C# Design Patterns Interview Questions And Answers
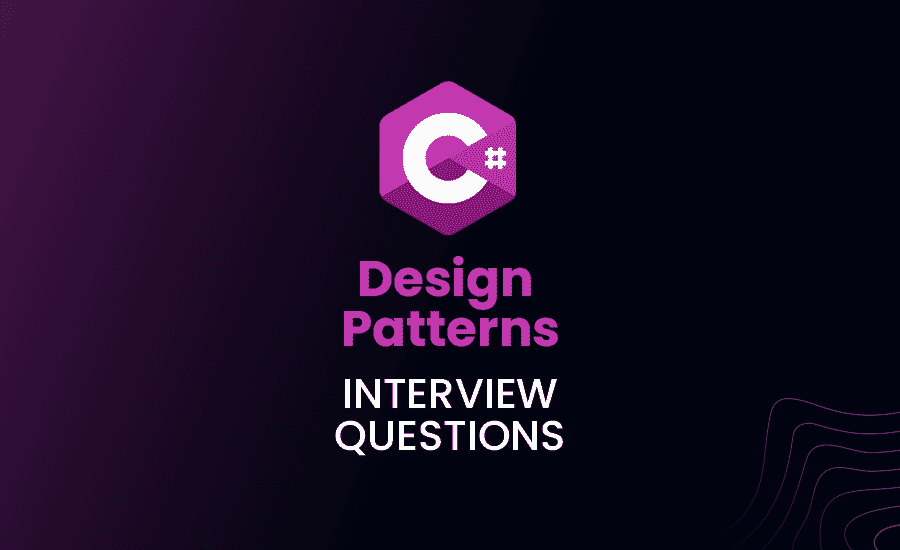
Are you preparing for a C# developer role and want to master your understanding of design patterns? You’ve come to the right place! In this article, we’ll explore some of the most common and challenging design patterns interview questions in C#.
From creational to structural and behavioral patterns, this comprehensive guide will give you insights into real-world scenarios and help you stand out in your interview. So, let’s dive right into these design patterns in C# scenario-based questions and become proficient in applying the principles of robust software architecture.
In the context of C# design patterns, can you discuss how the Abstract Factory pattern can be implemented using generics and reflection? Provide a code example to support your explanation.
Answer
The Abstract Factory pattern can be combined with generics and reflection to create a more flexible and dynamic implementation. It allows you to create instances of related classes without specifying their concrete types.
The basic idea is to create a generic abstract factory interface, with a method that takes a type parameter and returns an object of that type. The concrete factories then implement this interface and use reflection to create instances of the specified types.
Here is an example:
Loading code snippet...
Now you can create instances without specifying their concrete types:
Loading code snippet...
While this is a powerful technique, it also comes with some drawbacks:
- The use of reflection can have a negative impact on the performance.
- It relies on conventions, making the code harder to understand and maintain.
- Compile-time type checking is lost, increasing the possibility of runtime errors.
How can the Prototype design pattern be used in the C# language to optimize performance when dealing with complex object creation? What are potential scenarios where the Prototype pattern might be more beneficial than other creational patterns?
Answer
The Prototype design pattern can optimize performance when dealing with complex object creation by cloning existing objects instead of creating new ones from scratch. This can be particularly beneficial in situations when object construction is expensive or time-consuming, and you need to create multiple similar objects.
In C#, the Prototype pattern can be implemented using the ICloneable
interface, which defines a Clone
method that returns a shallow or deep copy of an object. Creating new objects through cloning can be faster than using constructors, especially when dealing with extensive object graphs or computationally expensive processes involved in initializing objects.
Some scenarios where the Prototype pattern can be more beneficial than other creational patterns:
- When creating an object is expensive or resource-intensive, and it would be more efficient to copy an existing object.
- When the system needs to create objects dynamically, and their types cannot be determined at compile time.
- When you want to create an object with the same state as a previous object, and the state initialization is complex.
Example:
Loading code snippet...
Explain how the State design pattern can be effectively used to manage different states within a complex application. Can you provide an example of its implementation in C# and discuss potential downsides of using this pattern?
Answer
The State design pattern is used to manage and represent different states of an object. It allows an object to change its behavior when its internal state changes. This pattern helps to keep the code more maintainable and organized by encapsulating state-specific behavior into separate classes and delegating functionality to the corresponding state objects.
