Implementing Clean Architecture in .NET Core
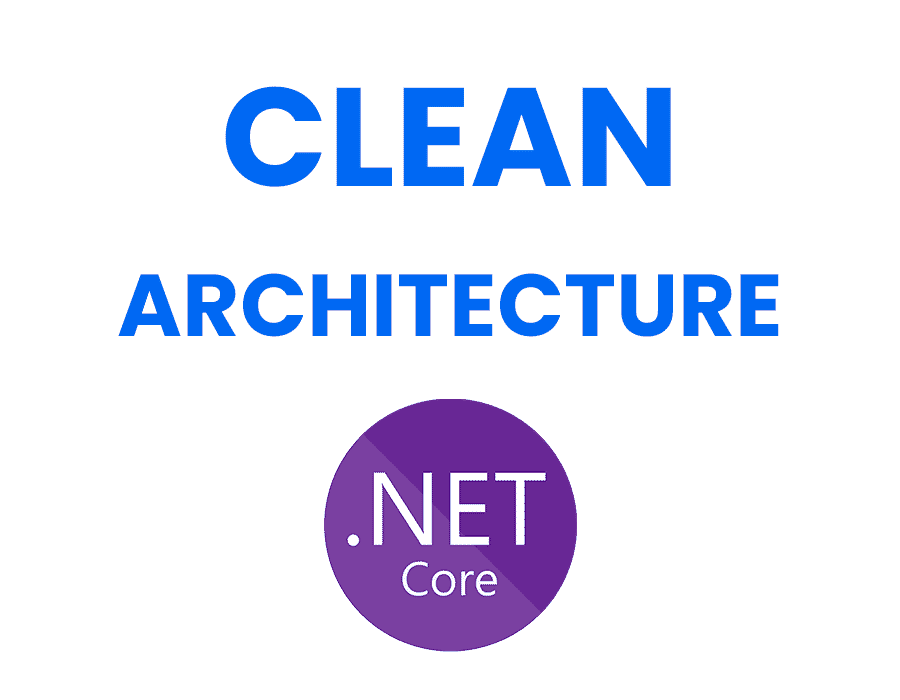
Clean Architecture has become a popular approach for designing and building software applications with a focus on maintainability, testability, and separation of concerns. In this article, we will discuss the concepts behind Clean Architecture and how to implement it in a .NET Core project, providing a solid foundation for future development.
Understanding Clean Architecture
Clean Architecture, also known as Hexagonal or Onion Architecture, was proposed by Robert C. Martin as a way to design software applications with a focus on long-term maintainability and scalability. The main goal of Clean Architecture is to create a decoupled and modular architecture that allows developers to build, test, and maintain their applications independently of external dependencies, such as databases, user interfaces, or external services.
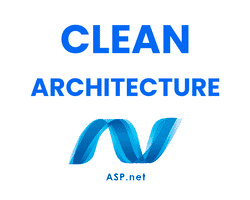
Clean Architecture in ASP.NET
January 15, 2023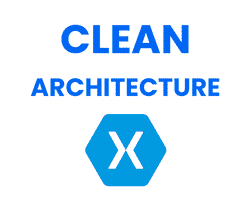
Clean Architecture in Xamarin
January 14, 2023Clean Architecture is a software design pattern that emphasizes the separation of concerns and the use of abstractions to promote a flexible, maintainable, and scalable codebase. By following the principles of Clean Architecture, developers can create software that is easy to modify, test, and extend over time.
Principles of Clean Architecture
There are several key principles at the foundation of Clean Architecture:
- Separation of concerns: The application is divided into multiple layers, each responsible for a specific aspect of the software. This promotes modular design and helps to avoid problems associated with tight coupling.
- : High-level modules should not depend on low-level modules; instead, they should depend on abstractions (such as interfaces) that can be easily replaced or expanded when needed.
- Testability: By designing the application in a decoupled manner, it becomes easier to write unit and integration tests, ensuring the application stays maintainable and bug-free.
- Agile development: Clean Architecture promotes the agile development methodology, emphasizing iterative and incremental improvements, as well as adapting to change.
In addition to these principles, Clean Architecture also encourages the use of SOLID principles, which further contribute to a maintainable and extensible codebase.
