Clean Architecture in Xamarin
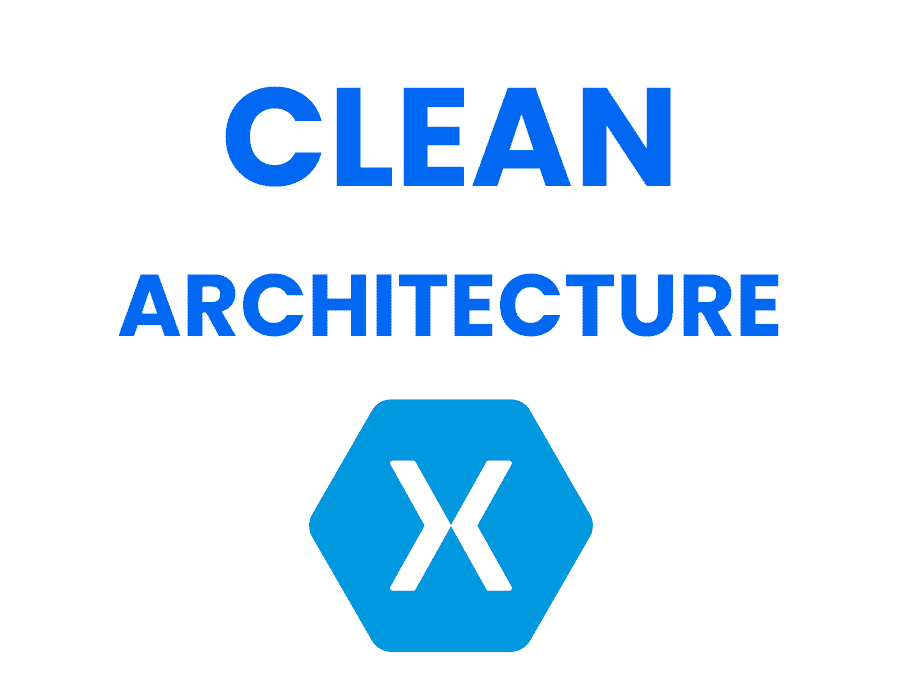
Introduction to Clean Architecture
Clean architecture is an approach to software development that promotes the separation of concerns, maintainability, and testability. It aims to create a scalable and easily understandable system by dividing it into various layers. Each layer has its own set of responsibilities and dependencies, allowing developers to focus on specific parts of the application without affecting others.
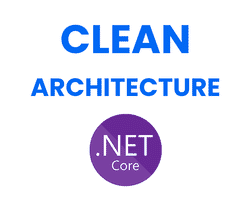
Clean Architecture in .NET Core
February 5, 2023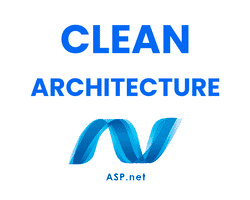
Clean Architecture in ASP.NET
January 15, 2023Benefits of Clean Architecture
Some of the key benefits of implementing clean architecture in your projects are:
- Improved maintainability: By separating concerns and defining clear boundaries, the codebase becomes more manageable and easier to update.
- Enhanced testability: Each layer can be tested independently, allowing for more accurate and efficient testing.
- Scalability: Clean architecture allows you to easily scale your application as it grows in complexity.
- Easier collaboration: With a well-defined structure, developers can work on different parts of the application without interfering with each other’s work.
Xamarin: A Brief Overview
Xamarin is a popular cross-platform app development framework that allows developers to build native applications for Android, iOS, and Windows using a single, shared codebase. It leverages the power of .NET and C# to enable developers to create high-performance, user-friendly apps that can access platform-specific features.
Implementing Clean Architecture in Xamarin
In this section, we’ll explore how to implement clean architecture in a Xamarin application. We’ll cover the solution structure, dependency injection, data binding, and unit testing.