Here’s an example of the State pattern implementation in C#:
Loading code snippet...
Potential downsides of using the State design pattern:
- Increased complexity due to the introduction of additional classes.
- State transitions may cause performance overhead if they happen frequently.
- Undefined behavior if states are created or modified outside of the central logic.
When implementing the Command design pattern in C#, what is the role of invoker, and how does it relate to the command and receiver components?
Answer
The Command design pattern is used to encapsulate requests or actions as objects, allowing the decoupling of the objects that initiate actions from the objects that actually perform them. The pattern consists of three components:
- Command: an interface or abstract class that defines the
Execute
method. - Receiver: the object that knows how to perform the requested action.
- Invoker: the object responsible for invoking the command’s
Execute
method.
In C#, the Invoker is typically a class that maintains a reference to a Command object and calls its Execute
method when a specific action needs to be performed. The Invoker is unaware of the underlying implementation details of the Command or how it interacts with the Receiver. This separation of concerns allows for greater flexibility, maintainability, and testability in the application.
Here’s an example of the Command pattern implementation in C#:
Loading code snippet...
Discuss the differences between the Decorator design pattern and the Proxy design pattern in the context of C#. Can you provide specific implementation examples and when each pattern should be applied?
Answer
The Decorator and Proxy design patterns are both structural patterns that involve wrapping an object with another object that has the same interface. However, they serve different purposes:
- Decorator is used to dynamically add or override responsibilities of an object without affecting its existing structure. Decorators are abstract classes or interfaces that inherit from the same base class or implement the same interface as the objects they wrap. They can be composed in any order and allow for flexible modifications to object behavior at runtime.
- Proxy is used to control access to the underlying object. Proxies can be used to add security checks, logging, caching, or other cross-cutting concerns. They are usually created by the client and have the same interface as the underlying object.
Decorator example in C#:
Loading code snippet...
Proxy example in C#:
Loading code snippet...
When to apply each pattern:
- Use Decorator when you need to dynamically add, remove, or modify the behavior of objects at runtime without affecting their existing structure.
- Use Proxy when you need to control, protect, or extend access to the underlying object, especially in cases where the cost of creating the original object is high.
As we’ve seen so far, design patterns play a significant role in simplifying complex problems and improving code maintainability.
Let’s continue our exploration of C# design questions with some fascinating examples, revealing how different patterns can optimize performance and memory usage by addressing real-world challenges.
Explain the concept of flyweight in C# and how it relates to the Flyweight design pattern. What is the significance of using this design pattern in C# applications, and what are potential obstacles developers should be aware of?
Answer
The Flyweight design pattern is used to minimize memory usage and improve performance when dealing with a large number of similar objects. The pattern promotes the sharing of common state between objects, called flyweights, to reduce memory consumption. Flyweights store intrinsic state (independent and shared among objects) while extrinsic state (dependent on and unique to each object) is passed through method parameters.
The concept of flyweight in C# refers to the implementation of this pattern, which often includes a flyweight factory responsible for managing flyweights and ensuring their sharing. The pattern is particularly useful in scenarios where the application creates a large number of objects that have common, sharable properties, reducing the memory footprint.
Here’s an example of the Flyweight pattern implementation in C#:
Loading code snippet...
Potential obstacles when using the Flyweight pattern:
- The need to manage extrinsic state separately from flyweight objects, which may increase overall complexity.
- The trade-off between memory savings and the increased cost of dealing with shared and unshared states.
- The potential difficulty in implementing the pattern correctly, especially when dealing with multithreading and synchronization.
In the C# language, how can the Facade design pattern be used to simplify interactions with complex subsystems? Provide an example of using the Facade pattern to hide complexity in a C# application.
Answer
The Facade design pattern is used to provide a simple, unified interface to a complex subsystem or group of subsystems with multiple interacting components. By abstracting the complexity of the subsystems behind the facade, it becomes easier to use and maintain the overall system.
Here is an example of using the Facade pattern in a C# application:
Loading code snippet...
The SubsystemFacade
class provides a SimpleOperation
method that hides the complexity of working with individual subsystems. Clients only interact with the facade, reducing the overall complexity and coupling between components.
