Clean Architecture in ASP.NET Core
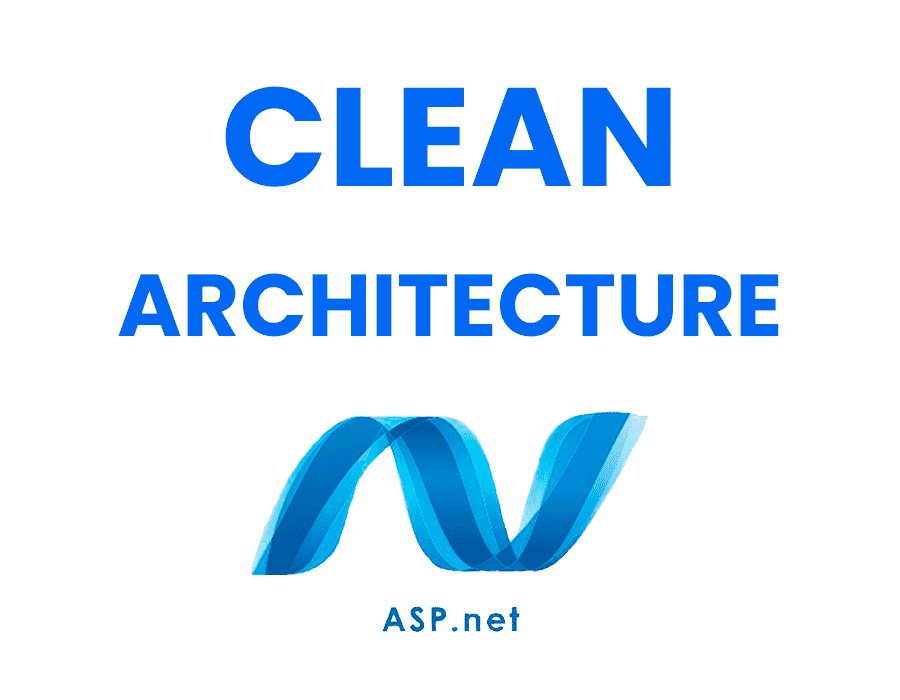
Introduction to Clean Architecture
Clean Architecture is a software design paradigm that focuses on the separation of concerns and the maintainability of code. It has become increasingly popular in recent years due to its ability to improve the quality of software applications by enforcing modularity, testability, and scalability. In this article, we’ll dive into the world of Clean Architecture and learn how it can be applied to ASP.NET Core applications.
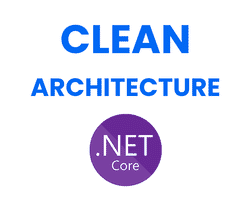
Clean Architecture in .NET Core
February 5, 2023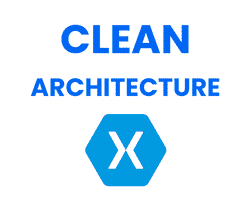
Clean Architecture in Xamarin
January 14, 2023Why Clean Architecture in ASP.NET Core?
ASP.NET Core is a versatile and high-performance framework for building modern web applications. With its flexibility, developers can choose different architectural patterns to suit their specific needs. Clean Architecture is one such pattern that perfectly complements the ASP.NET Core framework.
Benefits of Clean Architecture
- Modularity: Clean Architecture encourages the separation of code into independent modules, making it easier to manage, modify, and extend.
- Testability: By isolating the different parts of the application, testing becomes much more manageable and efficient.
- Scalability: The decoupled nature of Clean Architecture allows for scaling individual components as needed without affecting the entire application.
- Maintainability: A well-structured and modular codebase is easier to understand, making it simpler to maintain and troubleshoot.
ASP.NET Core features supporting Clean Architecture
- Dependency Injection: ASP.NET Core has built-in support for dependency injection, which enables loose coupling between components and simplifies testing.
- Middleware: Middleware allows you to add and remove components in the request-response pipeline, providing flexibility in composing the application’s behavior.
- Configuration: The configuration system in ASP.NET Core is highly extensible, allowing you to manage different environments and settings with ease.
Components of Clean Architecture
Clean Architecture is typically organized into four layers:
Domain Layer
The Domain Layer contains the core business logic and entities of the application. These entities represent the main concepts of the application and are independent of any specific technology or infrastructure.
Application Layer
The Application Layer is responsible for orchestrating the business logic and serves as a bridge between the Domain Layer and the other layers. It contains application-specific logic, such as validation and authorization, and communicates with the Domain Layer through interfaces.
Infrastructure Layer
The Infrastructure Layer contains the implementations of the interfaces defined in the Domain Layer. It is responsible for managing external dependencies, such as databases, APIs, and file systems.
Presentation Layer
The Presentation Layer is responsible for displaying data to the user and handling user input. In ASP.NET Core applications, the Presentation Layer typically consists of controllers, views, and view models.
Implementing Clean Architecture in ASP.NET Core
Now that we understand the core concepts of Clean Architecture, let’s go through the process of implementing it in an ASP.NET Core application.
Setting up the project structure
First, create a new ASP.NET Core project and organize it into the following folders:
- Domain: Contains the domain entities and interfaces.
- Application: Houses the application services, DTOs, and interfaces.
- Infrastructure: Contains the implementations of the interfaces defined in the Domain layer.
- Presentation: Consists of the controllers, views, and view models.
Configuring Dependency Injection
ASP.NET Core’s built-in dependency injection system is a powerful tool for managing dependencies between layers. Register your services and repositories in the Startup.cs
file by using the AddScoped
, AddTransient
, or AddSingleton
methods, depending on their desired lifetime.
Implementing Domain Entities and Interfaces
In the Domain folder, create the core business entities and define the interfaces for the services and repositories. These interfaces will later be implemented in the Infrastructure layer.
Creating Application Services and DTOs
In the Application folder, implement the application services that interact with the Domain layer through the interfaces. Use Data Transfer Objects (DTOs) to communicate between the Presentation and Application layers, ensuring a clean separation of concerns.
Building the Infrastructure and Presentation Layers
Finally, implement the Domain layer interfaces in the Infrastructure folder, and create the controllers and views in the Presentation folder.
Best Practices for Clean Architecture in ASP.NET Core
To ensure a robust implementation of Clean Architecture in your ASP.NET Core application, follow these best practices:
- Keep the Domain layer free of any technology-specific dependencies.
- Use the Dependency Inversion Principle to ensure loose coupling between layers.
- Avoid circular dependencies between layers by implementing the interfaces in the correct layer.
- Separate the concerns of your application by maintaining clear boundaries between layers.
- Write unit tests for each layer, focusing on testing the business logic in the Domain layer and the application logic in the Application layer.
Conclusion
Clean Architecture is an effective way to build maintainable, testable, and scalable applications in ASP.NET Core. By following the principles and best practices outlined in this article, you can create a robust application that is easier to manage and extend. Implementing Clean Architecture in your projects will result in higher quality software and a more enjoyable development experience.
FAQs
- What is Clean Architecture?
- Why should I use Clean Architecture in ASP.NET Core?
- What are the main components of Clean Architecture?
- How can I implement Clean Architecture in an ASP.NET Core project?
- What are some best practices for implementing Clean Architecture in ASP.NET Core?