C# Array Interview Questions and Anwsers
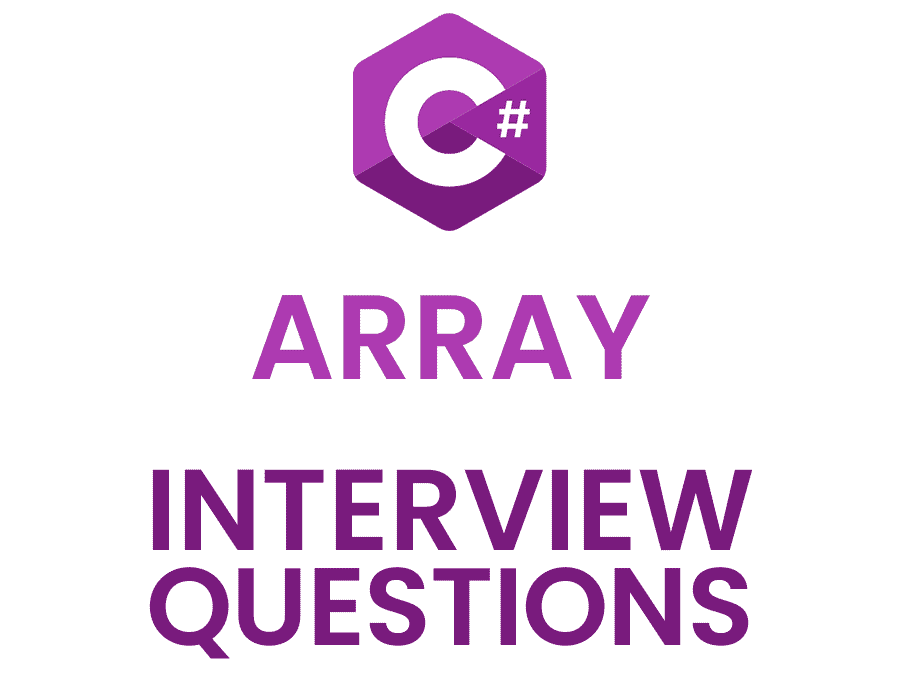
Are you preparing for a C# developer interview or simply looking to augment your knowledge of arrays in C#? No matter your motivation, this article will prove invaluable as we explore some of the most frequently asked and advanced array C# interview questions.
We’ll dive deep into the intricacies of multi-dimensional arrays, optimizing performance, memory management, and more. Whether you’re searching for array interview questions in C#, trying to wrap your head around array program in C# for interviews, or seeking array programming interview questions in C# .NET, this comprehensive guide has you covered.
In a multi-dimensional array, how does the principle of locality of reference play a role in the speed of array traversals, and how can you optimize your code accordingly for better performance in C#?
Answer
The principle of locality of reference comes into play when dealing with large data structures, like multi-dimensional arrays, in which consecutive elements are accessed sequentially or near each other. When elements are close together in memory, performance is optimized as caches frequently store contiguous memory blocks. To make the best use of CPU cache lines, it’s essential to traverse and access the array elements in the order they are stored in memory.
In C#, multi-dimensional arrays store elements in row-major order: that is, elements of the same row are stored in contiguous memory. To optimize your code for better performance, ensure that you traverse the array following the row-major order. To achieve this, nest your loops with the outer loop iterating through rows and the inner loop iterating through columns.
For example, suppose you have a 2D array with dimensions M x N. To optimize traversal, follow this pattern:
Loading code snippet...
By following the row-major order, you reduce cache misses and improve overall performance.
Explain the difference between Array.Resize() and Array.Recreate() in C#, providing example use-cases for when each would be more appropriate in terms of performance and memory management.
Answer
In C#, there is no built-in method called Array.Recreate()
. Instead, resizing an array can be done using the Array.Resize()
method.
Array.Resize()
is used to change the size of an existing array, creating a new array in the process if necessary. When resizing is needed, the method creates a new array with the specified size and copies the elements from the original array to the new one. This can impact performance and memory usage, especially when working with large arrays.
Here’s an example of using Array.Resize()
:
Loading code snippet...
If you meant Array.CreateInstance()
instead of Array.Recreate()
, then this method creates a new array with a specified type and dimensions. It does not copy any data from an existing array. You should use Array.CreateInstance()
when you want to create a new array from scratch, or if you need to create an array with a specific runtime type.