Compare and contrast the Template Method design pattern with the Strategy design pattern in the C# programming language. When would you choose to use one pattern over the other, and how might they be combined in a C# application?
Answer
The Template Method and Strategy design patterns are both behavioral patterns that define a family of algorithms and their interactions. However, they achieve this goal in different ways:
- Template Method: This pattern defines the skeleton of an algorithm in a base class (or abstract class) but defers certain steps of the algorithm to subclasses. This lets subclasses redefine specific steps of the algorithm without altering the overall structure. The Template Method pattern uses inheritance as its basis.
- Strategy: This pattern defines a family of interchangeable algorithms by encapsulating them behind a common interface. The actual algorithm implementation can be replaced at runtime, allowing for flexibility in the choice of algorithm. The Strategy pattern uses composition as its basis.
When to choose one pattern over the other:
- Use the Template Method when you need to define a fixed sequence of steps in an algorithm with some steps that can be overridden by subclasses.
- Use the Strategy pattern when you want to define a set of interchangeable algorithms and allow the client to choose an algorithm at runtime.
The Template Method and Strategy patterns can be combined in a C# application when some parts of an algorithm can be extended or replaced at runtime, and other parts are fixed and can only be overridden. The Template Method pattern can define the fixed part of the algorithm, while the Strategy pattern can define the interchangeable parts.
Explain the benefits of using the Singleton design pattern in C#. How can thread-safety be ensured when implementing Singleton instances, and what are potential downsides of using Singletons?
Answer
The Singleton design pattern ensures that a class has only one instance and provides a global point of access to that instance. This pattern is useful when you need a single point of control, coordination, or shared state management in the application.
Benefits of using the Singleton pattern in C#:
- Ensures a single instance of a class, preventing multiple instances from conflicting with each other.
- Provides a global access point to the instance, making it easy to access shared resources or state.
- Controls life cycle of the instance, allowing for lazy instantiation or cleanup when the application terminates.
Thread-safety can be ensured when implementing Singleton instances by using the following techniques:
- Double-checked locking: By synchronizing access to the creation logic, ensuring that only one thread can enter the critical section at a time, you can prevent multiple instances from being created.
Loading code snippet...
- Static initialization: By leveraging C#’s static constructor feature, you can ensure thread-safety without manual synchronization. The .NET runtime guarantees the static constructor is called only once and in a thread-safe manner.
Loading code snippet...
Potential downsides of using Singletons:
- They can create high coupling between components since they’re globally accessible.
- They can make testing more difficult due to their global state and restricted instantiation.
- They may lead to unintended shared state or resources, causing issues in a multithreaded environment.
In the context of C# development, how does the Adapter design pattern help with integrating third-party libraries or legacy code? Provide an example of using the Adapter pattern to achieve compatibility between two incompatible interfaces.
Answer
The Adapter design pattern allows two incompatible interfaces to work together by creating a wrapper class that converts one interface to another. This pattern is particularly useful when integrating third-party libraries or working with legacy code that cannot be modified easily.
Here’s an example of using the Adapter pattern to achieve compatibility between two incompatible interfaces in C#:
Loading code snippet...
In this example, Adapter
implements the ITarget
interface and converts the Adaptee
‘s method GetSpecificRequest
into the ITarget
‘s method GetRequest
. By using the Adapter pattern, the client can now work with the Adaptee
class through the ITarget
interface, achieving compatibility between the two interfaces.
We hope these design patterns C# interview questions and answers have given you a clearer understanding of the applications and intricacies behind the most common patterns.
As we move towards the next set of design patterns scenario-based questions in C#, be prepared to learn even more about the rich world of software design and the power of these patterns in enhancing the development process.
Describe how the Observer design pattern can be used in C# for implementing event-driven systems. Can you provide a code example to illustrate the implementation of this pattern?
Answer
The Observer design pattern introduces a one-to-many dependency between objects, where one object (the “subject”), notifies all its dependents (the “observers”) about any state changes. This pattern is particularly useful in event-driven systems, as it promotes loose coupling between the notification source and the listeners.
C# has built-in support for the Observer pattern through events and delegates, simplifying the implementation of this pattern. Here’s an example in C#:
Loading code snippet...
