating Time Differences with C# DateTime Now
Need to track time intervals or measure time elapsed? Math operations on DateTime objects come handy in making such calculations. The result is expressed in a TimeSpan
object. Here’s an example:
Loading code snippet...
In this example, we’re adopting the DateTime.Now property to determine the time elapsed while the thread sleeps for 2 seconds. The resulting time difference is then displayed in seconds.
Unraveling DateTime UtcNow in C#
While DateTime Now has its uses, there are certain scenarios where it might not be the best choice. Enter DateTime UtcNow! In this section, we’ll discuss the benefits of using UtcNow, how it works with time zones, and ways to convert between UTC and local time.
What is UtcNow? Understanding Coordinated Universal Time
Coordinated Universal Time (UTC) is the world standard for timekeeping, keeping everyone on “the same clock.” It’s immune to daylight savings changes and serves as the reference point for all local times and time zones. DateTime.UtcNow
helps developers access the current UTC DateTime.
C# DateTime UtcNow: Definition and Usage
DateTime.UtcNow
represents the current moment in Coordinated Universal Time. Being time-zone-agnostic, it’s handy when working across different time zones or when the application is intended for a global audience. Take a look at this example:
Loading code snippet...
In the code snippet above, we’re capturing the current UTC DateTime and printing it to the console.
Converting Between UTC and Local Time in C#
Working with users from different time zones often requires converting UTC time to local time (or vice versa). Fret not, as C# provides elegant methods to handle such transformations:
Loading code snippet...
In the example, we’re using ToUniversalTime()
to convert local time to UTC, and ToLocalTime()
for the reverse conversion. Voilà, timezone conversions made easy!
Handling UtcNow DateTime in Different Time Zones
What if your application needs to display time in a specific time zone? You can use the TimeZoneInfo
class to make the necessary conversions. Here’s a simple guide:
- Get the target time zone using
TimeZoneInfo.FindSystemTimeZoneById()
- Use
TimeZoneInfo.ConvertTimeFromUtc()
to convert UTC time to the desired time zone
Loading code snippet...
The code snippet above converts the current UTC time to Pacific Standard Time and displays it in the console.
Comparing DateTime Now and DateTime UtcNow in C#
As we venture further into our time-trek, we find ourselves at the crucial point where we must decide when to use DateTime Now vs DateTime UtcNow.
Each method has its distinct advantages depending on the application’s context, so let’s delve deeper into their various real-world use cases, complete with advanced code examples and thoughtful explanations.
When to Use DateTime Now
Let’s first examine situations where using DateTime.Now
would be the most suitable choice.
Interacting with User Interfaces
DateTime.Now
is ideal when displaying date and time to end-users within a user interface, as most users expect to see local times. Here’s an example that displays the current time in a user-friendly format:
Loading code snippet...
In this code snippet, we’re using DateTime.Now
to obtain the current local time, and the ToString method with format specifiers to display the time in a 12-hour format with AM/PM indicators.
Working with Local-Specific Data and Events
DateTime.Now
shines when managing data specific to local events, like appointments or store hours. Consider a clinic appointment scheduling app. For patients and staff, using local time would be more intuitive and less error-prone. Here’s an example:
Loading code snippet...
In this case, we create a local DateTime object for the appointment time and generate a reminder time by subtracting 48 hours. The output is displayed in the local time zone using the “f” format specifier.
When to Use DateTime UtcNow
Now, let’s explore situations where choosing DateTime.UtcNow
is the smarter choice.
Managing Time-Related Data for Global Applications
Applications that cater to a global audience, such as social media platforms, require a standard time format for storing and comparing data. DateTime.UtcNow
fits the bill perfectly. For instance, imagine storing the creation time of a post:
Loading code snippet...
Here, we take the current UTC time as the post creation time and display it. However, when showing the post creation time to users, you could then convert the stored UTC time to their local time zone.
Storing Timestamps in Databases
DateTime.UtcNow
is highly recommended for storing timestamps in databases, as it avoids potential discrepancies due to local time zone differences or daylight savings changes. Take a look at this example of a log entry:
Loading code snippet...
In this example, we’re capturing a log entry’s timestamp in UTC. When the stored timestamp needs to be presented, it can be converted back to the appropriate local time zone for easy readability and comprehension.
Performing Time-Related Calculations Across Multiple Time Zones
When you need to coordinate schedules or perform calculations across multiple time zones, working with UTC times can prevent potential errors. Imagine a project management tool that calculates deadlines in different time zones:
Loading code snippet...
In this example, we set a UTC deadline and calculate the corresponding deadlines in New York and London time zones using TimeZoneInfo
. This calculation illustrates the power of UTC when used in time zone conversion scenarios.
In conclusion, understanding the differences and use cases for C# DateTime Now and DateTime UtcNow is essential for accurate and efficient time calculations in your applications. By considering factors such as time zones and format requirements, you can make better decisions on which method to apply and avoid potential issues related to time managemen.
So, keep your clocks ticking and your apps in sync, and remember – time waits for no developer!