Benefits of Clean Architecture
Implementing Clean Architecture in your .NET Core project can provide several benefits:
- Maintainability: A clean, modular codebase is easier to maintain and expand, ensuring long-term development success.
- Scalability: Decoupling application components promotes horizontal and vertical scaling, allowing your application to grow and evolve as your organization does.
- Testability: Writing tests becomes more straightforward, ensuring that your application remains reliable and secure.
- Ease of collaboration: A well-organized project makes it easier for multiple developers to work on the same codebase concurrently.
Now that we have a solid understanding of Clean Architecture and its benefits, let’s dive into setting up a .NET Core project and organizing the project structure to adhere to Clean Architecture principles.
Setting Up a .NET Core Project
Implementing Clean Architecture in a .NET Core project can seem daunting at first, but with the appropriate tools and dependencies installed, it becomes a seamless process. In this section, we’ll guide you through the steps required to set up a .NET Core project for Clean Architecture.
Installing Required Tools and Dependencies
Before you begin, ensure that you have the following tools and dependencies installed on your computer:
- .NET Core SDK: Download and install the latest .NET Core SDK from the official website. This will give you access to all the necessary libraries and tools required for developing .NET Core applications.
- An Integrated Development Environment (IDE): Use a popular IDE like Visual Studio or Visual Studio Code for seamless development and debugging. These IDEs come with built-in support for .NET Core projects, making it easier to manage your codebase.
- Git: Having a version control system is essential for managing your project’s history and collaborating with teammates. Install Git from the official website to ensure that you have access to all the necessary features for version control.
With these tools and dependencies installed, you’re ready to create a new .NET Core solution for your Clean Architecture project.
Creating a New .NET Core Solution
To create a new .NET Core solution, follow these steps:
- Open your preferred IDE and create a new solution using the .NET Core template. This will give you access to all the necessary project templates and configurations required for building a .NET Core application.
- Name your solution according to your project requirements and select a suitable location to save it. This will help you keep track of your project files and ensure that they are organized in a logical manner.
- Add the necessary projects to your solution, such as Domain, Application, Infrastructure, and Presentation projects. This will help you organize your code according to Clean Architecture principles, making it easier to maintain and scale your application in the future.
- Save the solution, and it’s time to start organizing the project structure. This involves creating folders and files for each of the projects you added to your solution, as well as defining the dependencies between them.
With a new .NET Core solution in place, let’s dive into organizing the project structure according to Clean Architecture concepts.
Tip: If you’re new to .NET Core development, consider taking a course or reading a book on the subject. This will help you understand the fundamentals of .NET Core and make it easier to implement Clean Architecture concepts in your project.
Organizing the Project Structure
In a Clean Architecture-based project, the codebase is typically organized into four main layers: Domain, Application, Infrastructure, and Presentation. Each layer has a specific purpose and contains different types of code.
Domain Layer
The Domain layer is the core of the application. It contains the business logic and rules that define the behavior of the system. This layer should be independent of any external dependencies and frameworks. It should only contain pure business logic and data structures.
The Domain layer should define the entities, value objects, and domain services that represent the core concepts of the application. It should also define the interfaces for any external dependencies that the application needs to interact with, such as databases or external APIs.
Application Layer
The Application layer is responsible for coordinating the interactions between the Domain layer and the Infrastructure layer. It contains the use cases and application services that define the high-level behavior of the system.
The Application layer should not contain any business logic. Instead, it should delegate to the Domain layer to perform the necessary operations. The Application layer should also define the interfaces for any external dependencies that the system needs to interact with.
Infrastructure Layer
The Infrastructure layer is responsible for providing the implementation details for the interfaces defined in the Domain and Application layers. It contains the code that interacts with external dependencies such as databases, file systems, and external APIs.
The Infrastructure layer should be designed to be easily replaceable. This means that the code should be decoupled from any specific implementation details, such as the choice of database or web framework.
Presentation Layer
The Presentation layer is responsible for handling the user interface and user input. It contains the code that handles HTTP requests, renders HTML templates, and interacts with JavaScript on the client side.
The Presentation layer should be designed to be easily replaceable. This means that the code should be decoupled from any specific web framework or front-end library.
By organizing the codebase into these four layers, we can achieve a high degree of modularity and maintainability. Each layer has a specific purpose and responsibility, and changes in one layer should not affect the others.