In this example, Subject
class has a StateChanged
event that allows observers to subscribe to state updates. When the State
property is changed, the Subject
instance notifies all subscribed observers by invoking the StateChanged
event.
Explain the concept of Double Dispatch in C# and how it relates to the Visitor design pattern. What are some use cases where the Visitor pattern can be particularly beneficial in a C# application?
Answer
Double Dispatch is a technique that enables dynamic dispatch based on the types of two objects, rather than just one, by calling different methods in response to different combinations of object types. The Visitor design pattern is a way to implement the Double Dispatch concept in C#. The pattern allows for defining new operations on an object structure, without modifying the object structure or the data classes themselves.
In the Visitor pattern, we have:
- A hierarchy of element classes that define a method
Accept
taking a visitor as a parameter. - A visitor interface that defines methods for each type of element.
- One or more visitor classes that implement the visitor interface and define the behavior for each element.
The Visitor pattern is particularly beneficial in the following use cases:
- When you need to add new operations to an existing structure of objects without modifying their classes. This can be helpful if the object structure is part of a library or if the structure is stable and should not be changed.
- When you need to perform complex operations on an object hierarchy that requires interaction between multiple types within the hierarchy.
- When you want to separate concerns or keep some specific behaviors outside of the original object hierarchy classes.
Here’s a simple example of the Visitor pattern implementation in C#:
Loading code snippet...
How can using the Chain of Responsibility design pattern in C# improve modularity and extensibility in request handling? Provide an example of implementing this pattern and discuss potential challenges associated with its implementation.
Answer
The Chain of Responsibility design pattern allows you to decouple a sender of a request from its receivers, by allowing multiple handlers to process the request in a sequential chain. This creates a loose coupling between the sender and the receivers, making it easier to add, remove or reorder handlers in the chain. As a result, this pattern promotes modularity and extensibility in request handling.
Here is an example of implementing the Chain of Responsibility pattern in C#:
Loading code snippet...
In this example, we have two concrete handlers ConcreteHandlerA
and ConcreteHandlerB
. The requests are first handled by ConcreteHandlerB
, and if it cannot process them, they are passed to ConcreteHandlerA
. The handlers can be triggered by calling the HandleRequest
method on the first handler in the chain.
Potential challenges when implementing the Chain of Responsibility pattern:
- Finding the right balance between the number of handlers and the complexity of their behavior, to avoid creating an overly long and inefficient chain of calls.
- Ensuring that the chain has appropriate error handling or fallback mechanisms if no handler can process the request.
- Adding or removing handlers in the chain might require special considerations if handler dependencies need to be maintained.
Compare the Model-View-Controller (MVC) and Model-View-ViewModel (MVVM) design patterns in a C# application. What are the key advantages and disadvantages of each and when is one more suitable than the other?
Answer
Model-View-Controller (MVC) and Model-View-ViewModel (MVVM) are architectural design patterns used in C# applications to separate concerns and promote maintainability. They both consist of three main components but differ in their roles and interactions.
Model-View-Controller (MVC):
- Model: Represents the data and business logic.
- View: Represents the user interface and presentation of data.
- Controller: Acts as an intermediary between the Model and View, handling input, and updating the Model and View accordingly.
Advantages of MVC:
- Separation of concerns, which promotes maintainability and testability.
- Easy to understand and implement.
- Suitable for web applications, as it naturally fits the request-response nature of web applications.
Disadvantages of MVC:
- The controller may become complex and difficult to maintain if it consists of many responsibilities.
- The view susceptibility to code duplication, as views might share similar functionality.
Suitable for: web applications, where the request-response pattern is prevalent.
Model-View-ViewModel (MVVM):
- Model: Represents the data and business logic.
- View: Represents the user interface and presentation of data.
- ViewModel: An abstraction of the View that exposes data and commands for View to bind.
Advantages of MVVM:
- Encourages a clear separation of concerns, improving maintainability and testability.
- Supports two-way data binding, simplifying the synchronization between the View and ViewModel.
- Declarative nature of binding in XAML leads to cleaner, more readable code.
- ViewModel can be easily tested without dependencies on View or UI frameworks.
Disadvantages of MVVM:
- More complex to set up initially, especially when dealing with complex data binding or validation.