Solution Structure
A typical clean architecture solution in Xamarin consists of four layers:
Domain Layer
This layer contains the business logic and domain entities. It defines the core of the application and should have no dependencies on other layers. The domain layer typically includes interfaces for repositories, entities, value objects, and domain events.
Application Layer
The application layer is responsible for coordinating the domain layer with the infrastructure and presentation layers. It contains use cases, view models, and services that translate between the domain and the other layers. This layer should have no direct dependencies on the presentation or infrastructure layers.
Infrastructure Layer
This layer provides implementations for the interfaces defined in the domain layer. It is responsible for handling data access, networking, and other platform-specific tasks. The infrastructure layer should only depend on the domain layer.
Presentation Layer
The presentation layer is responsible for displaying the user interface and handling user interactions. It should be as thin as possible, focusing on presenting data from the application layer and passing user input back to it. This layer should only depend on the application layer.
Dependency Injection
Dependency injection (DI) is a technique for managing dependencies between objects in a clean and decoupled manner. In Xamarin, you can use DI frameworks like Autofac or Microsoft.Extensions.DependencyInjection to manage your dependencies across the different layers. This enables you to easily swap implementations and make your code more testable.
Data Binding
Data binding is an essential aspect of Xamarin applications, allowing you to bind properties of UI elements to properties of view models. This enables seamless communication between the presentation layer and the application layer. Xamarin.Forms provides a robust data-binding system that supports one-way and two-way data binding, as well as binding to commands for handling user interactions. By using data binding, you can maintain a clear separation of concerns and ensure that your presentation layer remains decoupled from the underlying layers.
Unit Testing
Unit testing is crucial for ensuring the stability and quality of your application. In a clean architecture solution, each layer can be tested independently, making it easier to identify and fix issues. Xamarin supports unit testing through the use of testing frameworks such as NUnit and xUnit. You can also use mocking libraries like Moq or NSubstitute to create mock implementations of your interfaces, allowing you to test your code in isolation.
Real-world Examples of Clean Architecture in Xamarin
Numerous Xamarin projects have successfully implemented clean architecture principles. Some examples include:
- eShopOnContainers: A sample application developed by Microsoft showcasing a microservices architecture using Xamarin.Forms, ASP.NET Core, and Docker.
- Xamflix: A sample Xamarin.Forms application that implements clean architecture, MVVM, and the repository pattern for accessing a movie database API.
- Xamarin.Forms-CleanArchitecture: A clean architecture template for Xamarin.Forms applications, including examples of domain-driven design, CQRS, and dependency injection.
Conclusion
Implementing clean architecture in Xamarin applications helps create scalable, maintainable, and testable solutions. By separating concerns and establishing clear boundaries between layers, you can ensure that your code remains modular and easier to manage. Using dependency injection, data binding, and unit testing further enhances the quality and stability of your application.
As Xamarin continues to grow in popularity, adopting clean architecture principles will become increasingly important for building high-quality cross-platform applications.
FAQs
- What is clean architecture?
Clean architecture is an approach to software development that emphasizes the separation of concerns, maintainability, and testability. It divides an application into various layers, each with its own responsibilities and dependencies.
- Why is clean architecture important in Xamarin applications?
Clean architecture helps create scalable, maintainable, and testable Xamarin applications. It allows for easier collaboration between developers, improves the code’s manageability, and enhances testability.
- What are the key layers in a clean architecture solution for Xamarin?
A clean architecture solution in Xamarin typically consists of four layers: domain, application, infrastructure, and presentation.
- How does dependency injection help in implementing clean architecture in Xamarin?
Dependency injection helps manage dependencies between objects in a clean and decoupled manner. It enables you to easily swap implementations and make your code more testable, promoting a cleaner architecture.
- Can I use clean architecture principles in Xamarin.Forms applications?
Yes, clean architecture principles can be applied to Xamarin.Forms applications, enabling you to build maintainable, scalable, and testable cross-platform apps.