Here’s an example of using Array.CreateInstance()
:
Loading code snippet...
In terms of performance and memory management:
- Use
Array.Resize()
when you have an existing array that needs resizing, and you want C# to handle the copying of elements. This is suitable when the array resizing is infrequent, and the cost of copying elements is not a critical concern. - Use
Array.CreateInstance()
when you need to create a new array with a specific type at runtime or when creating a new array without copying any data.
In C#, how does the garbage collector treat arrays with a large number of elements, and how can you avoid potential issues with garbage collection when working with large arrays?
Answer
In C#, the garbage collector (GC) manages memory by checking for objects that are no longer in use and deallocating their memory. When working with large arrays, a few issues related to garbage collection could arise:
- Memory pressure: Large arrays can cause memory pressure, leading to more frequent garbage collection cycles. These cycles can impact the application’s performance.
- Large object heap (LOH) allocations: Arrays larger than 85,000 bytes are allocated on the LOH (Large Object Heap). The LOH is collected less frequently than the rest of the heap, which can cause memory fragmentation and increased memory usage.
To avoid these potential issues with garbage collection when working with large arrays:
- Use smaller arrays if possible, as this can reduce the memory pressure and lead to fewer garbage collection cycles.
- When resizing large arrays, consider using an alternative data structure, like a
List<T>
, which has built-in resizing and avoids repeated copying. However, it may still store the underlying array in the LOH. - Optimize your application’s memory usage patterns by reducing the frequency of large array allocations and deallocations. For example, reuse large arrays or use object pooling.
- Consider using
ArrayPool<T>
from theSystem.Buffers
namespace, which provides a pooled memory resource for arrays, reducing memory allocations and LOH usage. - If it’s appropriate for your problem, consider using memory-mapped files to perform I/O operations instead of loading large files into memory using arrays.
By following these guidelines, you can minimize the impact of garbage collection on large arrays and improve the overall performance of your application.
What are the primary differences between IEnumerable and IQueryable extension methods when working with arrays in C#? Provide a code example showing the implications of choosing one over the other.
Answer
IEnumerable
and IQueryable
are both interfaces in C# that are primarily used for querying data from collections. Although they share some similarities, they have different behaviors and use cases:
IEnumerable
: This interface represents a collection of elements that can be iterated. It works on in-memory collections like arrays, lists, and other objects that implementIEnumerable<T>
. When using LINQ extension methods withIEnumerable
, the query is executed in-memory, and the data is fetched before the query is executed. This can lead to the full collection being loaded into memory, causing performance issues when dealing with large datasets.IQueryable
: This interface is a specialization ofIEnumerable
that is designed to work with out-of-memory collections, like remote databases. It allows you to define a query on the collection, but the query isn’t executed until the data is enumerated (deferred execution). Furthermore, the query generated byIQueryable
can be converted into a native query language (e.g., SQL) and can be executed remotely, reducing the data fetched to only the required subset.
Here is a code example that demonstrates the implications of using IEnumerable
and IQueryable
:
Loading code snippet...
In this example:
- When using
IEnumerable
, all products are fetched from the database and loaded into memory asproductsInMemory
. Then, the query is executed in memory to filter expensive products. This can cause performance issues when dealing with large datasets, as all data is loaded into memory. - When using
IQueryable
, the query is not executed until callingToList()
. This means that the filtering is done on the database side, and only the expensive products are fetched and loaded into memory, improving performance.
When working with arrays and in-memory collections, using IEnumerable
is generally the appropriate choice. Use IQueryable
when working with out-of-memory collections, such as remote databases, to benefit from deferred execution and remote query execution.
Describe how C# accommodates Covariant Arrays despite the CLR not supporting them. What potential issues might arise while using Covariant Arrays, and how can they be avoided?
Answer
Covariant arrays in C# allow you to assign an array of a derived type to an array of a base type. Although the CLR (Common Language Runtime) doesn’t directly support covariant arrays, C# implements this feature through runtime checks.
When you try to perform an array assignment that has a reference type element and the assignment is supposed to be covariant, C# introduces a runtime check to validate the type compatibility between the source and target arrays. If the types are compatible, the assignment is allowed; otherwise, an ArrayTypeMismatchException
is thrown at runtime.