- It can be challenging to understand and implement for developers unfamiliar with the pattern.
Suitable for: WPF, Xamarin, and UWP applications, where strong data-binding capabilities are available.
When deciding between MVC and MVVM, consider the following criteria:
- For web applications, MVC should be the default choice, as it naturally fits the web’s request-response pattern.
- For WPF, Xamarin, or UWP desktop applications, MVVM is the preferred choice because of its strong data-binding support and suitability for UI-centric applications.
Can you provide a practical example of using the Mediator design pattern in a C# application? What problems does the Mediator pattern address and what are its potential downsides?
Answer
The Mediator design pattern promotes loose coupling between classes by encapsulating the interactions between these classes within a separate mediator object. The classes do not interact directly with each other, instead, they use the mediator to communicate.
Mediator pattern can be useful in situations where multiple components need to collaborate but should be decoupled from each other, like GUI components in a complex application.
Here’s an example of implementing the Mediator pattern in C#:
Loading code snippet...
In this example, ConcreteComponentA
and ConcreteComponentB
are part of a mediator’s object, ConcreteMediator
. They notify the mediator of any events or actions, and the mediator decides how to respond and trigger other components’ actions.
This pattern addresses the problem of tightly coupled classes that directly interact with each other, thereby improving maintainability and scalability.
Potential downsides of the Mediator pattern:
- The Mediator can become too complex, making it harder to maintain, as it consolidates all the interactions between related components.
- The Mediator’s implementation may become a bottleneck if not designed well, negatively affecting performance.
By now, you have acquired valuable knowledge to navigate the world of design patterns with confidence.
Our next set of C# design interview questions will delve into more complex scenarios, highlighting how patterns can facilitate communication between classes while promoting modularity and extensibility in your applications.
Discuss the concept of “inheritance versus composition” within the context of C# design patterns. When is it more appropriate to use inheritance, and when should composition be preferred?
Answer
Inheritance and composition are two ways to create complex objects by combining simpler ones in C#.
Inheritance is a mechanism by which one class extends another, inheriting its properties and methods. Inheritance is a “is-a” relationship, as the subclass is a specific type of the superclass.
Inheritance should be used when:
- The subclass is a more specific version of the superclass (e.g.,
Car
class extendingVehicle
class). - You want to reuse a superclass’s behavior and potentially override or extend it in the subclass.
- You want to ensure a consistent interface across derived classes.
- You want to take advantage of polymorphism, where methods can accept objects of the superclass and deal with the specific subclasses’ implementations.
Composition is a way of constructing complex objects by combining simpler objects, which are referenced within the composite object. Composition utilizes a “has-a” relationship, as the composite object contains other objects as parts.
Composition should be used when:
- The relationship between objects is not a “is-a” relationship.
- You want to create more flexible and maintainable relationships between objects, as components can be replaced or altered without affecting the composite object.
- You want to avoid the problems associated with inheritance, such as tight coupling or unintended side effects from inherited behaviors.
As a general rule, favor composition over inheritance, as it promotes more flexible and maintainable code. However, use inheritance when you have a clear “is-a” relationship or need the polymorphic behavior it offers.
How does the Iterator design pattern support iterating through a complex data structure in C#? Provide an example of implementing this pattern in a C# application.
Answer
The Iterator design pattern provides a way to access elements of a complex data structure sequentially without exposing its underlying implementation details. The pattern separates the iteration logic from the collection object, making it easy to add new collection types without changing the iteration code.
C# has built-in support for the Iterator pattern with the IEnumerable
and IEnumerator
interfaces. IEnumerable
provides a method to create an iterator object, while IEnumerator
defines methods to iterate through the data.
Here’s an example of implementing the Iterator pattern in C#:
Loading code snippet...
In this example, we have a SimpleCollection
class implementing the IEnumerable<T>
interface, and we provide a custom iterator class called SimpleCollectionIterator<U>
. The iterator class implements the IEnumerator<U>
interface, providing methods for iterating through the collection.
Using the Iterator pattern in C#, we can iterate through complex data structures without exposing their internal details and easily extend existing collections or add support for new data structures.
Can you discuss the role of context objects in the Interpreter design pattern in C# and provide an example of their usage in a C# application? What are some potential drawbacks of using this pattern?