Consider the following example:
Loading code snippet...
In this example, the assignment of a Derived[]
to a Base[]
is allowed due to covariance. However, when attempting to assign a new Base()
to the array at index 0, a runtime check will be performed, which then results in an ArrayTypeMismatchException
.
One potential issue with covariant arrays is that they may introduce a performance penalty due to the runtime type checking. Additionally, there’s a risk of runtime exceptions if incompatible types are assigned.
To avoid these issues, consider using the following alternatives:
- Generics: Use generic collections like
List<T>
orIEnumerable<T>
instead of arrays, leveraging generic covariance and contravariance features available in C#. - Explicit type casting: When working with arrays and you’re sure of the type compatibility, use explicit type casting to avoid runtime type checking and potential exceptions.
By using these alternatives, you can bypass potential issues and improve the safety and performance of your code.
Now that we have delved into the intriguing world of Covariant Arrays, let’s transition our focus towards another exciting enhancement introduced in C# 8.0: Array Slicing.
This powerful feature offers new possibilities for processing arrays, so let’s unravel its advantages and potential risks by analyzing an example use-case.
Explain the concept of Array Slicing in C# 8.0, providing an example use-case and highlighting its advantages and potential risks.
Answer
Array slicing is a feature introduced in C# 8.0 that allows you to access a range of elements from an existing array or other indexable data structures, such as Span<T>
or ReadOnlyMemory<T>
, without creating a new array. It leverages the Range
and Index
types and the ..
operator to specify the start and end indices of the slice.
Here’s an example of array slicing:
Loading code snippet...
Advantages of array slicing:
- Performance: Slicing doesn’t create a new array, reducing the memory overhead and improving performance. This is especially helpful when working with large data structures.
- Readability: The slicing syntax is concise and easy to read.
- Flexibility: Slicing can be used with other types that support indexing, such as
Span<T>
orReadOnlyMemory<T>
.
Potential risks of array slicing:
- Shared data: Since slicing doesn’t create a new array, any changes made to the slice will also affect the original array, which may lead to unintended side effects.
To mitigate the potential risks of shared data, consider the following:
- When working with mutable data, you can create a copy of the slice if required to avoid sharing data with the original array.
- Use
ReadOnlyMemory<T>
orReadOnlySpan<T>
when you need to provide read-only access to a slice of data, preventing modification of the original data.
Describe the role of Array Pools in C# and .NET Core. How do they help in optimizing performance and memory usage when working with large arrays, and provide an example of how to implement Array Pooling in your code.
Answer
Array Pools in C# and .NET Core are designed to help optimize performance and memory usage when working with large arrays by reusing memory that has already been allocated, instead of creating new arrays each time they are needed. Array Pools can significantly improve the performance of your application, especially in scenarios where you repeatedly allocate and deallocate arrays.
The .NET Core System.Buffers
namespace provides the ArrayPool<T>
class for managing a shared pool of arrays. Here’s how Array Pools can help optimize performance and memory usage:
- Reuse of memory: Array Pools allow you to reuse previously allocated memory, which can help reduce memory pressure and garbage collection cycles.
- Reduced allocations: By reusing arrays from the pool, you reduce the need to allocate new memory, which can improve performance.
Here’s an example of using Array Pooling in your code:
Loading code snippet...
When implementing Array Pooling, ensure that the rented arrays are returned to the pool after use. Failing to return rented arrays can lead to memory leaks.
Discuss the concept of Array Segments in C#. Explain how they are different from a traditional subarray approach, provide an example of their usage, and describe the advantages and disadvantages of using Array Segments.
Answer
Array Segments in C# represent a portion of an existing array and give you the ability to work with a range of elements without copying the data or creating a new array. ArraySegment<T>
is a struct that encapsulates an underlying array along with an offset and length.
Comparing Array Segments with traditional subarray approaches:
- Memory efficiency: Unlike traditional subarray approaches, where creating a subarray requires copying data into a new array, Array Segments do not need additional memory or copying, as they only reference the existing array.
- Performance: Array Segments can provide better performance than traditional subarrays since they do not require additional memory allocations or copying operations.
- Updated data: Since the Array Segment refers to the original array, any modifications made to the Array Segment are reflected in the original array.
Here’s an example of using an Array Segment:
Loading code snippet...
Advantages of using Array Segments:
- Improved memory efficiency and performance, as no copying operations or additional memory allocations are needed.
- Simplicity and ease of use when working with portions of arrays, particularly in APIs where you only need a range of elements from an array.
Disadvantages of using Array Segments:
- If you need to make modifications to a portion of an array without affecting the original array, Array Segments are not suitable.
When working with a multi-dimensional array in C#, how can you minimize the possibility of cache misses and improve performance using cache-friendly traversals or storage methods?
Answer
To minimize the possibility of cache misses and improve performance when working with multi-dimensional arrays in C#, keep the following points in mind:
- Follow row-major order: Since C# stores multi-dimensional arrays in row-major order, you should traverse the array in a way that respects this order. Access elements in nested loops with the outer loop iterating through rows and the inner loop iterating through columns.
Loading code snippet...
- Cache-friendly data structures: Consider using alternatives to multi-dimensional arrays that provide better cache locality, such as jagged arrays or flattened 1D arrays.
- Jagged Arrays: Less memory is wasted when working with non-rectangular data, and each inner array can be accessed sequentially in memory.
Loading code snippet...
- Flattened 1D array: Use a 1D array and compute the index using the row and column information.
Loading code snippet...
- Block-based techniques: Divide the array into smaller blocks that can fit into the cache and process these blocks sequentially. By processing smaller blocks, you can keep the data required for any computation in cache, reducing cache misses and improving performance.
By using cache-friendly traversals, alternative data structures, and block-based techniques, you can reduce cache misses and improve the performance of your code when working with multi-dimensional arrays.
What is the effect of boxing and unboxing operations on the performance and memory usage when dealing with value-type arrays in C#? How can you mitigate these overheads with alternative approaches?
Answer
Boxing and unboxing have performance implications when working with value-type arrays in C#. Boxing is the process of converting a value type to an object type (essentially wrapping it into a reference type). Unboxing is converting that object back into a value type.
Both operations have the following effects:
- Performance: Boxing and unboxing operations introduce runtime overhead since the value needs to be converted to an object and back. This can significantly slow down the execution when dealing with large value-type arrays or when boxing/unboxing is done repeatedly.
- Memory: Boxing requires additional memory to be allocated for the object instances that are created. This additional memory usage might lead to higher pressure on the Garbage Collector, which can further decrease overall performance.
To mitigate these overheads, consider the following alternative approaches:
- Generics: Use generic collections like
List<T>
andDictionary<TKey, TValue>
instead of non-generic collections likeArrayList
orHashTable
. Generic collections avoid boxing/unboxing because they take value types directly without converting them to objects.
Loading code snippet...
- ValueTuple: When creating custom structures, use
ValueTuple
instead ofTuple
to create value-type tuples that avoid boxing/unboxing overheads.
Loading code snippet...
- Avoid mixing value types and object types: Design interfaces and classes so that value types don’t need to be boxed.
As we have examined the impact of boxing and unboxing operations on value-type arrays, it is essential to consider how parallel techniques in C# can be employed to sort and search large arrays efficiently in a multi-threaded environment.
In the ensuing sections, we will elucidate this concept with practical examples demonstrating varying approaches.
In C#, how can you effectively sort and search large arrays in a multi-threaded environment using parallel methods? Provide examples demonstrating your approach.
Answer
To effectively sort and search large arrays in C# using parallel methods, you can use the System.Linq.Parallel
namespace, also known as PLINQ. PLINQ enables you to parallelize most LINQ queries just by adding an .AsParallel()
call to your query, allowing you to take advantage of multiple processor cores.
Below is an example of a parallel sort and search on an array of integers:
Loading code snippet...
Remember, PLINQ parallelism introduces overhead, so it’s best suited for scenarios where it provides an actual performance boost. Always measure and compare the performance when using parallel methods to ensure that the benefits outweigh the additional overheads.
With the introduction of Span in C# 7.2, how does it improve working with arrays over traditional methods? Discuss the memory implications and potential use-cases where Span could provide a significant advantage.