Answer
In the Interpreter design pattern, a context object is used to store and manage the state or information that needs to be shared among the interpreter objects during the interpretation process. It provides a way to share information, variables, or configuration settings among interpreters, without having to pass this data explicitly at each step.
Here’s an example of implementing the Interpreter pattern in C#:
Loading code snippet...
In this example, we have a context object that encapsulates variables VariableX
and VariableY
. The AddExpression
and MultiplyExpression
classes implement the IExpression
interface and use the context to perform their calculations.
Potential drawbacks of using the Interpreter pattern:
- The code can become more complex and harder to maintain if the grammar rules that the interpreter needs to parse are very complicated.
- The pattern can have performance bottlenecks, as it usually relies on a recursive traversal of the abstract syntax tree, which can have a large memory footprint and slower execution time compared to other approaches like using lookup tables or regular expressions.
- The pattern is less suitable when there are existing libraries or tools that can be used to achieve the same result, like regular expressions, parsing libraries, or domain-specific languages.
Explain the benefits of applying the Memento design pattern in C# for managing the states of objects through snapshots. When should the Memento pattern be applied and what are its potential limitations?
Answer
The Memento design pattern allows an object, known as the originator, to save its internal state and restore it at a later point. This is achieved by creating a memento object that stores the state snapshot, without exposing the originator’s internal representation. The pattern is useful when you need to provide undo or rollback functionality or track an object’s state history.
Benefits of the Memento pattern:
- Encapsulates the state and restoration logic, without exposing the originator’s internal structure.
- Maintains the encapsulation of the originator by storing the state in a separate memento object.
- Provides an easy way to undo changes or restore the previous state when needed.
When to apply the Memento pattern:
- Implement undo/redo functionality in applications like text editors or drawing programs.
- Save the state of the application for later restoration, like in games or simulation software.
- Track the history of internal object states in cases where observing the state history is important.
Here’s an example of implementing the Memento pattern in C#:
Loading code snippet...
In this example, we have an Originator
class that can save and restore its state using Memento
objects. A Caretaker
class manages the mementos and is responsible for saving and restoring states.
Potential limitations of the Memento pattern:
- Storing a large number of snapshots might consume significant memory or disk resources.
- Saving and restoring the state of an object can have performance consequences when dealing with large or complex objects.
- Mementos might become invalidated when the originator’s internal structure changes, potentially leading to errors or data loss during state restoration.
How can the Bridge design pattern be helpful in avoiding the cartesian product complexity issue when combining abstraction and implementation in a C# application? Can you provide an example of implementing the Bridge pattern and explain its major components?
Answer
The Bridge design pattern decouples an abstraction from its implementation, allowing the two to evolve independently. This pattern can be helpful in avoiding the cartesian product complexity issue when combining abstraction and implementation.
The cartesian product complexity issue arises when the number of concrete classes explodes due to the multiple combinations of possible abstractions and their implementations. With the Bridge pattern, you can separate the hierarchies of abstraction and implementation and reduce the complexity when extending them.
The major components of the Bridge pattern are:
- Abstraction: It defines the interface for the abstraction and maintains a reference to the implementation.
- Concrete Abstraction: It extends the Abstraction class and represents specialized abstractions.
- Implementor: It defines the interface for the implementation.
- Concrete Implementor: It provides the concrete implementation of the Implementor interface.
Here’s an example of implementing the Bridge pattern in C#:
Loading code snippet...
In this example, we have an abstract RemoteControl
class representing the abstraction, and a BasicRemoteControl
as its concrete implementation. The IDevice
interface acts as the Implementor, while the Television
and Radio
classes serve as concrete implementors. The Bridge pattern is applied by having the RemoteControl
class reference an IDevice
, allowing it to interact with different device types, while keeping the device implementations independent.
We hope this comprehensive guide on design patterns interview questions in C# has helped you expand your knowledge, improve your software architecture skills, and better prepare for your upcoming interviews. The scenarios and patterns discussed in this article are just a glimpse of the vast domain of design principles in the programming world.
Remember, mastering design patterns takes practice and continuous learning, and we encourage you to explore even more resources. With determination and a clear understanding of these C# design questions, you are one step closer to acing that interview and landing your dream job as a C# developer.
Good luck!