Answer
Span<T>
is a new type introduced in C# 7.2 that provides a way to work with contiguous blocks of memory in a safe and efficient manner. Here are some improvements it offers over traditional methods when working with arrays:
- Avoid memory allocations:
Span<T>
enables you to create slices of an existing array without copying the underlying data, avoiding the additional memory allocations and their associated overheads. - Reduced garbage: Because the array slices created with
Span<T>
do not result in additional memory allocations, the garbage collector has less work to do, which reduces GC pressure. - Performance: Since
Span<T>
avoids memory allocations and copies, it can provide better performance when working with large arrays or in resource-constrained environments. - Safety:
Span<T>
provides bounds checks and is memory-safe, similarly to traditional arrays. - Flexibility:
Span<T>
can work with various data sources, including managed arrays, unmanaged memory, and stack-allocated memory.
A common use-case for Span<T>
is parsing and processing strings or binary data, where it provides significant advantages by eliminating memory allocations while keeping the code safe.
Loading code snippet...
Explain how you can implement custom iterators when working with arrays in C#, detailing the considerations that need to be made for performance and memory management.
Answer
To implement custom iterators when working with arrays in C#, you can create a custom collection class that implements IEnumerable<T>
and IEnumerator<T>
. The key performance and memory management considerations are:
- Avoid unnecessary allocations and copies: When implementing the iterator, be mindful of creating new objects or copying data if it can be avoided.
- Use value types where applicable: If your custom iterator can be represented as a value type (
struct
), prefer this over reference types to avoid memory allocations and lower GC pressure. - Consider using
yield return
: You can use theyield return
statement to provide a simplified iterator implementation if it’s suitable for your scenario. However, be mindful thatyield return
can introduce additional overheads in certain situations.
Here’s an example of a custom iterator for an array of integers:
Loading code snippet...
Usage:
Loading code snippet...
When should you use Array.ConvertAll in C# instead of a simple for loop or Array.ForEach? Demonstrate how to use Array.ConvertAll with a custom conversion function that returns a different output type.
Answer
Array.ConvertAll
should be used when you need to:
- Transform an input array into an output array of a different type, applying a conversion function on each element of the input array.
- Keep the input array unchanged, creating a new array with the converted elements.
- Write concise code, skipping explicit loops that can be error-prone.
Compared to Array.ForEach
, Array.ConvertAll
returns a new array with the results of the conversion function applied to each input array element. Array.ForEach
only performs an action on each array element but doesn’t return a new array.
Here’s an example of using Array.ConvertAll
with a custom conversion function that converts an array of integers into an array of strings:
Loading code snippet...
How can you implement a custom, high-performance, generic array-based data structure in C# that supports dynamic resizing, without using built-in lists or arrays? Provide a code example demonstrating your solution.
Answer
To implement a custom, high-performance, generic array-based data structure without using built-in lists or arrays, you can utilize an underlying buffer (like a raw memory block or an array) that grows and shrinks dynamically. Here is an example of such a data structure named DynamicArray<T>
:
Loading code snippet...
Usage example:
Loading code snippet...
Having outlined how to implement an effective, custom array-based data structure, it is worth exploring the implications of using fixed-size buffers in C# when working with arrays. In the following section, we will scrutinize their impact on performance and highlight ways to use them to significant effect.
What are the implications of using fixed-size buffers in C# when working with arrays? Explain how they can be used to improve performance and provide a code example showing how to declare and use a fixed-size buffer.
Answer
Using fixed-size buffers in C# can have several implications on performance when working with arrays:
- Memory allocation: Fixed-size buffers are allocated on the stack instead of the heap, reducing heap memory usage and avoiding garbage collection overhead.
- Performance: Stack memory access is generally faster than heap memory access, which can lead to improved performance when working with fixed-size buffers.
Keep in mind that using fixed-size buffers comes with constraints:
- You can only use them inside
unsafe
contexts. - They must be placed within a
struct
. - Fixed-size buffers always have a fixed element type, like
char
orint
.
To declare and use a fixed-size buffer, follow these steps:
- Define a
struct
containing the fixed-size buffer field marked with thefixed
keyword. - Use the
unsafe
keyword to indicate that you’re working inside an unsafe context.
Example of declaring and using a fixed-size buffer of integers:
Loading code snippet...
Rendering of certain elements may not be correct in Jupyter Notebook Markdown.
Explain different methods for checking if an array in C# is sorted under certain conditions, such as ascending or descending order, or if each element is unique. Provide code examples with varying levels of efficiency and explain their trade-offs.
Answer
There are several approaches to check if an array is sorted in various conditions:
- Simple iterative approach: Traverse the array and check if the elements are sorted in the desired order (ascending or descending). This method takes O(n) time complexity where n is the number of elements.
Loading code snippet...
- Use LINQ extension methods: Use methods like
Zip
,All
, andSequenceEqual
to concisely check for sorted order. These methods are easy to read but can introduce execution overheads compared to a simple loop.
Loading code snippet...
- Checking for unique elements: When checking for unique elements, you can use a
HashSet
to track the occurrence of each element in the array while traversing it. This approach has an additional O(n) space overhead but maintains time complexity of O(n) where n is the number of elements.
Loading code snippet...
Trade-offs between these methods include:
- Readability vs. Performance: Simple loops are less expressive than LINQ methods but execute faster.
- Time Complexity vs. Space Complexity: Checking for unique elements using a HashSet requires additional memory, but is more efficient than alternative methods like nested loops (O(n^2) time complexity).
How can you create efficient run-length encoding/decoding algorithms using C# arrays? Provide a code example of both an encoding and decoding function, and discuss their performance characteristics.
Answer
Run-length encoding (RLE) is a simple data compression algorithm that condenses data by replacing consecutive, identical elements (runs) with a single element and an integer representing the length of the run.
Here’s an example of an encoding and decoding function for run-length encoding using C# arrays:
Loading code snippet...
Performance characteristics:
- Encoding: The encoding function has a time complexity of O(n), where n is the length of the input array. It iterates through the input array once while appending characters and counts to the result StringBuilder.
- Decoding: The decoding function has a time complexity of O(n), where n is the length of the encoded string. The function iterates through the input string once, decoding runs and adding them to a List that’s then converted to an array.
The space complexities for both functions are also O(n), as they store intermediate results in data structures that grow proportionally to the input size.
Expound on how to efficiently perform matrix operations, such as addition, subtraction, and multiplication, in C# using multi-dimensional arrays. Include code examples showcasing your approach and discuss optimization techniques for these operations.
Answer
Matrix operations can be performed efficiently using multi-dimensional arrays in C#. Here’s an example of functions for performing addition, subtraction, and multiplication on matrices:
Loading code snippet...
Optimization techniques:
- Cache-friendly traversals: Iterating through matrices in a row-major order, with inner loops scanning rows and outer loops scanning columns, can improve cache locality and performance when accessing elements in multi-dimensional arrays.
- Parallelization: Matrix operations can be parallelized efficiently using techniques like parallel loops or task-based parallelism, depending on the problem size and hardware capabilities, enabling faster execution on multi-core processors.
- SIMD: Exploit SIMD operations for matrix arithmetic, if available, to perform multiple operations using a single instruction, resulting in improved performance.
How do SIMD (Single Instruction Multiple Data) operations provided by System.Runtime.Intrinsics namespace improve performance when working with large arrays in C#? Give an example of utilizing SIMD operations in a computationally-intensive array manipulation algorithm.
Answer
SIMD (Single Instruction Multiple Data) allows performing the same operation on multiple data elements concurrently, which can greatly improve performance when dealing with large arrays and computationally intensive algorithms.
In C#, SIMD operations are available in the System.Runtime.Intrinsics namespace, which provides various types representing SIMD registers and operations on supported architectures (e.g., AVX, SSE, and ARM Neon).
Here’s an example of using SIMD operations to add two large float arrays:
Loading code snippet...
In this example, we perform SIMD addition on chunks of the input arrays using AVX operations when supported by the architecture, falling back to scalar addition for any remaining elements.
SIMD operations can significantly improve performance, especially in computationally-intensive algorithms that process large arrays. However, keep in mind that SIMD support might vary across architectures, and properly written fallback code is necessary to ensure compatibility.
Throughout this article, we dissected an array of topics, ranging from array programs in C# for interviews to general array-related concepts, equipping you with the confidence to tackle any array programming challenge. With this newfound knowledge of key array interview questions in C#, you’ll be well-prepared to impress potential employers or enhance your professional skills further.
As you advance in your career as a C# developer, remember that a solid understanding of the versatile and performant array data structure is essential to writing robust and efficient C# code, and practising complex array operations will enable you to produce solutions that stand out in the competitive tech landscape.